Harnessing GPT for Effortless Kotlin App Development
Updated on November 17, 2024

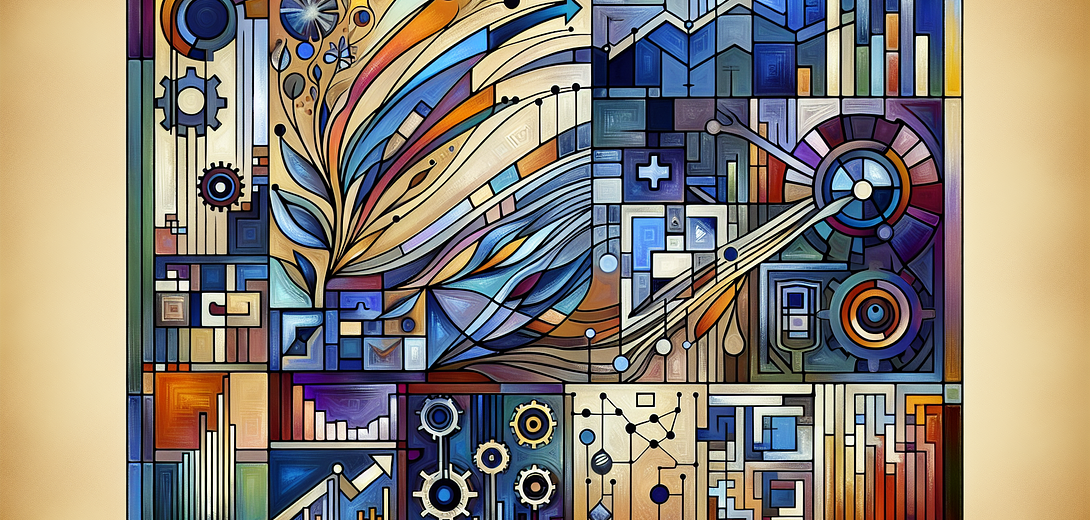
As developers, we constantly seek tools that can simplify and enhance our development processes. Enter Cloving CLI, an AI-powered command-line interface tool designed to integrate AI into your workflow, making app development fast and efficient. In this blog post, we’ll focus on how Cloving can be leveraged to assist in Kotlin app development, offering practical examples, tips, and best practices.
Getting Started with Cloving CLI
Before diving into the wonders of AI-assisted Kotlin programming, let’s set up Cloving in your development environment.
1. Install and Configure Cloving
First, ensure Cloving is installed globally:
npm install -g cloving@latest
Following installation, configure Cloving to use your preferred AI model and API key by running:
cloving config
This setup process is crucial as it allows you to specify the models that Cloving will employ to generate code.
2. Initialize Your Kotlin Project
To provide Cloving with the necessary context about your project, initialize it in your Kotlin project’s root directory:
cloving init
This command analyzes your project structure and creates a cloving.json
configuration file that contains essential information about your application to facilitate better code generation.
Effective Kotlin Development with Cloving
With configuration out of the way, it’s time to put Cloving to work for us in Kotlin development.
3. Generating Kotlin Code Snippets
Cloving’s generate code
command is powerful for generating functional Kotlin code snippets based on your prompts. Let’s say you’re working on a Kotlin Android app and need to create a basic RecyclerView adapter. Use Cloving like this:
cloving generate code --prompt "Create a basic RecyclerView adapter for displaying a list of strings" --files app/src/main/java/com/example/myapp/MainAdapter.kt
This will generate a Kotlin class, such as:
package com.example.myapp
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.recyclerview.widget.RecyclerView
import com.example.myapp.databinding.ItemStringBinding
class MainAdapter(private val items: List<String>) : RecyclerView.Adapter<MainAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val binding = ItemStringBinding.inflate(LayoutInflater.from(parent.context), parent, false)
return ViewHolder(binding)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.bind(items[position])
}
override fun getItemCount(): Int = items.size
class ViewHolder(private val binding: ItemStringBinding) : RecyclerView.ViewHolder(binding.root) {
fun bind(item: String) {
binding.textView.text = item
}
}
}
4. Revising and Enhancing Code
Once you have the generated code, you can review and refine it. Cloving makes revisions simple. For example, if you want to extend the RecyclerView
adapter to include click listeners, you can initiate an interactive session:
cloving chat -f app/src/main/java/com/example/myapp/MainAdapter.kt
In the chat session, ask Cloving to add click listener functionality:
Add an on-click listener to each item in the RecyclerView adapter.
5. Generating Unit Tests for Kotlin Code
Testing is an integral part of development. Cloving can also assist in generating unit tests, ensuring that your code performs as expected. To create unit tests for your adapter:
cloving generate unit-tests -f app/src/main/java/com/example/myapp/MainAdapter.kt
The outcome may appear as follows:
package com.example.myapp
import org.junit.Assert.assertEquals
import org.junit.Before
import org.junit.Test
import org.mockito.Mockito.mock
class MainAdapterTest {
private lateinit var adapter: MainAdapter
private val mockContext = mock(Context::class.java)
@Before
fun setUp() {
val items = listOf("Item 1", "Item 2", "Item 3")
adapter = MainAdapter(items)
}
@Test
fun `getItemCount returns correct item count`() {
assertEquals(3, adapter.itemCount)
}
}
6. Utilizing Cloving for Commit Messages
Cloving can also aid in writing concise and descriptive commit messages. This is particularly useful for maintaining clarity in version control. Instead of typing a commit message manually, use:
cloving commit
Here’s how GPT might help generate commit messages at each step:
-
After Initial Code Generation:
- “Added initial version of RecyclerView adapter for string display.”
- “Generated basic Kotlin RecyclerView adapter class.”
-
After Code Revision:
- “Enhanced RecyclerView adapter with click listener functionality.”
- “Refactored adapter class to include item click handling.”
-
After Unit Test Generation:
- “Added unit tests for RecyclerView adapter item count verification.”
- “Generated unit tests to ensure adapter integrity and functionality.”
Cloving will analyze the recent changes and propose a relevant and informative commit message, reducing the time spent on commit documentation.
Conclusion
Harnessing the Cloving CLI for Kotlin development significantly enhances productivity and code quality by integrating AI-driven insights directly into your workflow. Whether you’re generating code, refining it, or ensuring its robustness with unit tests, Cloving acts as an intelligent co-coder, empowering you to focus more on creativity and less on repetitive tasks.
Start leveraging Cloving today and experience the ease and efficiency of AI-enhanced Kotlin app development! Remember, while Cloving is a powerful tool, it complements rather than replaces your coding expertise. Let it augment your workflow and elevate your coding projects to new heights.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.