How to Build Angular Components with GPT
Updated on June 26, 2024

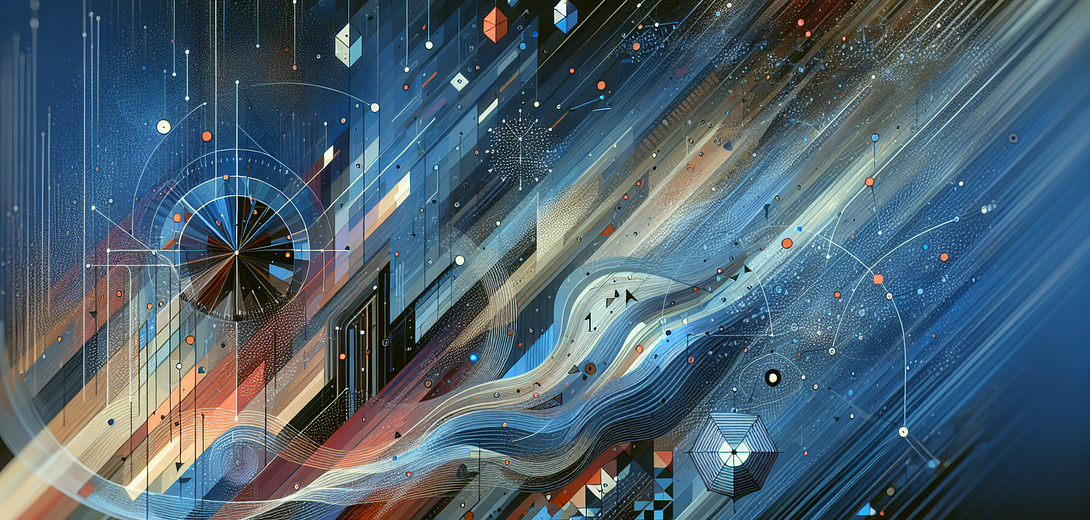
In the bustling world of front-end development, Angular continues to be a widely favored framework for building dynamic web applications. However, creating efficient and maintainable Angular components can often be a complex and time-consuming task.
By embracing the concept of cloving—integrating human creativity and intuition with the advanced processing capabilities of artificial intelligence (AI)—programmers can significantly enhance their efficiency and effectiveness.
In this blog post, we will explore how AI tools like GPT can assist you in building Angular components, making your development process faster and more intuitive.
Understanding Cloving
Cloving merges the unique strengths of human intuition and creativity with the computational power of AI. By fostering a collaborative relationship between human and machine, developers can achieve superior results faster and with fewer errors.
1. Component Creation and Scaffolding
Starting from scratch can be daunting. GPT can provide the foundational boilerplate code and assist in setting up your Angular components.
Example:
Imagine you are tasked with creating a new Angular component for displaying user profiles. You can prompt GPT with:
Generate an Angular component for displaying user profiles, including fields for name, email, and profile picture.
GPT will respond with a scaffolded component, complete with necessary boilerplate code:
// user-profile.component.ts
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-user-profile',
templateUrl: './user-profile.component.html',
styleUrls: ['./user-profile.component.css']
})
export class UserProfileComponent {
@Input() name: string;
@Input() email: string;
@Input() profilePictureUrl: string;
}
<!-- user-profile.component.html -->
<div class="user-profile">
<img [src]="profilePictureUrl" alt="{{ name }}'s profile picture">
<h2>{{ name }}</h2>
<p>{{ email }}</p>
</div>
/* user-profile.component.css */
.user-profile {
text-align: center;
}
.user-profile img {
width: 100px;
height: 100px;
border-radius: 50%;
}
2. Complex Logic Implementation
GPT can provide guidance and implementations for more complex logic that might need to be integrated into your Angular components.
Example:
If you need to implement a function within the component that filters a list of users based on a search query, you can ask GPT:
Add a method to the UserProfileComponent for filtering an array of users based on a search query.
GPT will generate the appropriate code:
// user-profile.component.ts
export class UserProfileComponent {
@Input() name: string;
@Input() email: string;
@Input() profilePictureUrl: string;
@Input() users: Array<{ name: string, email: string, profilePictureUrl: string }>;
filterUsers(searchQuery: string) {
return this.users.filter(user => user.name.toLowerCase().includes(searchQuery.toLowerCase()));
}
}
3. Ensuring Best Practices
Staying up-to-date with Angular best practices can enhance both performance and maintainability of your components. GPT can help provide insights into current best practices.
Example:
To ensure your component adheres to the latest best practices, you can query GPT:
What are the best practices for building efficient and maintainable Angular components in 2024?
GPT might provide a list such as:
- Use Angular’s built-in reactive programming tools like RxJS for efficient state management.
- Lazy-load components to improve performance.
- Keep components small and focused on a single responsibility.
- Utilize Angular’s dependency injection system to manage service lifecycles effectively.
4. Generating Unit Tests
Writing unit tests is crucial, but it can be monotonous. GPT can speed up this process by generating relevant test cases.
Example:
If you need to write unit tests for the filterUsers method, you can ask GPT:
Generate Jasmine unit tests for the filterUsers method in UserProfileComponent.
GPT will produce the necessary test cases:
// user-profile.component.spec.ts
import { UserProfileComponent } from './user-profile.component';
describe('UserProfileComponent', () => {
let component: UserProfileComponent;
beforeEach(() => {
component = new UserProfileComponent();
});
it('should filter users based on search query', () => {
const users = [
{ name: 'Alice', email: '[email protected]', profilePictureUrl: '' },
{ name: 'Bob', email: '[email protected]', profilePictureUrl: '' }
];
component.users = users;
const filteredUsers = component.filterUsers('alice');
expect(filteredUsers.length).toBe(1);
expect(filteredUsers[0].name).toBe('Alice');
});
});
5. Documentation and Explanation
Clear documentation is essential for collaborative development and future maintenance. GPT can help generate comprehensive documentation for your components.
Example:
When you need to document the UserProfileComponent, you can prompt GPT:
Generate documentation for the UserProfileComponent, including inputs and methods.
GPT will create the following documentation:
## UserProfileComponent
### Inputs
- **`name: string`** - The name of the user.
- **`email: string`** - The email address of the user.
- **`profilePictureUrl: string`** - The URL of the user's profile picture.
- **`users: Array<{ name: string, email: string, profilePictureUrl: string }>`** - An array of user objects.
### Methods
- **`filterUsers(searchQuery: string): Array<{ name: string, email: string, profilePictureUrl: string }>`**
- Filters the `users` array based on the provided search query.
- **Parameters**: `searchQuery` - The search string to filter users by.
- **Returns**: An array of users whose names include the search query (case insensitive).
Conclusion
Building Angular components with AI assistance exemplifies the power of cloving—leveraging human creativity and intuition along with AI’s computational prowess. By integrating GPT into your application development workflow, you can enhance productivity, reduce errors, and stay current with best practices. Embrace cloving and discover how this collaborative approach can revolutionize your Angular development experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the prompts you see above:
How can I integrate Angular Material with this component?
And here is another:
Generate E2E tests for this component using Protractor.
And one more:
What are other GPT prompts I could use to streamline Angular development?
Note:
Whenever you see “[code snippet]” in the above prompts, replace it with the corresponding code snippets to facilitate a more comprehensive output from GPT.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.