GPT-Generated Unit Tests for Rails Applications
Updated on June 26, 2024

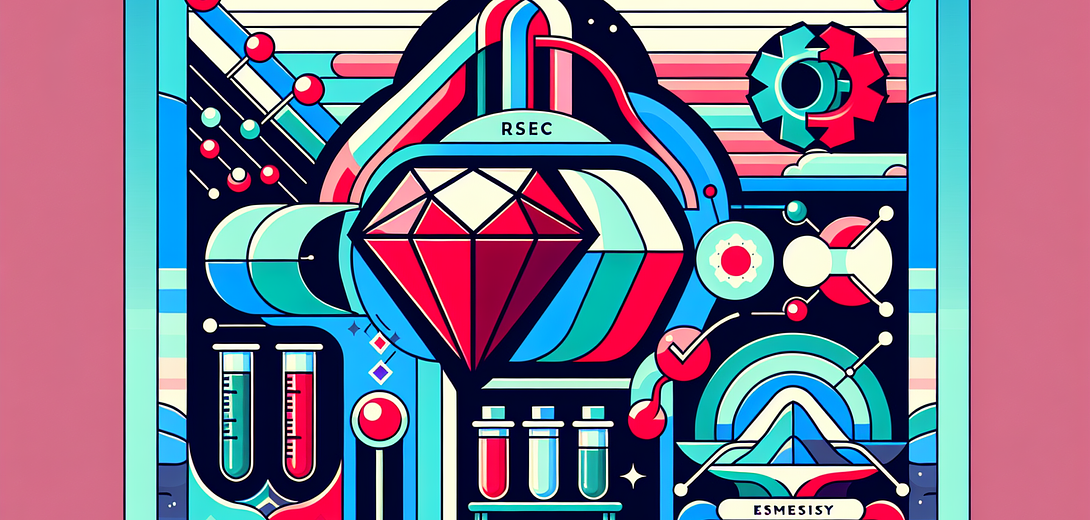
Unit testing is a critical part of modern software development, ensuring that individual components of an application work as intended. For Ruby on Rails developers, writing unit tests can be time-consuming and often repetitive. This is where GPT (Generative Pre-trained Transformer) can step in, providing a powerful tool to automate and streamline the process. In this blog post, we’ll explore how GPT can assist in generating unit tests for Rails applications, improving productivity and code quality.
Understanding GPT and Its Capabilities
GPT, developed by OpenAI, is an advanced language model capable of understanding and generating human-like text. Its applications are vast, ranging from content creation to code generation. By leveraging GPT, developers can automate various coding tasks, including the generation of unit tests.
Why Generate Unit Tests with GPT?
- Efficiency: Automating the creation of unit tests saves significant time, allowing developers to focus on more complex and critical aspects of the application.
- Consistency: GPT ensures that unit tests follow a consistent structure and style, reducing the likelihood of errors.
- Coverage: Automated test generation can help achieve higher test coverage, identifying edge cases that might be overlooked by manual testing.
Setting Up GPT for Unit Test Generation
Before diving into the specifics, you’ll need to set up a GPT environment. OpenAI provides APIs that you can integrate into your development workflow. Here’s the updated basic setup:
- Sign Up for OpenAI API: Obtain an API key from OpenAI by signing up for their service.
