How to Automate Frontend Code Generation for Angular with GPT
Updated on March 30, 2025

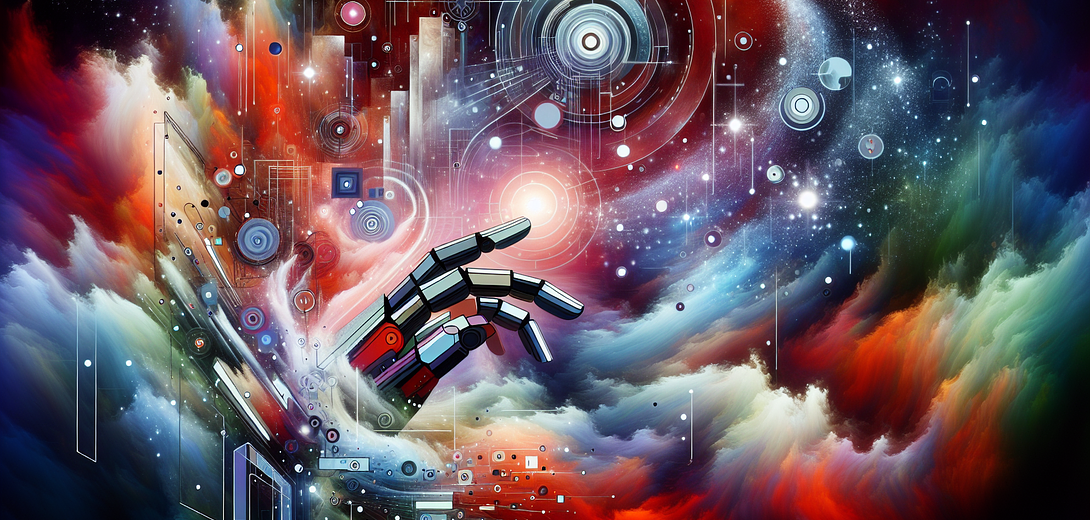
Accelerating Angular Development with Cloving CLI
Angular is a popular framework for building feature-rich web applications. However, as your project grows, creating and maintaining components, services, and tests can become time-consuming. Cloving CLI, an AI-powered command-line interface, streamlines these tasks by generating and refining your Angular code using GPT-based models. In this tutorial, we’ll explore how to integrate Cloving CLI into your Angular workflow to boost productivity and enhance code quality.
1. Introduction to Cloving CLI
Cloving CLI is a tool designed to act like an AI “pair programmer,” assisting developers in:
- Generating Angular code (like components, modules, services)
- Reviewing existing code for improvements
- Refining code interactively via a chat environment
- Creating unit tests for your Angular components
- Suggesting commit messages for improved version control
By integrating Cloving into your Angular project, you minimize boilerplate and expedite routine tasks.
2. Setting Up Cloving CLI
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
After installing, configure Cloving by providing your API key and AI model:
cloving config
Follow the interactive steps to finalize your environment (e.g., GPT-3.5, GPT-4). Once done, Cloving can generate code and handle advanced tasks for your Angular app.
3. Initializing Your Angular Project
Navigate to your Angular project root (where package.json
and your src/
folder typically reside), and run:
cloving init
Cloving analyzes your folder structure and code, creating a cloving.json
with metadata about your Angular app. This allows Cloving to produce relevant, context-aware code.
4. Generating Angular Components with Cloving
4.1 Example: User Profile Component
Suppose you need a user profile component. Use cloving generate code
:
cloving generate code --prompt "Create an Angular component for a user profile" --files src/app/components/user-profile/user-profile.component.ts
Sample Output:
// src/app/components/user-profile/user-profile.component.ts
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-user-profile',
templateUrl: './user-profile.component.html',
styleUrls: ['./user-profile.component.css']
})
export class UserProfileComponent {
@Input() user: { name: string, email: string, age: number };
constructor() {}
}
Notes:
- Cloving automatically sets up basic scaffolding (component decorator, @Input property).
