How to Build Lightning-Fast Node.js Services with GPT's Help
Updated on November 27, 2024

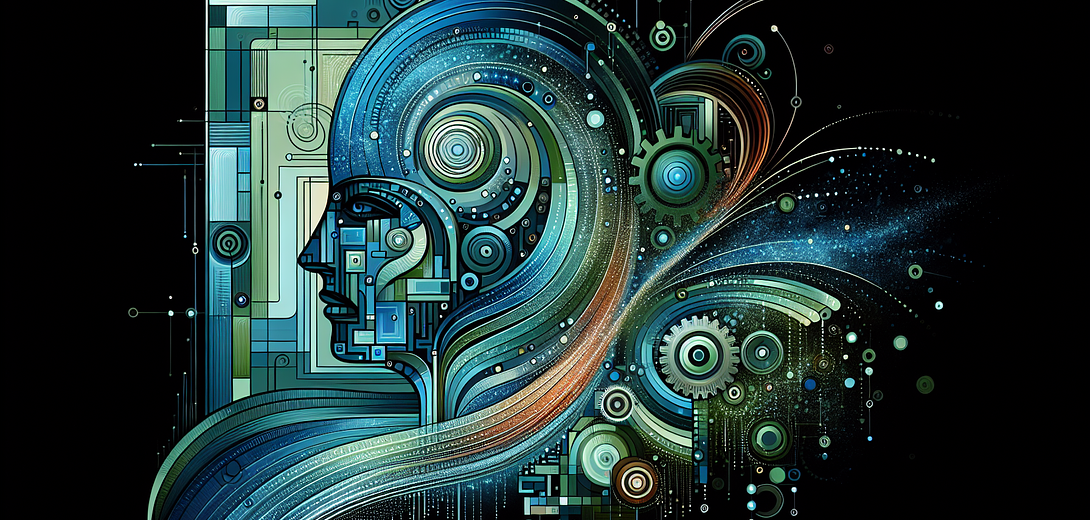
Building efficient, high-performance Node.js services can be challenging, especially when balancing development speed with reliability and scalability. Fortunately, the Cloving CLI tool can be your AI-enhanced co-pilot, assisting you in crafting lightning-fast Node.js services with ease. In this blog post, we’ll explore how to integrate GPT’s capabilities into your Node.js workflows, using Cloving, to elevate code quality and optimize productivity.
Getting Started with Cloving CLI
To begin harnessing the power of Cloving CLI in your Node.js development environment, follow the steps below to install and configure the tool:
Step 1: Install Cloving
First, you need to install Cloving globally on your system. You can achieve this by running the following command:
npm install -g cloving@latest
Step 2: Configure Cloving
To integrate Cloving with GPT models and set your preferences, you’ll need to configure it with your API key and model selection:
cloving config
Follow the interactive prompts to set up Cloving with your preferred model and enter your API key.
Step 3: Initialize Your Project
Initialize Cloving in your Node.js project directory to provide it with your project context:
cloving init
This command will generate a cloving.json
file that stores metadata and context about your project, facilitating AI-assisted code generation.
Generating Efficient Code with Cloving
Once Cloving is set up, you can start leveraging its code generation capabilities to create efficient Node.js services rapidly.
Example: Creating a RESTful Service
Suppose you want to create a RESTful service to manage user data. You can ask Cloving to generate a basic Node.js service with Express:
cloving generate code --prompt "Create a basic Express.js RESTful service for managing users with endpoints for CRUD operations" --files src/server.js
Cloving will use the context to generate a service file like below:
// src/server.js
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
let users = [];
// Create a new user
app.post('/users', (req, res) => {
const user = req.body;
users.push(user);
res.status(201).send(user);
});
// Read all users
app.get('/users', (req, res) => {
res.send(users);
});
// Update a user
app.put('/users/:id', (req, res) => {
const { id } = req.params;
const userUpdate = req.body;
users = users.map(user => user.id === Number(id) ? { ...user, ...userUpdate } : user);
res.send(users.find(user => user.id === Number(id)));
});
// Delete a user
app.delete('/users/:id', (req, res) => {
const { id } = req.params;
users = users.filter(user => user.id !== Number(id));
res.status(204).send();
});
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Utilizing Cloving Chat for Reviewing and Iterating on Generated Code
For situations where you need ongoing guidance or explanations, leverage Cloving’s chat mode:
cloving chat -f src/server.js
This will open an interactive chat session where you can ask Cloving to explain code snippets, suggest optimizations, or assist with more complex service implementations.
For example, after generating your basic service, you can review and iterate on the code using the interactive capabilities of Cloving Chat:
What would you like to do?
cloving> Revise the server to include validation for user input
Cloving will assist you in refining the service by adding validation logic, ensuring robustness and reliability.
Generating Documentation and Tests
Cloving can also generate documentation and unit tests, streamlining your documentation processes and increasing test coverage:
cloving generate unit-tests --files src/server.js
The above command will create unit tests for your service, enhancing its reliability:
// src/server.test.js
const request = require('supertest');
const app = require('./server');
describe('User API', () => {
it('should create a user', async () => {
const response = await request(app).post('/users').send({ id: 1, name: 'John Doe' });
expect(response.statusCode).toBe(201);
expect(response.body.name).toBe('John Doe');
});
// additional tests...
});
Making Commits with AI-generated Messages
To maintain clear and concise code repositories, use Cloving to generate informative commit messages:
cloving commit
This will analyze your changes and suggest a commit message that captures the essence of your modifications.
Conclusion
Leveraging Cloving CLI throughout your Node.js service development can lead to faster, more efficient coding sessions and higher-quality services. Through AI-powered code generation, interactive chat assistance, and automated testing, Cloving empowers you to build Node.js services that are not only fast but also reliable and maintainable.
Begin integrating Cloving into your development workflows and witness the transformative impact of harnessing AI to boost your programming capabilities.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.