GPT-Driven Automation for Python Web Scraping Applications
Updated on December 25, 2024

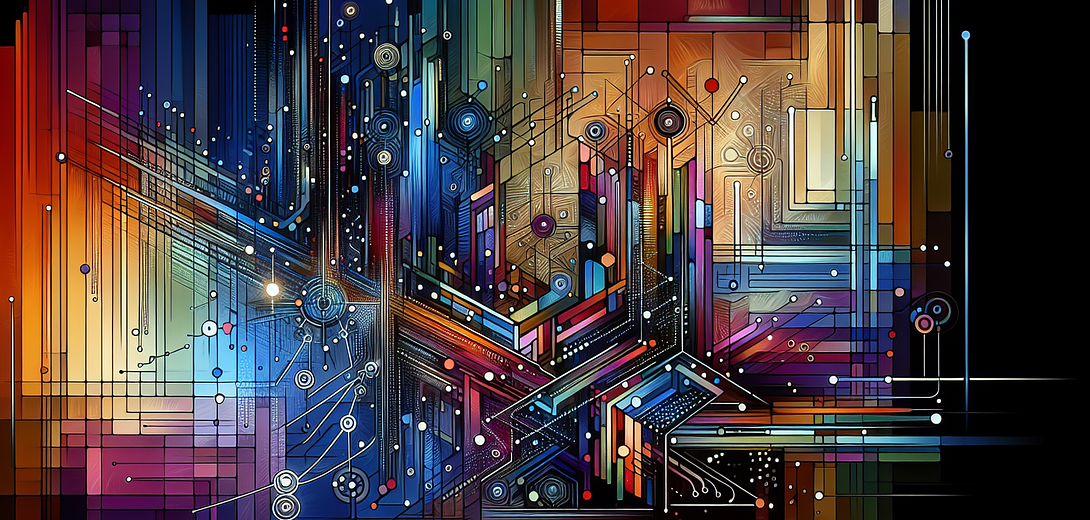
Web scraping is a powerful tool for gathering data from the internet, often used in market research, data analysis, and competitive monitoring. With the rise of AI-powered tools, developers now have advanced options to enhance their web scraping processes. The Cloving CLI tool brings the power of AI into your workflow, allowing you to automate and optimize web scraping applications efficiently. This blog post will guide you through leveraging the Cloving CLI for automating Python web scraping tasks.
1. Getting Started with Cloving CLI
Before diving into automation, we need to set up Cloving in your environment.
Installation:
Ensure you have Cloving installed globally using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving with your preferred AI model and API key:
cloving config
This interactive setup will guide you in configuring the API key, selecting a model, and setting initial preferences.
2. Initializing Your Web Scraping Project
To make the most of Cloving’s capabilities, initialize it in your project directory:
cloving init
This command creates a cloving.json
file in your project, encompassing metadata and context for your scraping application.
3. Automating Code Generation for Web Scraping
One of the most significant advantages of using Cloving is the seamless code generation it offers. Suppose you aim to scrape data from a website using BeautifulSoup and requests in Python.
Example:
To generate a Python script for scraping a webpage:
cloving generate code --prompt "Generate a Python script to scrape data from a webpage using BeautifulSoup and requests"
Cloving analyzes your requirements and generates a relevant code snippet:
import requests
from bs4 import BeautifulSoup
def scrape_webpage(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
data = [element.text for element in soup.find_all('p')]
return data
else:
return f"Error: {response.status_code}"
url = "http://example.com"
data = scrape_webpage(url)
print(data)
This script initializes a web request and fetches all paragraph text (<p>
tags) from a target page using BeautifulSoup.
4. Generating Unit Tests for Web Scraping
After creating your scraping script, it’s essential to ensure its reliability by implementing unit tests. Cloving simplifies this process:
cloving generate unit-tests -f src/scraper.py
This command generates a test file with appropriate test cases for your web scraper:
import unittest
from scraper import scrape_webpage
class TestScrapeWebpage(unittest.TestCase):
def test_successful_scrape(self):
data = scrape_webpage("http://example.com")
self.assertIsInstance(data, list)
def test_invalid_url(self):
data = scrape_webpage("invalid_url")
self.assertIn('Error', data)
if __name__ == '__main__':
unittest.main()
5. Enhancing Scripts with Cloving Chat
For more intricate requirements or assistance, use Cloving’s interactive chat to fine-tune or expand your scripts.
cloving chat -f src/scraper.py
This session allows ongoing interaction with the AI, where you can request code improvements, explanations, or additional features for your script.
6. Improving Automation with Script Generation
Cloving supports creating shell scripts to automate repetitive tasks. For instance, automating the execution of the scraper script periodically:
cloving generate shell --prompt "Create a shell script to run the scraper.py script every day at midnight"
Cloving can generate a cron job script for task automation:
echo "0 0 * * * /usr/bin/python3 /path/to/scraper.py" | crontab -
This command schedules the script to run daily at midnight using cron.
7. Automated Code Reviews for Best Practices
Leverage Cloving’s AI-powered code review feature to identify improvements or issues in your script:
cloving generate review
A comprehensive review output will guide you through best practices, optimizations, and potential refactors for better performance and maintainability.
Conclusion
The integration of GPT-based automation using Cloving CLI revolutionizes how we approach web scraping applications in Python. By developing and refining scripts with AI-enhanced tools, you can significantly streamline your data collection processes, boost productivity, and ensure robust code quality. Consider Cloving as your AI-support system, turning complex web scraping tasks into manageable, efficient workflows. Explore, experiment, and embrace the potential of AI to elevate your development capabilities.
By following these steps and utilizing Cloving’s features, you can achieve efficient and effective automation for your Python web scraping projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.