How to Generate Serverless Functions Using GPT
Updated on June 26, 2024

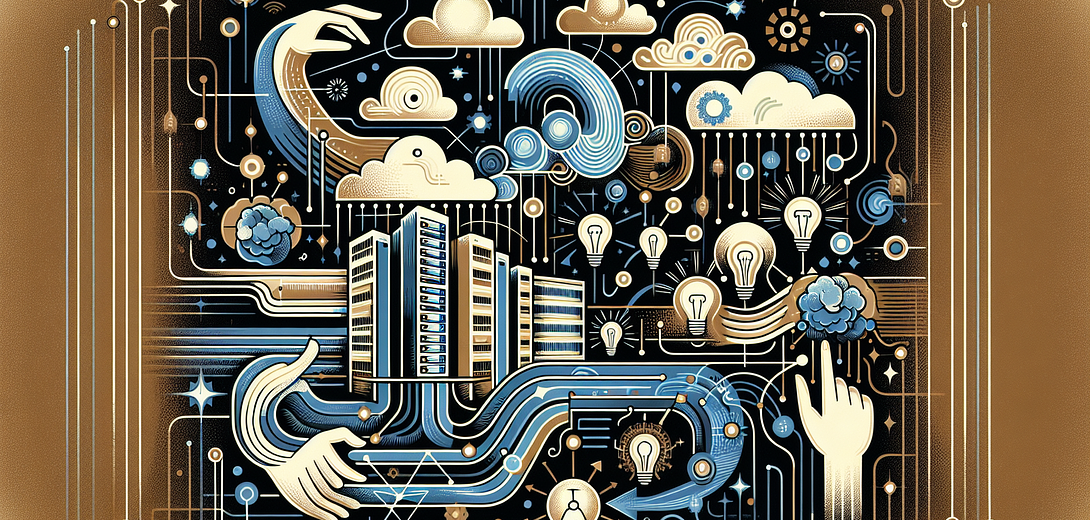
Serverless computing has revolutionized the way we build and deploy applications. By allowing developers to create on-demand, scalable functions without worrying about server management, it offers unparalleled flexibility and efficiency.
But what if you could further enhance this workflow by integrating the analytical prowess of AI with your human creativity and intuition? Enter cloving: a synergistic approach where human expertise and artificial intelligence work together to achieve a common goal.
In this post, we’ll explore how to generate serverless functions using GPT to make your programming tasks more efficient and effective.
Understanding Cloving
Cloving isn’t just about using AI; it’s about creating a harmonious relationship where both human and machine strengths are fully leveraged. By combining your domain knowledge and creativity with GPT’s analytical capabilities, you can streamline the process of generating serverless functions.
1. Generating Basic Function Templates
Writing the boilerplate code for serverless functions can be tedious. GPT can generate solid starting templates, ensuring you cover all the necessary components.
Example: Generating AWS Lambda Function Template
If you’re building an AWS Lambda function to process incoming data, you can prompt GPT:
Write an AWS Lambda function in Python that processes incoming JSON data and logs the content.
GPT will generate:
import json
def lambda_handler(event, context):
# Parse the incoming JSON data
data = json.loads(event['body'])
# Log the content
print("Received data:", data)
return {
'statusCode': 200,
'body': json.dumps('Data processed successfully!')
}
2. Enhancing Functions with Business Logic
Once you have the basic template, you can ask GPT to add specific business logic to the function.
Example: Adding Custom Data Processing
If you need to add custom processing steps to the function, you can ask:
Modify the Lambda function to filter out users based on their age and return only users older than 18.
GPT will enhance the function:
import json
def lambda_handler(event, context):
# Parse the incoming JSON data
data = json.loads(event['body'])
# Filter users based on age
adults = [user for user in data if user['age'] > 18]
# Log the content
print("Filtered adult users:", adults)
return {
'statusCode': 200,
'body': json.dumps(adults)
}
3. Generating Deployment Scripts
Deploying serverless functions can involve multiple steps. GPT can help you create deployment scripts to streamline the process.
Example: Generating AWS CLI Deployment Script
To deploy the Lambda function using AWS CLI, you can prompt GPT:
Generate an AWS CLI script to deploy the Lambda function written in Python.
