How to Generate Clean and Efficient C# Code with GPT
Updated on January 07, 2025

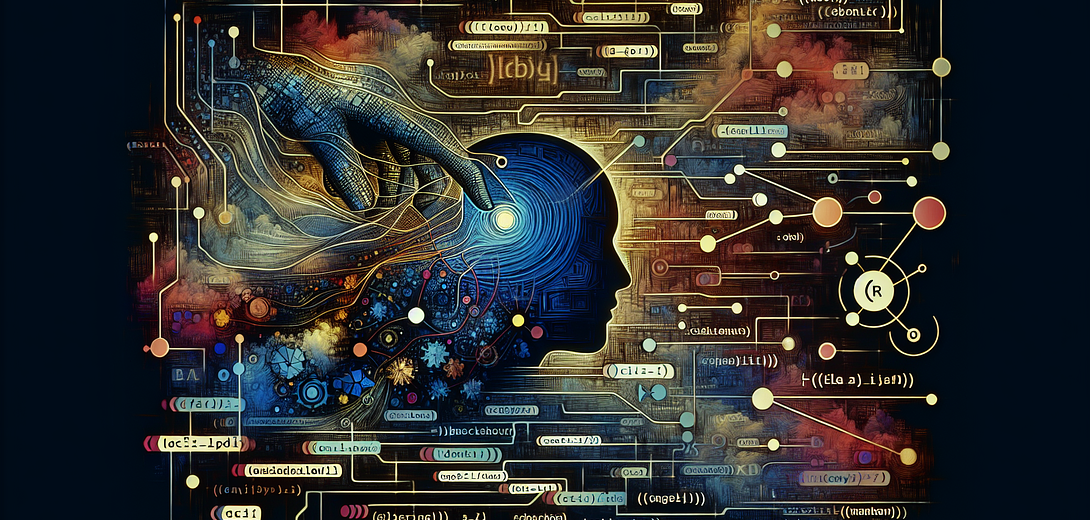
In the ever-evolving landscape of software development, writing clean and efficient code is paramount. The Cloving CLI tool offers a powerful way to integrate AI into your coding workflow, enabling you to generate high-quality C# code swiftly and effectively. Whether you are building a complex application or automating mundane tasks, Cloving can enhance your development experience. In this article, we’ll walk through the steps to generate clean and efficient C# code with Cloving CLI, including practical examples and best practices.
Getting Started with Cloving CLI
Before diving into code generation, it is essential to have Cloving installed and configured in your development environment.
Installation
To install Cloving globally, use npm:
npm install -g cloving@latest
Configuration
Once installed, you need to configure Cloving with your AI settings:
cloving config
Follow the interactive prompts to select the preferred AI model, API key, and other settings tailored to your needs.
Initializing Your Project
Navigate to your C# project directory and initialize Cloving:
cloving init
This command analyzes your project and sets up a cloving.json
file to store metadata and contextual information.
Generating C# Code
Cloving’s generate
command can be used to produce C# snippets that fit within the context of your project.
Example: Creating a C# Class
Suppose you’re developing a library management system and you need a Book
class. You can ask Cloving to generate it for you:
cloving generate code --prompt "Create a C# class for a Book with title, author, and ISBN properties" --files src/LibrarySystem/Book.cs
Here’s a possible output for the C# class:
namespace LibrarySystem
{
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public string ISBN { get; set; }
public Book(string title, string author, string isbn)
{
Title = title;
Author = author;
ISBN = isbn;
}
public void DisplayInfo()
{
Console.WriteLine($"Title: {Title}, Author: {Author}, ISBN: {ISBN}");
}
}
}
Reviewing and Refining Generated Code
Cloving allows you to review, refine, and save your generated code. You can request explanations or make specific revisions:
Revise the Book class to include a method that checks if two books have the same ISBN
This helps to ensure your code is clean, efficient, and aligns with your project needs.
Generating Unit Tests
Quality assurance is crucial, and Cloving can aid you in generating unit tests for your classes.
Example: Generating Unit Tests for the Book Class
To create unit tests for the Book
class, use the following command:
cloving generate unit-tests -f src/LibrarySystem/Book.cs
Expected output might be a set of tests to validate the functionalities of your Book
class:
using System;
using Xunit;
using LibrarySystem;
public class BookTests
{
[Fact]
public void BookInitializationTest()
{
var book = new Book("The Great Gatsby", "F. Scott Fitzgerald", "1234567890");
Assert.Equal("The Great Gatsby", book.Title);
Assert.Equal("F. Scott Fitzgerald", book.Author);
Assert.Equal("1234567890", book.ISBN);
}
[Fact]
public void DisplayInfoMethodTest()
{
var book = new Book("The Great Gatsby", "F. Scott Fitzgerald", "1234567890");
book.DisplayInfo(); // This should print: "Title: The Great Gatsby, Author: F. Scott Fitzgerald, ISBN: 1234567890"
}
}
Interactive Chat with Cloving
For more complex tasks or when you need interactive assistance, Cloving’s chat feature is invaluable:
cloving chat -f src/LibrarySystem/Book.cs
Engage in an interactive session, where you can pose questions, request code snippets, or gain explanations about your codebase.
Best Practices for Generating C# Code with Cloving
-
Provide Specific and Descriptive Prompts: When using the generate command, ensure your prompts are detailed and specific to get the best results.
-
Leverage Project Context: Utilize the
--files
option to feed relevant files as context to Cloving, aiding in generating code tailored to your project. -
Review and Iterate: Regularly review and refine generated code to align it with your coding standards and project requirements.
-
Combine with Unit Testing: Use Cloving to generate unit tests along with business logic, maintaining high code quality.
-
Utilize Chat for Complex Queries: Engage with the Cloving chat mode to handle complex tasks that require more than a one-off code snippet.
Conclusion
Integrating Cloving CLI into your C# development workflow can greatly enhance your productivity and code quality. By leveraging AI, you can generate clean and efficient C# code, streamline testing, and automate repetitive tasks. Embrace Cloving as a powerful support tool to augment your programming skills and boost your productivity in the ever-demanding software development landscape.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.