Harnessing GPT for Automated TypeScript Component Generation
Updated on March 05, 2025

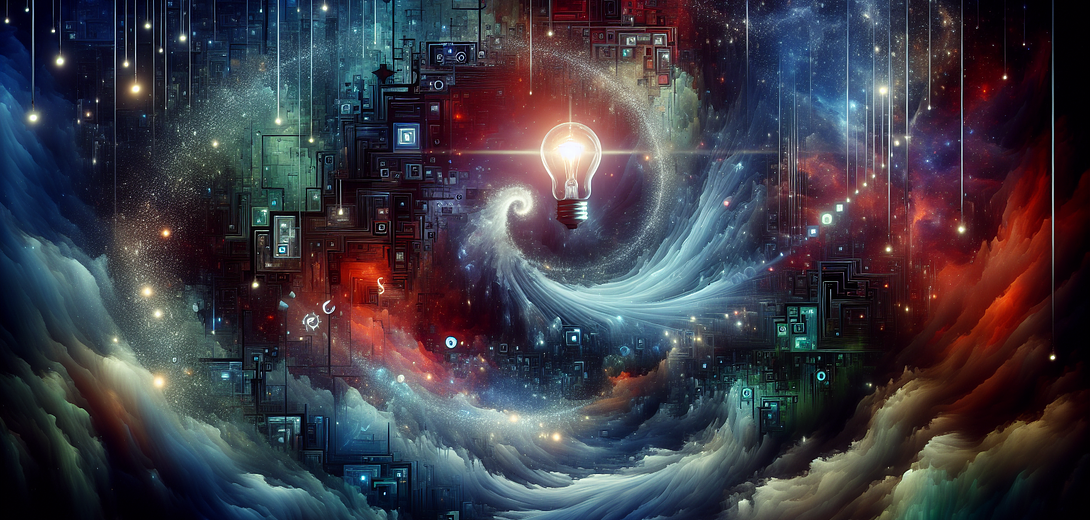
Below is an expanded version of the post, featuring additional best practices, examples, and tips to help you harness the full potential of Cloving CLI for generating and testing TypeScript components.
Accelerating TypeScript Component Development with Cloving CLI
In today’s fast-paced development environment, high-quality and efficient code is essential. Tools like Cloving CLI—which integrates GPT models—can automate the boilerplate elements of TypeScript development, allowing you to focus on more complex tasks. In this guide, we’ll explore how Cloving can help you quickly create and refine TypeScript components, generate unit tests, and provide real-time assistance via its interactive chat feature.
1. Getting Started with Cloving CLI
1.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
1.2 Configuration
Next, configure Cloving to work with your preferred AI models and credentials:
cloving config
Follow the prompts to enter your API key and select the GPT model that fits your needs (e.g., GPT-3.5, GPT-4).
1.3 Project Initialization
Navigate to your TypeScript project directory and initialize Cloving:
cd /path/to/typescript/project
cloving init
This creates a cloving.json
file, storing project metadata. With this context, Cloving tailors generated code to match your conventions more closely.
2. Generating TypeScript Components
Once Cloving is set up, you can create TypeScript components with minimal effort—be it React, Vue, or any other TypeScript-compatible framework.
2.1 Example: Creating a React Functional Component
Suppose you’re building a user profile component to display name, age, and email. Instead of manually writing boilerplate, let Cloving generate it for you:
cloving generate code --prompt "Create a TypeScript component for a UserProfile that displays user name, age, and email" --files src/components/UserProfile.tsx
Sample Output:
// src/components/UserProfile.tsx
import React from 'react';
interface UserProfileProps {
name: string;
age: number;
email: string;
}
const UserProfile: React.FC<UserProfileProps> = ({ name, age, email }) => {
return (
<div>
<h2>{name}</h2>
<p>Age: {age}</p>
<p>Email: {email}</p>
</div>
);
};
export default UserProfile;
2.1.1 Refining Your Component
You can easily refine or extend the generated code. For instance, to add an optional bio
field:
Revise the UserProfile component to include an optional bio field.
