How to Build Laravel Controllers with GPT
Updated on July 01, 2024

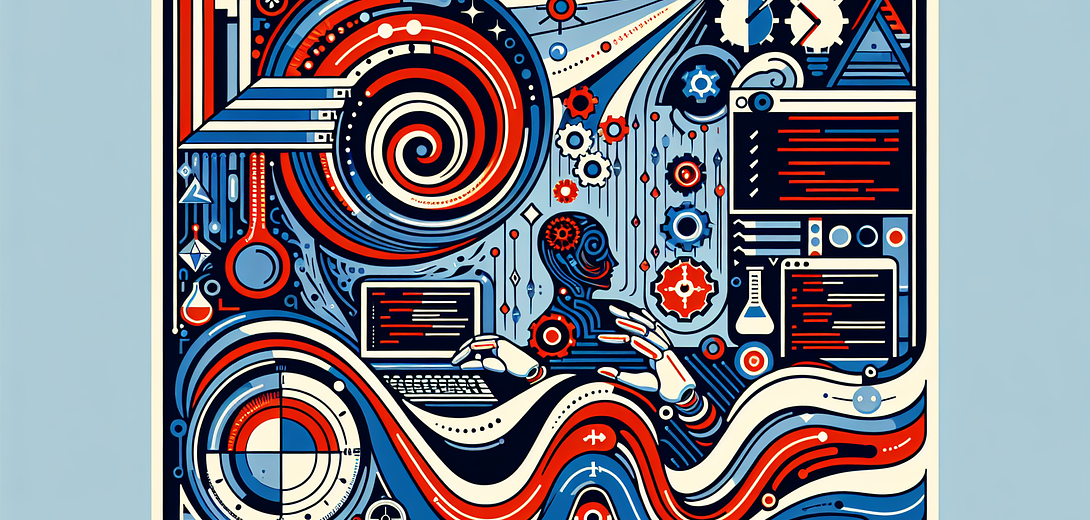
In today’s rapidly evolving technological landscape, integrating artificial intelligence (AI) into our workflows can dramatically enhance efficiency and productivity. For computer programmers, understanding and utilizing AI tools like GPT can make a substantial difference in daily tasks. One area where this integration, or “cloving”—the combination of human creativity and AI processing power—can be particularly impactful is in building Laravel controllers.
In this blog post, we’ll walk through practical examples, tips, and best practices for incorporating GPT into your Laravel development workflow to streamline the process of creating controllers.
Understanding Cloving
Cloving represents the synergistic blend of human intuition and creativity with AI’s analytical capabilities. It’s not just about employing AI for the sake of it; it’s about fostering a collaborative effort where both human and machine attributes are leveraged, leading to more efficient and effective outcomes.
1. Generating Basic Controller Code
Often, the initial step in creating a new Laravel controller is setting up the basic structure. GPT can help you get started quickly by providing a boilerplate controller code tailored to your requirements.
Example:
Suppose you need to create a PostController
for handling blog post-related operations. You can prompt GPT:
Generate a basic Laravel controller named PostController with the methods index, create, store, show, edit, update, and destroy.
GPT will generate the following code:
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function create()
{
return view('posts.create');
}
public function store(Request $request)
{
$validatedData = $request->validate([
// validation rules
]);
Post::create($validatedData);
return redirect()->route('posts.index');
}
public function show(Post $post)
{
return view('posts.show', compact('post'));
}
public function edit(Post $post)
{
return view('posts.edit', compact('post'));
}
public function update(Request $request, Post $post)
{
$validatedData = $request->validate([
// validation rules
]);
$post->update($validatedData);
return redirect()->route('posts.index');
}
public function destroy(Post $post)
{
$post->delete();
return redirect()->route('posts.index');
}
}
2. Validating Requests
Validation is a key aspect of handling user input. GPT can assist in generating robust validation logic within your controller methods.
Example:
If you need to add validation rules for the store
and update
methods in your PostController
, you can prompt GPT:
Add validation rules for a Laravel `PostController` that requires title and body fields in the `store` and `update` methods.
