Harnessing AI to Simplify the Creation of TailwindCSS Components
Updated on March 05, 2025

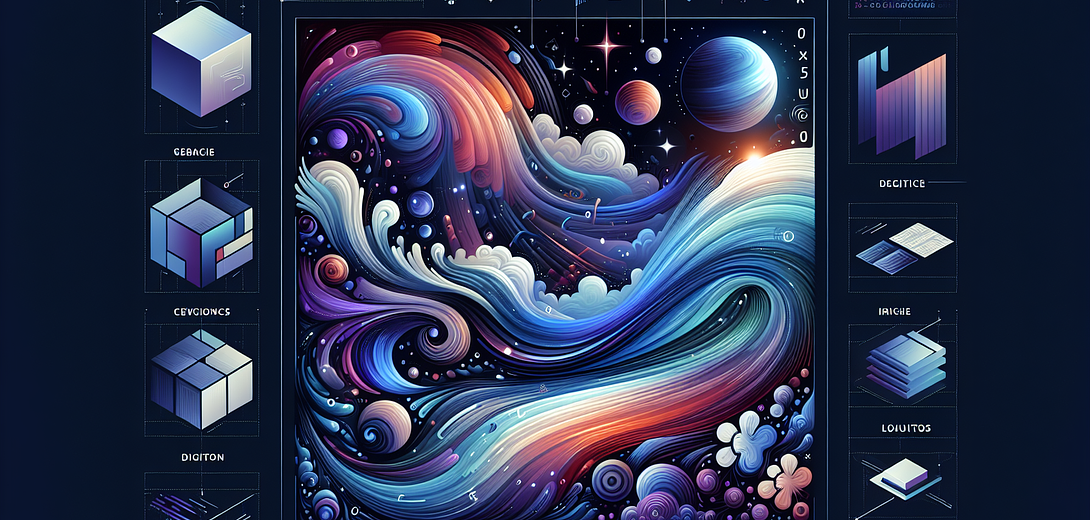
Streamlining TailwindCSS Component Development with Cloving CLI
TailwindCSS simplifies your CSS workflow by providing utility classes for rapid UI development, but crafting reusable components with consistent styling can still be time-consuming. Cloving CLI – an AI-powered command-line tool – can help you automate repetitive tasks, reduce boilerplate, and maintain a cleaner workflow as you build TailwindCSS-based components. In this guide, we’ll explore how to integrate Cloving into your TailwindCSS projects and unlock AI-driven productivity gains.
1. Getting Started with Cloving CLI
1.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
1.2 Configuration
Next, run:
cloving config
Here, you’ll provide your API key and select an AI model (e.g., GPT-3.5, GPT-4) that Cloving will use to generate code snippets tailored to your needs.
1.3 Initialize Your Project
To ensure Cloving understands your project’s context, navigate to the root of your TailwindCSS application and initialize Cloving:
cloving init
This creates a cloving.json
file containing relevant metadata about your project (e.g., directories, frameworks used), enabling Cloving to provide more accurate and context-aware suggestions.
2. Building TailwindCSS Components with Cloving
Cloving excels at automating code generation for repetitive tasks, such as setting up base components or scaffolding new UI features. Below, we’ll explore how to create a TailwindCSS button component using Cloving.
2.1 Example: Generating a TailwindCSS Button
Suppose you want a reusable button with primary, secondary, and disabled variants. Use Cloving’s generate command:
cloving generate code --prompt "Create a TailwindCSS button component with variants for primary, secondary, and disabled states" --files src/components/Button.tsx
Sample Output:
// src/components/Button.tsx
import React from 'react';
interface ButtonProps {
variant: 'primary' | 'secondary' | 'disabled';
children: React.ReactNode;
}
const Button: React.FC<ButtonProps> = ({ variant, children }) => {
const baseClasses = 'px-4 py-2 rounded text-white font-semibold';
const variantClasses = {
primary: 'bg-blue-500 hover:bg-blue-600',
secondary: 'bg-gray-500 hover:bg-gray-600',
disabled: 'bg-gray-300 cursor-not-allowed',
};
return (
<button className={`${baseClasses} ${variantClasses[variant]}`}>
{children}
</button>
);
};
export default Button;
Notes:
- Cloving references your project’s structure, aiming to fit your naming or code style.
- You can tweak classes or add new utility classes, e.g.,
text-xl
oruppercase
.
2.2 Refining Generated Code
After generation, you can:
- Explain: Ask Cloving to clarify any piece of the generated code.
- Revise: Use
cloving chat
to request changes (e.g., different color classes, extra states). - Save: Commit the final code snippet to your files.