Cloving updates the interface and markup to incorporate bio
(e.g., bio?: string;
).
2.2 Non-React Components
Cloving can also generate TypeScript classes or standalone utility functions. For example:
cloving generate code --prompt "Create a TypeScript utility function to format dates into a readable string" --files src/utils/dateFormatter.ts
Sample Output:
// src/utils/dateFormatter.ts
export function formatDate(date: Date): string {
return date.toLocaleDateString('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric'
});
}
3. Streamlining Unit Test Creation
Comprehensive testing is essential for maintaining reliable TypeScript code. Cloving can generate unit tests based on the structure of your existing files.
3.1 Example: Generating Tests for a React Component
cloving generate unit-tests -f src/components/UserProfile.tsx
Sample Output:
// src/components/UserProfile.test.tsx
import React from 'react';
import { render, screen } from '@testing-library/react';
import UserProfile from './UserProfile';
describe('UserProfile Component', () => {
it('displays user name, age, and email', () => {
render(<UserProfile name="John Doe" age={30} email="[email protected]" />);
expect(screen.getByText('John Doe')).toBeInTheDocument();
expect(screen.getByText('Age: 30')).toBeInTheDocument();
expect(screen.getByText('Email: [email protected]')).toBeInTheDocument();
});
});
This scaffold helps you quickly validate that your component renders properly and responds to typical use cases.
3.2 Example: Testing Utility Functions
cloving generate unit-tests -f src/utils/dateFormatter.ts
Sample Output:
// src/utils/dateFormatter.test.ts
import { formatDate } from './dateFormatter';
describe('formatDate', () => {
it('formats the date into a readable string', () => {
const date = new Date('2023-01-01');
const formatted = formatDate(date);
expect(formatted).toMatch(/January 1, 2023/);
});
});
4. Leveraging Cloving Chat for Advanced Assistance
Complex tasks often require an iterative approach. Cloving’s interactive chat mode allows you to refine, explain, or discuss potential solutions in real time.
4.1 Example: Real-Time Problem Solving
cloving chat -f src/components/UserProfile.tsx
Here, you can ask:
I want the UserProfile to display the user's bio only if provided, with a default fallback otherwise.
Cloving will then propose modifications to the interface and component, adding conditional rendering for the bio
property.
5. Best Practices and Tips
-
Provide Detailed Prompts
The more specific your prompts—e.g., specifying framework, styling preferences, or interface definitions—the more accurate the generated code. -
Iterate with Chat
Use short iterative steps in the chat to gradually build or refactor components, ensuring each revision is well-focused. -
Use
cloving commit
for Meaningful Messages
Leverage Cloving’s AI-driven commit suggestions to maintain a tidy commit history. For example:cloving commit
You’ll receive a suggested commit message summarizing the changes.
-
Integrate with Your Existing Toolchain
Cloving can coexist with tools like ESLint, Prettier, and your build system. Just ensure your environment is configured to run type checking and linting on generated files. -
Review and Validate
While Cloving produces strong starting points, always review logic, perform thorough testing, and ensure compliance with your domain requirements.
6. Example Workflow
A typical Cloving-driven development cycle might look like this:
- Initialize:
cloving init
to createcloving.json
. - Generate Components: Use
cloving generate code
to scaffold new components. - Refine: Make iterative changes with
cloving chat
. - Test:
cloving generate unit-tests
to produce a test suite. - Commit:
cloving commit
for AI-generated commit messages. - Deploy: Integrate with your CI/CD pipeline and check for any final issues.
7. Conclusion
By integrating Cloving CLI into your TypeScript development flow, you’ll accelerate component creation, standardize coding patterns, and maintain a robust test suite. The AI-driven approach allows you to focus on business logic and feature innovation, while Cloving automates repetitive or boilerplate tasks.
Embrace Cloving to streamline your TypeScript workflow and experience the transformative benefits of AI-assisted coding. With Cloving’s flexible code generation, test scaffolding, and interactive support, your projects can achieve new levels of speed, consistency, and maintainability.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.