GPT will enhance the controller methods like this:
public function store(Request $request)
{
$validatedData = $request->validate([
'title' => 'required|string|max:255',
'body' => 'required|string',
]);
Post::create($validatedData);
return redirect()->route('posts.index');
}
public function update(Request $request, Post $post)
{
$validatedData = $request->validate([
'title' => 'required|string|max:255',
'body' => 'required|string',
]);
$post->update($validatedData);
return redirect()->route('posts.index');
}
3. Generating Test Cases
Testing your controllers is crucial for maintaining robust applications. GPT can generate PHPUnit tests for your controller methods.
Example:
To create PHPUnit tests for your PostController
, you can prompt GPT:
Generate PHPUnit tests for the `index`, `store`, `show`, `update`, and `destroy` methods of the `PostController`.
GPT will produce the following test cases:
<?php
namespace Tests\Feature;
use App\Models\Post;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class PostControllerTest extends TestCase
{
use RefreshDatabase;
/** @test */
public function it_can_display_a_list_of_posts()
{
$this->actingAs(User::factory()->create());
Post::factory()->count(3)->create();
$response = $this->get(route('posts.index'));
$response->assertStatus(200);
$response->assertViewIs('posts.index');
}
/** @test */
public function it_can_store_a_new_post()
{
$this->actingAs(User::factory()->create());
$postData = [
'title' => 'Sample Post',
'body' => 'This is a sample post body.',
];
$response = $this->post(route('posts.store'), $postData);
$response->assertRedirect(route('posts.index'));
$this->assertDatabaseHas('posts', $postData);
}
/** @test */
public function it_can_display_a_single_post()
{
$post = Post::factory()->create();
$response = $this->get(route('posts.show', $post));
$response->assertStatus(200);
$response->assertViewIs('posts.show');
}
/** @test */
public function it_can_update_a_post()
{
$post = Post::factory()->create();
$postData = [
'title' => 'Updated Post Title',
'body' => 'Updated Post Body.',
];
$response = $this->put(route('posts.update', $post), $postData);
$response->assertRedirect(route('posts.index'));
$this->assertDatabaseHas('posts', $postData);
}
/** @test */
public function it_can_delete_a_post()
{
$post = Post::factory()->create();
$response = $this->delete(route('posts.destroy', $post));
$response->assertRedirect(route('posts.index'));
$this->assertDeleted($post);
}
}
4. Learning and Adapting Best Practices
Staying up-to-date with Laravel’s best practices is integral for maintaining efficient and maintainable code. GPT can provide insights and guidance on the latest trends and best practices.
Example:
To learn about the latest best practices for building Laravel controllers, you can ask GPT:
What are some best practices for building Laravel controllers in 2024?
GPT might suggest the following:
- Use resource controllers to handle common CRUD operations.
- Keep controllers thin by moving business logic to service classes or models.
- Use request validation classes to handle complex validation logic.
- Keep actions in controllers focused and limited to a single responsibility.
- Adopt the repository pattern for data retrieval to keep controllers clean.
- Make use of dependency injection to manage dependencies.
5. Documentation and Explanation
Understanding and documenting complex controllers can be challenging. GPT can help generate clear explanations and documentation.
Example:
To document your PostController
, you can prompt GPT:
Generate documentation for the `PostController`.
GPT will create detailed comments for each method:
/**
* Display a listing of the posts.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
// ...
}
/**
* Show the form for creating a new post.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
// ...
}
/**
* Store a newly created post in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
// ...
}
/**
* Display the specified post.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function show(Post $post)
{
// ...
}
/**
* Show the form for editing the specified post.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function edit(Post $post)
{
// ...
}
/**
* Update the specified post in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Post $post)
{
// ...
}
/**
* Remove the specified post from storage.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function destroy(Post $post)
{
// ...
}
Conclusion
By embracing cloving and leveraging the power of GPT, you can drastically enhance your efficiency in building Laravel controllers. Incorporating GPT into your workflow—from generating initial controller structures to validation, testing, best practices, and documentation—will save you time and help you stay current with industry standards. Embrace this powerful collaboration between human creativity and AI capability to transform your Laravel development experience.
Bonus Follow-Up Prompts
Here are a few extra prompts you could use to refine the process even further:
How can I configure GitHub to run these tests automatically for me?
And here is another:
Generate accompanying factories, for example, data.
And one more:
What are other GPT prompts I could use to make this more efficient?
By integrating these principles and tactics into your daily development workflow, you can become more efficient and effective at your job, harnessing the full potential of cloving with GPT.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.