How to Accelerate PHP Laravel Development with GPT's Code Generation
Updated on March 30, 2025

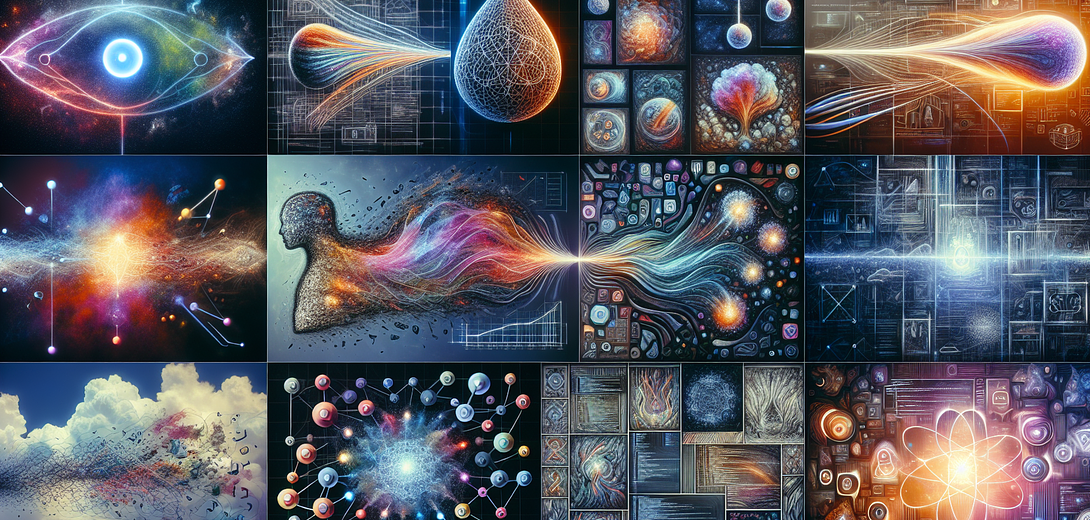
Enhancing Laravel Development with Cloving CLI
Laravel is a powerful PHP framework that simplifies many aspects of building robust web applications, from routing and templating to database operations. However, you can further optimize your workflow by integrating Cloving CLI, an AI-powered command-line interface, which handles code generation, test creation, and iterative improvements using GPT-based models. In this post, we’ll discuss how Cloving CLI can streamline your Laravel development and free you to focus on higher-level application design.
1. Understanding Cloving CLI
Cloving CLI acts as an AI “pair programmer,” offering:
- Code Generation – from controllers and models to migrations
- Test Creation – scaffolding test files for your Laravel components
- Code Review – scanning for potential improvements
- Interactive Refinement – through a chat-based environment
- AI-powered Commit Messages – ensuring consistent, descriptive version control history
By capturing your project’s context, Cloving can tailor code outputs to your existing Laravel conventions and best practices.
2. Setting Up Cloving for Laravel
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
Next, configure Cloving with your API key and chosen AI model (e.g., GPT-3.5, GPT-4):
cloving config
Follow the prompts to finalize your environment. Once set up, Cloving is equipped to generate or improve Laravel code.
2.3 Initialization
In your Laravel project root (where composer.json
, .env
, and the app/
, routes/
, database/
folders typically reside), run:
cloving init
Cloving scans your project structure and creates a cloving.json
that contains essential metadata. This ensures that Cloving’s code generation aligns with your Laravel application context.
3. AI-Powered Code Generation in Laravel
3.1 Example: Creating a Laravel Controller
Let’s say you need a new controller for managing users. Instead of manual scaffolding, prompt Cloving:
cloving generate --prompt "Create a Laravel controller for managing users" --files src/Http/Controllers
Sample Output:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index()
{
$users = User::all();
return view('users.index', compact('users'));
}
public function create()
{
return view('users.create');
}
public function store(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email|unique:users,email',
]);
User::create($validatedData);
return redirect()->route('users.index');
}
public function show($id)
{
$user = User::findOrFail($id);
return view('users.show', compact('user'));
}
public function edit($id)
{
$user = User::findOrFail($id);
return view('users.edit', compact('user'));
}
public function update(Request $request, $id)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email|unique:users,email,' . $id,
]);
$user = User::findOrFail($id);
$user->update($validatedData);
return redirect()->route('users.index');
}
public function destroy($id)
{
$user = User::findOrFail($id);
$user->delete();
return redirect()->route('users.index');
}
}
Notes:
- Cloving references typical Laravel patterns (e.g.,
Request
,User
model). - Expand or refine each method in subsequent prompts or the interactive chat, e.g., adding roles or advanced validations.