- Install OpenAI SDK: Use the following command to install the OpenAI SDK in your Rails project:
gem install openai
- Configure API Key: Set up your API key in your application, typically in an initializer file:
# config/initializers/openai.rb OpenAI.api_key = ENV['OPENAI_API_KEY']
Generating Unit Tests for Rails Models
Let’s start with generating unit tests for a Rails model. Consider a simple Post
model with title and body attributes:
class Post < ApplicationRecord
validates :title, presence: true
validates :body, presence: true
end
To generate unit tests for this model using GPT, you can write a script that sends the model definition to the GPT API and retrieves the test code. Here’s an updated example using the latest OpenAI gem:
require 'openai'
class TestGenerator
def initialize(model_code)
@model_code = model_code
end
def generate_tests
client = OpenAI::Client.new
response = client.chat(
parameters: {
model: "gpt-4o",
messages: [{ role: "user", content: "Generate RSpec unit tests for the following Rails model:\n#{@model_code}\n" }],
temperature: 0.7,
})
response.dig("choices", 0, "message", "content").strip
end
end
model_code = <<~MODEL
class Post < ApplicationRecord
validates :title, presence: true
validates :body, presence: true
end
MODEL
generator = TestGenerator.new(model_code)
puts generator.generate_tests
Sample Output
Running the script above might generate the following RSpec tests:
require 'rails_helper'
RSpec.describe Post, type: :model do
it 'is valid with valid attributes' do
post = Post.new(title: 'Sample Title', body: 'Sample body text')
expect(post).to be_valid
end
it 'is not valid without a title' do
post = Post.new(body: 'Sample body text')
expect(post).to_not be_valid
end
it 'is not valid without a body' do
post = Post.new(title: 'Sample Title')
expect(post).to_not be_valid
end
end
Generating Tests for Rails Controllers
In addition to models, you can use GPT to generate unit tests for Rails controllers. Here’s an example for a PostsController
:
class PostsController < ApplicationController
def index
@posts = Post.all
end
def show
@post = Post.find(params[:id])
end
def create
@post = Post.new(post_params)
if @post.save
redirect_to @post
else
render :new
end
end
private
def post_params
params.require(:post).permit(:title, :body)
end
end
To generate tests for this controller, you can extend the TestGenerator
class to handle controller code as well:
class ControllerTestGenerator
def initialize(controller_code)
@controller_code = controller_code
end
def generate_tests
client = OpenAI::Client.new
response = client.chat(
parameters: {
model: "gpt-4o",
messages: [{ role: "user", content: "Generate RSpec controller tests for the following Rails controller:\n#{@controller_code}\n" }],
temperature: 0.7,
})
response.dig("choices", 0, "message", "content").strip
end
end
controller_code = <<~CONTROLLER
class PostsController < ApplicationController
def index
@posts = Post.all
end
def show
@post = Post.find(params[:id])
end
def create
@post = Post.new(post_params)
if @post.save
redirect_to @post
else
render :new
end
end
private
def post_params
params.require(:post).permit(:title, :body)
end
end
CONTROLLER
generator = ControllerTestGenerator.new(controller_code)
puts generator.generate_tests
Sample Output
Running the script above might generate the following RSpec tests:
require 'rails_helper'
RSpec.describe PostsController, type: :controller do
describe 'GET #index' do
it 'returns a success response' do
get :index
expect(response).to be_successful
end
end
describe 'GET #show' do
it 'returns a success response' do
post = Post.create!(title: 'Sample Title', body: 'Sample body text')
get :show, params: { id: post.id }
expect(response).to be_successful
end
end
describe 'POST #create' do
context 'with valid params' do
it 'creates a new Post' do
expect {
post :create, params: { post: { title: 'Sample Title', body: 'Sample body text' } }
}.to change(Post, :count).by(1)
end
it 'redirects to the created post' do
post :create, params: { post: { title: 'Sample Title', body: 'Sample body text' } }
expect(response).to redirect_to(Post.last)
end
end
context 'with invalid params' do
it 'renders the new template' do
post :create, params: { post: { title: '', body: 'Sample body text' } }
expect(response).to render_template(:new)
end
end
end
end
Benefits and Considerations
Using GPT to generate unit tests for Rails applications offers several benefits:
- Speed: Quickly generate tests for various components, reducing the manual effort required.
- Consistency: Ensure that tests adhere to a standard format, making them easier to read and maintain.
- Learning Tool: For less experienced developers, generated tests can serve as examples to learn from.
However, it’s important to review and possibly tweak the generated tests to ensure they accurately reflect your application’s requirements and edge cases. While GPT is powerful, it may not fully understand the intricacies of your specific application context.
Conclusion
GPT offers a promising way to automate the generation of unit tests for Rails applications, saving developers time and effort while promoting better test coverage and consistency. By integrating GPT into your development workflow, you can focus more on building features and solving complex problems, leaving the repetitive task of writing unit tests to the AI.
If you haven’t tried it yet, consider integrating GPT into your Rails development process and experience the benefits of automated test generation. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.