3. More Advanced TailwindCSS Usage
While generating a simple button is a quick example, Cloving can also assist with more complex UI patterns:
3.1 Responsive Layout Classes
For instance, you might need a responsive grid layout. You can prompt Cloving to generate utility classes or a utility-based layout component:
cloving generate code --prompt "Generate a responsive grid layout component using TailwindCSS" --files src/components/GridLayout.tsx
Sample Output:
// src/components/GridLayout.tsx
import React from 'react';
interface GridLayoutProps {
children: React.ReactNode;
}
const GridLayout: React.FC<GridLayoutProps> = ({ children }) => {
return (
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-4 gap-4 p-4">
{children}
</div>
);
};
export default GridLayout;
Tailwind utility classes ensure consistent spacing, and Cloving can adapt the grid definition to your preferences (columns, responsive breakpoints, etc.).
3.2 Tailwind Config Customizations
If you have a tailwind.config.js file with custom themes, color palettes, or spacing values, mention them in your prompt so Cloving can incorporate them into generated code.
cloving generate code --prompt "Create a hero banner component with brand colors from tailwind.config.js" --files src/components/HeroBanner.tsx
Cloving may detect or reference custom color tokens (e.g., brand-blue
, brand-yellow
) if they’re declared in your config.
4. Interactive Assistance with Cloving Chat
For real-time or iterative improvements, use the Cloving chat feature:
cloving chat -f src/components/Button.tsx
You can ask:
cloving> How can I add an outline variant to this TailwindCSS button?
Cloving may respond with new classes or an updated snippet that integrates an outline style (e.g., border-2 border-blue-500 text-blue-500 bg-transparent
).
5. Generating Meaningful Commit Messages
Frequent commits are a best practice for documenting changes. Cloving can automate commit message suggestions:
cloving commit
Cloving reviews staged changes and proposes a message such as:
Add TailwindCSS button component with primary, secondary, and disabled states
You can accept or refine this suggestion for a clear, descriptive commit history.
6. Incorporating Tests for Your Tailwind Components
While Tailwind focuses on styling, you can also test any interaction or conditional rendering logic present in your components. Cloving’s generate unit-tests command can scaffold basic test suites:
cloving generate unit-tests -f src/components/Button.tsx
Sample Output:
// src/components/Button.test.tsx
import React from 'react';
import { render, screen } from '@testing-library/react';
import Button from './Button';
describe('Button Component', () => {
it('renders children correctly', () => {
render(<Button variant="primary">Click Me</Button>);
expect(screen.getByText('Click Me')).toBeInTheDocument();
});
it('applies correct Tailwind classes for primary variant', () => {
render(<Button variant="primary">Primary</Button>);
const button = screen.getByRole('button', { name: 'Primary' });
expect(button).toHaveClass('bg-blue-500 hover:bg-blue-600');
});
it('disables functionality for disabled variant', () => {
render(<Button variant="disabled">Disabled</Button>);
const button = screen.getByRole('button', { name: 'Disabled' });
expect(button).toHaveClass('cursor-not-allowed');
});
});
Notes:
- Expand tests as needed for user interactions or advanced logic.
- Combine with tools like
@testing-library/react
for snapshot or integration tests.
7. Best Practices for Cloving + Tailwind
-
Leverage Tailwind’s Config
If you have custom utility classes or extended theme definitions (e.g., for colors, spacing), mention these in your prompt so Cloving can incorporate them. -
Iterative Code Generation
Start with a basic component, then refine or add variants, transitions, or custom breakpoints via Cloving chat. This ensures minimal editing on your part. -
Refactor Freedoms
AI-generated code is a foundation. Adapt the naming conventions, logic separation (e.g., additional props or abstracted sub-components), and advanced ARIA attributes as needed. -
Focus on Reusability
Where possible, keep components flexible. For instance, your button might accept additional classes from props to handle edge design cases. -
Monitor Performance
If you’re generating large volumes of classes or dynamic variants, consider Tailwind’s JIT mode or other performance optimizations.
8. Example Workflow with Cloving
A typical approach to building a TailwindCSS component with Cloving might be:
- Initialize:
cloving init
in project root to gather context. - Generate:
cloving generate code --prompt "Create a responsive navbar with Tailwind" -f src/components/Navbar.tsx
- Refine: Use interactive chat:
cloving chat -f src/components/Navbar.tsx
– ask for color changes, transitions, etc. - Test:
cloving generate unit-tests -f src/components/Navbar.tsx
for initial coverage. - Commit: Summarize changes with
cloving commit
.
9. Conclusion
By blending TailwindCSS’s utility-first design with Cloving CLI’s AI-powered code generation, you can drastically reduce repetitive coding and keep your UI consistent and maintainable. From scaffolding complex layout components to adding subtle style variants, Cloving automates the heavy lifting, allowing you to focus on design creativity and business logic.
Remember: Cloving complements your expertise, enabling you to move faster without sacrificing code quality. As you continue building out your TailwindCSS-based design system, consider using Cloving to accelerate component creation, testing, and iterative refinement. Embrace AI as part of your toolkit and enjoy a more productive, streamlined development experience!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.