Harnessing GPT for JSON API Generation in Golang Backend Services
Updated on January 02, 2025

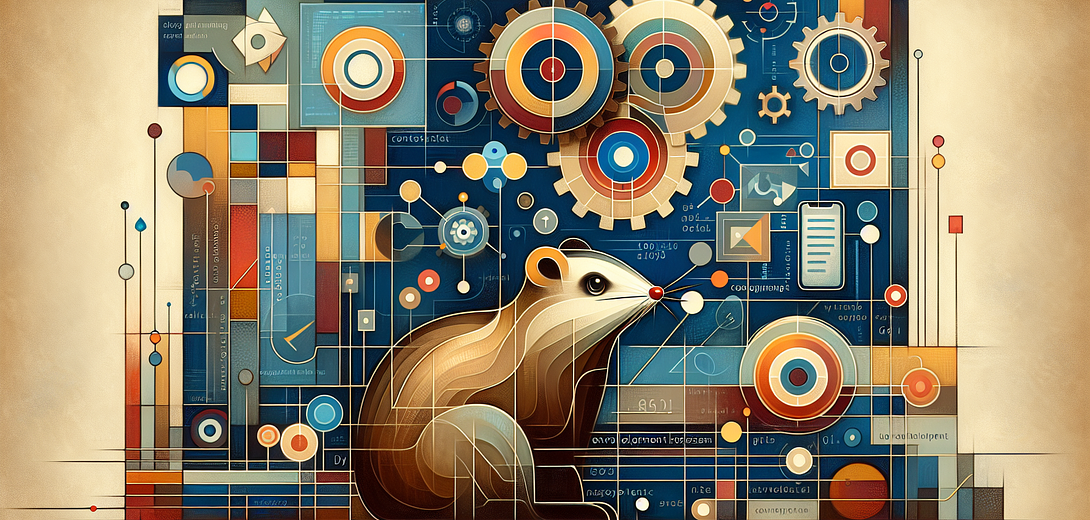
In the realm of backend development, creating APIs is a frequent but often tedious task. Enter the Cloving CLI, a powerful AI-driven tool that can supercharge your API development process in Golang. By harnessing Generative Pre-trained Transformers (GPT), Cloving simplifies generating JSON APIs, allowing developers to meet project requirements swiftly and effectively. Let’s dive into leveraging Cloving to enhance your Golang backend services.
Setting Up Cloving for Golang Projects
Before utilizing Cloving for API generation, you need to set it up in your environment.
Installation
Ensure you have Node.js installed, then install Cloving globally using npm:
npm install -g cloving@latest
Configuration
To configure Cloving for your API work:
cloving config
Follow the prompts to select your AI model and link your API key. This setup is crucial as it defines the model Cloving will use for generating APIs.
Initializing the Project
After configuration, initialize Cloving in your Golang project directory to provide necessary context:
cloving init
This command creates a cloving.json
file that outlines the project’s settings and context, preparing Cloving to generate code specific to your Golang backend.
Efficient JSON API Generation with Cloving
Once set up, you can begin generating APIs using Cloving’s generate
command. This feature is particularly powerful as it personalizes code snippets to your Golang architecture.
Example: Generate a Simple JSON API
Suppose you need to create an endpoint for a customer resource in your Golang service. Using Cloving, proceed as follows:
cloving generate code --prompt "Create a Golang JSON API for customer resource: GET, POST, PUT, DELETE" --files handlers/customer.go
This prompt instructs Cloving to generate CRUD operations for a customer resource in your specified file. A typical result could be:
package handlers
import (
"net/http"
"encoding/json"
"github.com/gorilla/mux"
)
type Customer struct {
ID string `json:"id"`
Name string `json:"name"`
Email string `json:"email"`
}
var customers = []Customer{}
func GetCustomers(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(customers)
}
func CreateCustomer(w http.ResponseWriter, r *http.Request) {
var customer Customer
_ = json.NewDecoder(r.Body).Decode(&customer)
customers = append(customers, customer)
json.NewEncoder(w).Encode(customer)
}
func UpdateCustomer(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
for index, item := range customers {
if item.ID == params["id"] {
customers = append(customers[:index], customers[index+1:]...)
var customer Customer
_ = json.NewDecoder(r.Body).Decode(&customer)
customer.ID = params["id"]
customers = append(customers, customer)
json.NewEncoder(w).Encode(customer)
return
}
}
}
func DeleteCustomer(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
for index, item := range customers {
if item.ID == params["id"] {
customers = append(customers[:index], customers[index+1:]...)
break
}
}
json.NewEncoder(w).Encode(customers)
}
Revising and Enhancing Generated Code
Cloving goes beyond mere generation; it supports revising code. If adjustments are needed, simply prompt the tool for enhancements like adding authentication.
Add JWT authentication for customer API endpoints.
Cloving will amend your handlers to incorporate JWT checks, bolstering security in your API.
Chat-Based API Development
For more dynamic and real-time assistance, utilize Cloving’s chat feature. Engage directly with the AI to generate nuanced and refined API components:
cloving chat -f handlers/customer.go
In chat mode, interactively develop and refine API endpoints, ensuring your code aligns precisely with your backend blueprint.
cloving> Refactor API endpoints to include error handling and logging.
Optimize Productivity with Commit Messages
Once your API is complete, leverage Cloving’s commit feature to generate insightful commit messages:
cloving commit
This command analyzes changes such as API functionality implementations and auto-suggests context-rich commit details, saving you time and enhancing documentation quality.
Conclusion
Harnessing Cloving CLI for JSON API development in Golang empowers developers to efficiently craft sophisticated backend services with minimal coding errors. By leveraging AI, you can streamline your production process, produce superior code, and maintain competitive development speeds. Integrate Cloving CLI into your workflow to transform how you generate and maintain Golang backend services.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.