- You can specify more details (like if you want a constructor that sets default values or additional methods) in your prompt.
4.2 Enhancing the Generated Component
If you need new features or data-binding logic, mention them in your prompt or refine the generated code using an interactive session (see section 6).
5. Unit Testing with Cloving
5.1 Generating Test Stubs
Robust testing ensures stable Angular apps. Let Cloving produce test scaffolding:
cloving generate unit-tests -f src/app/components/user-profile/user-profile.component.ts
Sample Output:
// src/app/components/user-profile/user-profile.component.spec.ts
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { UserProfileComponent } from './user-profile.component';
describe('UserProfileComponent', () => {
let component: UserProfileComponent;
let fixture: ComponentFixture<UserProfileComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [ UserProfileComponent ]
}).compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(UserProfileComponent);
component = fixture.componentInstance;
component.user = { name: 'John Doe', email: '[email protected]', age: 30 };
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('should display user information', () => {
const compiled = fixture.nativeElement;
expect(compiled.querySelector('.user-name').textContent).toContain('John Doe');
expect(compiled.querySelector('.user-email').textContent).toContain('[email protected]');
});
});
Notes:
- Cloving references standard Angular test patterns (e.g.,
TestBed
). - Expand these tests to cover more scenarios, such as error conditions or advanced data-binding logic.
6. Interactive Code Generation and Revision
6.1 Interactive Chat
For iterative tasks—like adding new fields, hooking into a service, or applying advanced logic—open a chat:
cloving chat -f src/app/components/user-profile/user-profile.component.ts
In the chat, you can:
- Ask for usage of Angular services or advanced patterns
- Refine performance or handle state changes
- Add or remove inputs, outputs, or lifecycle hooks
Example:
cloving> Add an @Output event emitter for user updated events
Cloving then modifies your code, injecting an @Output() userUpdated = new EventEmitter<User>();
plus a method to emit the updated data.
7. Streamlining Git Operations
7.1 Generating Commit Messages
When you’re ready to commit your changes, let Cloving propose a descriptive message:
cloving commit
Cloving inspects your staged code differences, offering a contextual summary (like “Add user profile component and associated tests.”). You can accept or refine it as needed.
8. Best Practices for Angular + Cloving
- Initialize in Your Angular Project Root
Runningcloving init
ensures the AI recognizes yoursrc/
folder,angular.json
, and any relevant config. - Iterate
If your app uses advanced patterns (like NGRX, Nx monorepo structure), mention them in your prompts or show a sample reference file for Cloving to follow. - Review
Usecloving generate review
to scan for potential issues or missed best practices (like unsubscribing from Observables). - Custom Prompts
For specialized tasks, include details in your prompt (e.g., “Create a form group with name, email fields, plus validations.”). - Extend Tests
The basic test stubs from Cloving are a foundation. Expand coverage for edge cases (e.g., invalid data, user interactions, or Angular lifecycle checks).
9. Example Workflow
Here’s a typical approach to adopting Cloving in an Angular environment:
- Initialize:
cloving init
in your Angular project root. - Generate:
cloving generate code --prompt "Create an Angular service for product management" --files src/app/services/product.service.ts
. - Test:
cloving generate unit-tests -f src/app/services/product.service.ts
for coverage. - Refine: Use
cloving chat -f src/app/services/product.service.ts
to handle advanced logic or external APIs. - Commit: Summarize changes with
cloving commit
. - Review:
cloving generate review
if you want a final pass on your code’s performance or style.
10. Conclusion
By integrating Cloving CLI into your Angular workflow, you can significantly reduce repetitive coding tasks, generate robust unit tests, and keep your code clean and maintainable. Cloving’s GPT-based intelligence helps accelerate Angular development, freeing you to focus on design, architecture, and user experience.
Remember: Cloving’s AI suggestions are a starting point; always review and adapt code or tests to your project’s domain requirements and best practices. By merging AI-driven code generation with your expertise, you’ll achieve a streamlined, high-quality Angular development process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.