Harnessing AI for Debugging Asynchronous Operations in Node.js
Updated on April 21, 2025

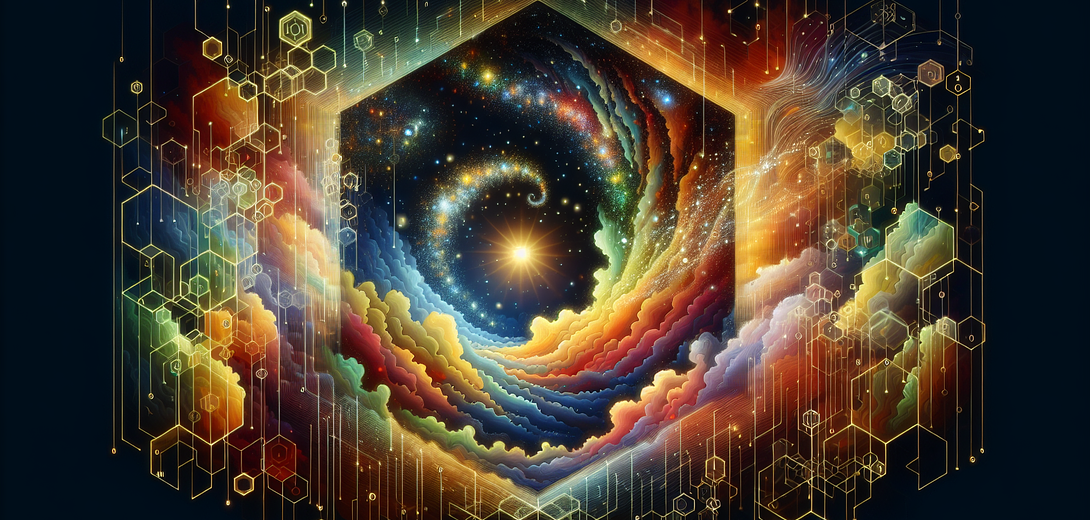
Debugging asynchronous operations is a critical skill for any Node.js developer. Asynchronous code adds complexity to an application due to the non-blocking nature of JavaScript, making it challenging to pinpoint where issues might arise. Enter Cloving CLI—a powerful AI-powered command-line tool that can enhance your Node.js development workflow, particularly when dealing with asynchronous operations. In this post, we’ll explore how to leverage Cloving CLI to debug asynchronous operations more effectively.
Setting Up the Cloving CLI
To get started, you need to set up the Cloving CLI tool in your development environment. If you haven’t already installed it, you can do so by running:
npm install -g cloving@latest
Once installed, configure Cloving by providing your API key and selecting your preferred AI models with the following command:
cloving config
Follow the prompts to complete your setup.
Initializing Your Node.js Project
Begin by initializing your Node.js project with Cloving to help it understand the context of your code:
cloving init
This will create a cloving.json
file in your project with essential metadata.
Debugging Asynchronous Code with Cloving Chat
One of the key advantages of Cloving CLI is its interactive chat
feature, allowing you to engage with AI to resolve questions around your asynchronous code.
To start a chat session, specify the files related to your asynchronous operations:
cloving chat -f src/asyncOperations.js
This command opens an interactive chat session where you can inquire about how to handle specific errors in your asynchronous code:
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
Type a freeform request or question to interact with your Cloving AI pair programmer.
cloving> How do I debug an unhandled promise rejection in `src/asyncOperations.js`?
Cloving AI: To debug an unhandled promise rejection, consider using `Promise.catch()` to handle potential errors or use the `async` and `await` pattern to streamline exception handling. I can help you add these patterns to your existing code.
Example Asynchronous Code
Suppose you have the following asynchronous function, which fetches data from an external API:
async function fetchData(url) {
const response = fetch(url);
const data = await response.json();
return data;
}
fetchData('https://api.example.com/data')
.then(data => console.log(data))
.catch(err => console.error(err));
Revising with Cloving Chat
Using Cloving chat, you can request a revision to your function to better handle promise rejections:
cloving> Revise `fetchData` to ensure all promise rejections are handled.
Cloving AI: Here's an updated version of your `fetchData` function, with improved error handling:
```javascript
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`Error ${response.status}: ${response.statusText}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Failed to fetch data:', error.message);
}
}
Generating Unit Tests for Asynchronous Functions
Testing your asynchronous functions is crucial. Cloving CLI can also help generate unit tests for your code, ensuring that your promises resolve and reject correctly.
cloving generate unit-tests -f src/asyncOperations.js
This command will create a unit test template specifically for your asynchronous code.
import { fetchData } from './asyncOperations';
describe('fetchData', () => {
test('should fetch data successfully', async () => {
const data = await fetchData('https://api.example.com/data');
expect(data).toBeDefined();
});
test('should handle fetch errors gracefully', async () => {
await expect(fetchData('invalid-url')).rejects.toThrow('Failed to fetch data');
});
});
Enhancing Workflow with Cloving Proxy Server
For projects involving multiple asynchronous requests, debugging network calls can be complex. Launching a Cloving proxy server can help intercept these calls for closer inspection:
cloving proxy
Through the proxy, you can better understand how network requests are being made, adjusted, and catered to asynchronous operations.
Conclusion
Incorporating Cloving CLI into your Node.js development workflow empowers you to tackle asynchronous operations with confidence. With its AI-enhanced capabilities, you can streamline debugging, strengthen your code with comprehensive error handling, and automate the testing process. By harnessing Cloving CLI, you can significantly enhance both efficiency and code quality within your asynchronous function handling.
Remember, the goal is to augment your skills with AI-driven tools like Cloving, enabling you to focus more on crafting innovative solutions without being bogged down by debugging challenges.
Visit the Cloving CLI documentation for more tips and advanced usage: Cloving Documentation.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.