How to Implement Design Patterns in C# with GPT
Updated on December 24, 2024

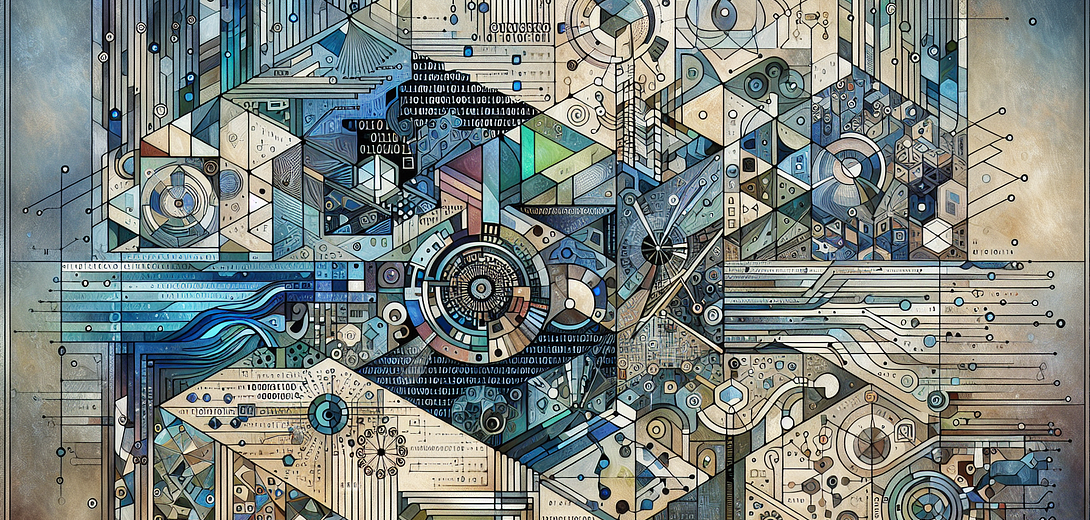
Design patterns are essential in software development, offering solutions to common issues and enhancing code maintainability. By integrating design patterns with AI-powered tools like Cloving CLI, you can significantly boost your coding workflow. This blog post will guide you through using Cloving to efficiently implement design patterns in C#.
What is Cloving CLI?
Cloving CLI is an AI-enhanced command-line interface designed to augment your programming workflow. It provides a suite of features that improve productivity and code quality by leveraging AI to generate, review, and refactor code seamlessly.
With Cloving CLI, you can:
- Generate code snippets based on design patterns
- Review existing code for pattern adherence
- Refine pattern implementations interactively
Let’s delve into the practical steps to implement design patterns using Cloving CLI.
1. Setting Up Cloving
To start using Cloving, you need to configure it within your development environment.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving to align with your environment:
cloving config
You’ll be prompted to supply your API key and select a preferred model.
2. Project Initialization
Initialize Cloving in your C# project directory to enable context understanding:
cloving init
3. Implementing Design Patterns
Cloving’s powerful code generation can help implement various design patterns. Consider the Singleton Pattern as an example.
Implementing the Singleton Pattern:
Open your command line in the project directory and run the following command:
cloving generate code --prompt "Implement a Singleton Pattern in C#" --files src/Singleton.cs --save
This command generates the C# code for the Singleton pattern. An example might look like this:
// src/Singleton.cs
public sealed class Singleton
{
private static Singleton instance = null;
private static readonly object padlock = new object();
Singleton()
{
}
public static Singleton Instance
{
get
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
}
4. Reviewing and Refining Patterns
After generating the code, engage Cloving to review or refine the implementation. Start an interactive session to enhance or refactor:
cloving chat -f src/Singleton.cs
Within the chat session, you can make requests such as:
- Refine for thread safety
- Explain the design pattern
- Add comments for clarity
Example output from Cloving might refactor the Singleton pattern like this:
// src/Singleton.cs
public sealed class Singleton
{
private static Singleton instance = null;
private static readonly object padlock = new object();
// Constructor is private to prevent instantiation.
private Singleton() {}
// Public method to provide access to the instance.
public static Singleton Instance
{
get
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
}
return instance;
}
}
}
5. Implementing Other Patterns
Cloving can assist in implementing various design patterns. Here are some examples:
Factory Pattern:
cloving generate code --prompt "Implement a Factory Pattern in C#" --files src/Factory.cs --save
Output:
// src/Factory.cs
public interface IProduct
{
string ShipFrom();
}
public class ProductA : IProduct
{
public string ShipFrom()
{
return "Shipping from Product A";
}
}
public class ProductB : IProduct
{
public string ShipFrom()
{
return "Shipping from Product B";
}
}
public class ProductFactory
{
public static IProduct CreateProduct(string type)
{
switch (type)
{
case "A":
return new ProductA();
case "B":
return new ProductB();
default:
throw new ArgumentException("Invalid product type");
}
}
}
6. Testing with Generated Unit Tests
To ensure the correctness of your design pattern implementations, generate unit tests:
cloving generate unit-tests -f src/Singleton.cs
Cloving will create unit tests that verify your Singleton implementation behaves correctly.
7. Committing Your Changes
Once satisfied with the implementation and review, use Cloving to create insightful commit messages:
cloving commit
The command generates a well-contextualized commit message to summarize your changes.
Conclusion
Utilizing Cloving CLI for implementing design patterns in C# makes your development process more efficient and robust. The AI-powered tool not only facilitates seamless code generation but also enhances code quality through reviews and refinements.
By leveraging Cloving CLI, developers can focus more on architectural decisions, while AI handles code snippets and pattern applications. Start integrating Cloving into your C# projects today and enjoy the benefits of AI-driven development. Whether it’s the Singleton, Factory, or any other pattern, Cloving is your AI ally in software craftsmanship.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.