Harnessing GPT to Automate Golang Code Tasks
Updated on January 16, 2025

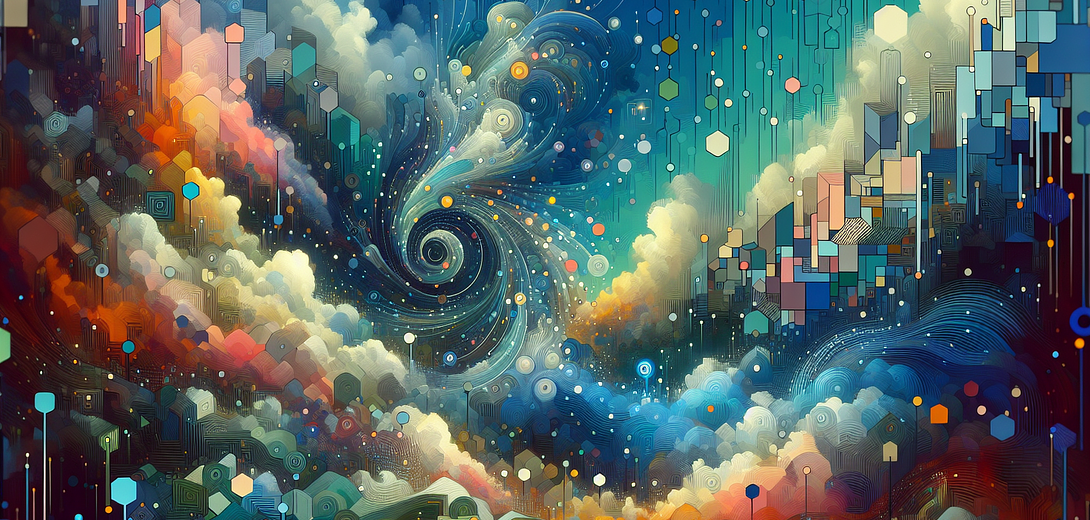
As a Golang developer, you’re always looking for ways to streamline your workflow and improve code quality. Cloving CLI offers a powerful solution by integrating AI into your command-line environment. With Cloving, you can leverage GPT to automate repetitive coding tasks, generate code snippets, perform code reviews, and even conduct contextual chats with your AI pair programmer. In this tutorial, we will explore how to effectively utilize Cloving in your Go projects to enhance productivity and efficiency.
Getting Started with Cloving
1. Installation and Configuration
First, install Cloving globally using npm:
npm install -g cloving@latest
Once installed, configure Cloving with your API key and preferred GPT models:
cloving config
Follow the prompts to input your API key and choose the model that best fits your needs.
2. Initializing Your Go Project
Next, initialize Cloving in your Go project directory to provide context:
cloving init
This command analyzes your project structure and creates a cloving.json
file that aids in context-aware code generation.
Automating Code Tasks with Cloving
3. Generating Golang Code
Imagine you need a function to fetch data from an API and handle errors gracefully. Instead of writing it from scratch, use Cloving:
cloving generate code --prompt "Create a Golang function to fetch JSON data from an API and handle errors" --files main.go
Cloving will generate code based on your project context:
package main
import (
"encoding/json"
"fmt"
"net/http"
"io/ioutil"
)
func fetchData(url string) (map[string]interface{}, error) {
resp, err := http.Get(url)
if err != nil {
return nil, fmt.Errorf("failed to fetch data: %v", err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
return nil, fmt.Errorf("failed to read response: %v", err)
}
var data map[string]interface{}
if err := json.Unmarshal(body, &data); err != nil {
return nil, fmt.Errorf("failed to parse JSON: %v", err)
}
return data, nil
}
4. Testing with Code Generation
Writing unit tests is crucial for maintaining robust code. Cloving can automate test generation for your Go functions:
cloving generate unit-tests -f utils.go
This command produces meaningful unit tests based on your function’s logic:
package main
import (
"testing"
)
func TestFetchData(t *testing.T) {
url := "https://api.example.com/data"
result, err := fetchData(url)
if err != nil {
t.Fatalf("Expected no error, got %v", err)
}
if len(result) == 0 {
t.Errorf("Expected non-empty result, got empty")
}
}
5. Using Cloving Chat for Complex Tasks
For intricate programming challenges, engage Cloving’s chat feature:
cloving chat -f main.go
This opens a session where you can directly converse with your AI assistant. Use queries like:
cloving> Can you suggest optimizations for the fetchData function?
Cloving will provide insightful recommendations, enhancing your code further.
Streamlining Your Workflow with Cloving
6. Automated Code Reviews
Conducting code reviews manually can be labor-intensive. Utilize Cloving for AI-driven code reviews:
cloving generate review
Receive comprehensive feedback and suggestions to refine your codebase.
7. Creating Contextual Commit Messages
Enhance your commit messages with contextual understanding through Cloving:
cloving commit
This command drafts a meaningful commit message reflecting the changes made, reducing the cognitive load involved in summarizing updates.
Conclusion
By integrating Cloving CLI into your Golang development workflow, you’re not just automating mundane tasks but elevating your overall productivity. Whether it’s generating sophisticated code snippets, refining existing implementations, or creating intelligent commit messages, Cloving is your go-to tool.
Embrace the power of AI in programming—let Cloving transform your coding experience in the dynamic world of Golang development. Remember, while Cloving enhances your workflow, it complements rather than replaces your expertise. Use it to augment your capabilities and streamline your development processes efficiently.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.