GPT will produce:
# Package the Lambda function
zip function.zip lambda_function.py
# Create a new Lambda function
aws lambda create-function \
--function-name ProcessUserData \
--zip-file fileb://function.zip \
--handler lambda_function.lambda_handler \
--runtime python3.8 \
--role arn:aws:iam::account-id:role/lambda-execution-role
4. Generating Test Cases
Thorough testing is crucial for ensuring function reliability. GPT can help you generate test cases for your serverless functions.
Example: Generating Test Events for AWS Lambda
To create test events for your Lambda function, you can ask GPT:
Generate a test event for the Lambda function that processes JSON data of users.
GPT will generate an appropriate test event:
{
"body": "[{\"name\": \"John Doe\", \"age\": 20}, {\"name\": \"Jane Doe\", \"age\": 17}]"
}
You can use this JSON as a test event in the AWS Lambda console or through AWS CLI to ensure your function behaves as expected.
5. Documentation and Explanation
Clear documentation is critical for maintaining and scaling codebases. GPT can assist you in generating comprehensive documentation for your serverless functions.
Example: Documenting the Lambda Function
When you need to document your Lambda function, you can ask GPT:
Generate documentation for the Lambda function that processes user data.
GPT will generate:
# ProcessUserData Lambda Function
## Description
This AWS Lambda function processes incoming JSON data of users and filters out users based on their age.
## Parameters
- `event`: The event object containing the request data.
- `context`: AWS Lambda context object.
## Input
The function expects a JSON object in the `body` field with an array of users. Each user should have the following format:
```json
{
"name": "string",
"age": "integer"
}
Output
The function returns a JSON array of users who are older than 18.
Example
Input
{
"body": "[{\"name\": \"John Doe\", \"age\": 20}, {\"name\": \"Jane Doe\", \"age\": 17}]"
}
Output
[
{
"name": "John Doe",
"age": 20
}
]
Conclusion
Integrating GPT into your workflow for generating serverless functions is a prime example of cloving—harnessing the combined power of human creativity and AI’s analytical capabilities. By following these steps, you can make your serverless development process more efficient, effective, and enjoyable. Embrace cloving and experience the transformative impact of this collaborative approach on your programming tasks.
Bonus Prompts
To further enhance your programming workflows, here are a few bonus prompts that can be incredibly useful. These prompts will help you generate more sophisticated scripts and adapt to different languages seamlessly.
Creating a Terraform Deployment Script
Terraform is a powerful tool for infrastructure as code, allowing you to manage and provision your cloud resources efficiently. GPT can assist in generating a basic Terraform deployment script.
Example: Generating a Terraform Script for AWS Lambda Deployment
You can prompt GPT:
Generate a Terraform script to deploy an AWS Lambda function using Python.
GPT will generate:
provider "aws" {
region = "us-west-2"
}
resource "aws_lambda_function" "process_user_data" {
filename = "function.zip"
function_name = "ProcessUserData"
role = aws_iam_role.lambda_exec.arn
handler = "lambda_function.lambda_handler"
runtime = "python3.8"
source_code_hash = filebase64sha256("function.zip")
}
resource "aws_iam_role" "lambda_exec" {
name = "lambda_exec_role"
assume_role_policy = jsonencode({
Version = "2012-10-17",
Statement = [
{
Action = "sts:AssumeRole",
Effect = "Allow",
Principal = {
Service = "lambda.amazonaws.com"
}
}
]
})
}
resource "aws_iam_role_policy_attachment" "lambda_exec_policy" {
role = aws_iam_role.lambda_exec.name
policy_arn = "arn:aws:iam::aws:policy/service-role/AWSLambdaBasicExecutionRole"
}
Changing the Language to Node.js
Adapting to different languages is a common need in diverse development environments. GPT can assist in converting your serverless functions to Node.js.
Example: Converting Python Lambda Function to Node.js
You can prompt GPT:
Convert the AWS Lambda function that processes JSON data of users from Python to Node.js.
GPT will generate:
const AWS = require('aws-sdk');
exports.handler = async (event) => {
// Parse the incoming JSON data
const data = JSON.parse(event.body);
// Filter users based on age
const adults = data.filter(user => user.age > 18);
// Log the content
console.log("Filtered adult users:", adults);
const response = {
statusCode: 200,
body: JSON.stringify(adults),
};
return response;
};
These bonus prompts further illustrate the power of integrating GPT into your workflow, making your programming tasks even more versatile and efficient. By leveraging GPT for creating deployment scripts and adapting code to different languages, you can continue to streamline your development process and maintain a high level of productivity.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.