3.2 Additional Example: Generating a Model
If you also need a model for your domain, Cloving can generate or refine it:
cloving generate --prompt "Create a Laravel model named Project with a fillable name field" --files app/Models
Cloving might produce a Project.php
with:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Project extends Model
{
use HasFactory;
protected $fillable = [
'name'
];
}
Mention any relationships (belongsTo, hasMany) or advanced features in your prompt if needed.
4. Adding Unit Tests for Laravel
4.1 Generating Tests
Cloving also automates test creation. Suppose you want basic test coverage for UserController
:
cloving generate unit-tests -f src/Http/Controllers/UserController.php
Sample Output:
<?php
namespace Tests\Unit;
use Tests\TestCase;
use App\Models\User;
use App\Http\Controllers\UserController;
use Illuminate\Http\Request;
class UserControllerTest extends TestCase
{
public function testIndexReturnsUsers()
{
$response = $this->get('/users');
$response->assertStatus(200);
$response->assertViewHas('users');
}
public function testStoreCreatesUser()
{
$response = $this->post('/users', [
'name' => 'John Doe',
'email' => 'john@example.com'
]);
$response->assertRedirect('/users');
$this->assertDatabaseHas('users', ['email' => 'john@example.com']);
}
}
Notes:
- Cloving references the standard Laravel
TestCase
class. - If your code uses Dusk or other specialized testing libraries, specify them.
5. Managing Database Migrations
5.1 Example: Adding a “profile” column to “users” table
For simpler schema changes, let Cloving generate migrations:
cloving generate --prompt "Create a Laravel migration to add a 'profile' column to the 'users' table"
Sample Output:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddProfileToUsersTable extends Migration
{
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->text('profile')->nullable();
});
}
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('profile');
});
}
}
Run php artisan migrate
to apply the changes. If you need advanced logic (e.g., indexing, foreign keys), mention them in your prompt.
6. Enhancing Code via Cloving Chat
6.1 Interactive Sessions
For iterative tasks—like refining complex queries, hooking up advanced Eloquent relationships, or implementing caching—open Cloving’s chat:
cloving chat -f src/Http/Controllers/UserController.php
Within this environment, you can:
- Ask about performance improvements (like Eager Loading or chunking queries)
- Add or remove advanced validations
- Refine your route definitions or model relationships
Example:
cloving> Modify the index method to paginate users, returning 15 per page
Cloving might produce an updated index method using $users = User::paginate(15);
.
7. Optimizing Git Commits
7.1 AI-Suggested Commit Messages
After finalizing or adjusting code, let Cloving generate a commit message summarizing changes:
cloving commit
Cloving checks your staged changes (like newly created or updated controller, tests, etc.), offering a descriptive message, e.g. “Add user creation and retrieval methods with test coverage in UserController.” You can revise or accept it before committing.
8. Best Practices for Laravel + Cloving
- Initialize Each Project
If you have multiple Laravel apps, runcloving init
in each so the AI recognizes that codebase’s structure. - Iterate
Start with basic code generation, then refine or expand features using either repeated prompts or the chat environment. - Review
Usecloving generate review
if you want an AI-based overview of potential improvements or missing best practices in your newly generated code. - Consider Domain Complexity
If your app uses advanced domain logic (like custom request classes, repositories, or DDD patterns), mention them in your prompt or show Cloving existing examples. - Extend Tests
Cloving’s test scaffolding is helpful but limited – expand coverage for negative scenarios, concurrency, or advanced logic as you see fit.
Conclusion
By integrating Cloving CLI into your Laravel workflow, you drastically reduce boilerplate and expedite code generation. Cloving’s GPT-driven approach frees you from manual scaffolding of controllers, migrations, and test files, letting you concentrate on architectural decisions and domain logic.
Remember: Cloving’s output is a foundation—always validate it for domain-specific correctness, performance constraints, and alignment with your project’s style. Embrace AI in your Laravel development, and experience a more streamlined, high-quality coding process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.