Generating Vue.js Components with GPT
Updated on June 26, 2024

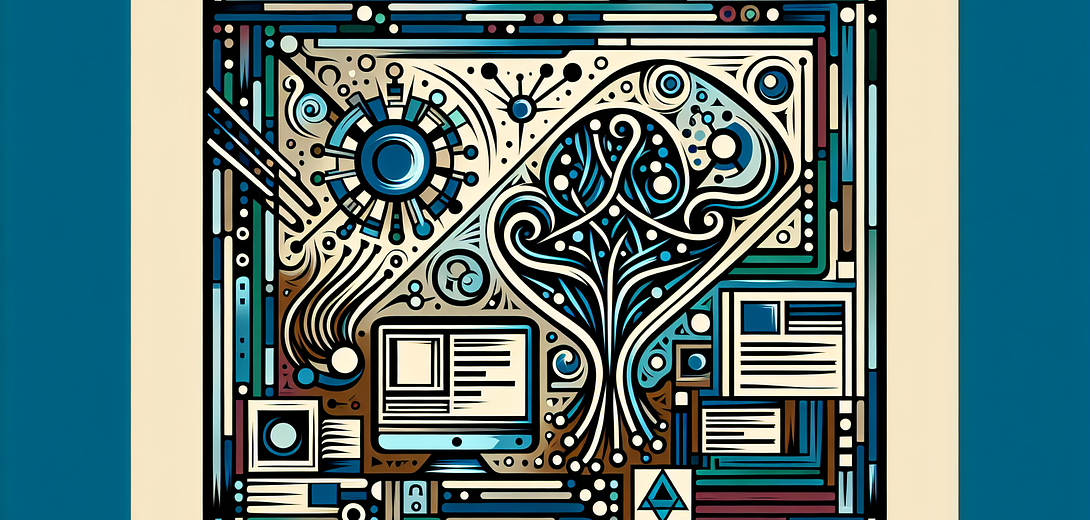
The landscape of web development is ever-evolving, and staying at the forefront often requires harnessing the latest tools and methodologies. Cloving—harmoniously melding human creativity with the computational might of artificial intelligence—presents a revolutionary approach. In this post, we delve into how you can leverage GPT (Generative Pre-trained Transformer) to generate and enhance Vue.js components, making your development process more efficient and effective.
Understanding Cloving
Cloving is the practice of combining human intuition and creativity with the computational power of AI to achieve shared objectives. This isn’t just about using AI as a tool; it’s about creating a collaborative environment where both human and machine flourish together.
1. Rapid Component Generation
Generating Vue.js components can often become repetitive. With GPT, you can streamline this process, creating boilerplate code quickly and customizing it to your needs.
Example:
Let’s say you need to create a new Vue.js component for a user profile card. You can prompt GPT:
Generate a Vue.js component that displays a user profile, including the user's name, avatar, and bio.
GPT will provide you with a starting point for your component:
<template>
<div class="user-profile">
<img :src="avatar" alt="User Avatar" />
<h2>{{ name }}</h2>
<p>{{ bio }}</p>
</div>
</template>
<script>
export default {
name: 'UserProfile',
props: {
name: String,
avatar: String,
bio: String,
},
};
</script>
<style scoped>
.user-profile {
border: 1px solid #ddd;
padding: 16px;
border-radius: 8px;
text-align: center;
}
.user-profile img {
border-radius: 50%;
width: 100px;
height: 100px;
}
</style>
This provides a functional foundation that you can then refine and expand upon.
2. Code Optimization and Best Practices
Keeping up with best practices can be daunting. GPT can offer insights into the latest conventions and help optimize your code.
Example:
If you’re unsure whether your current method of handling props in a Vue.js component meets best practices, you can ask GPT:
How can I improve the handling of props in this Vue.js component to follow best practices?
GPT might respond with advice on type checking, using default values, or documenting prop usage with JSDoc comments.
3. Automated Testing
Testing is a crucial part of development, but writing tests can be laborious. GPT can automate the creation of unit tests, ensuring your components are robust and reliable.
Example:
Suppose you’ve written a Vue.js component and need to create unit tests. You can prompt GPT:
Generate unit tests for this Vue.js component: [UserProfile component code].
GPT will generate relevant unit tests using a framework like Jest:
import { shallowMount } from '@vue/test-utils';
import UserProfile from '@/components/UserProfile.vue';
describe('UserProfile.vue', () => {
it('renders props when passed', () => {
const name = 'John Doe';
const avatar = 'http://example.com/johndoe.jpg';
const bio = 'Frontend Developer';
const wrapper = shallowMount(UserProfile, {
propsData: { name, avatar, bio },
});
expect(wrapper.text()).toMatch(name);
expect(wrapper.text()).toMatch(bio);
expect(wrapper.find('img').attributes('src')).toBe(avatar);
});
});
4. Enhanced Documentation
Maintaining clear and concise documentation can be challenging, especially for complex components. GPT can assist in generating and refining documentation.
Example:
When you need to document a Vue.js component, you can ask GPT:
Generate documentation for this Vue.js component: [UserProfile component code].
GPT might produce something like this:
### UserProfile Component
#### Props:
- `name` (String): The name of the user.
- `avatar` (String): URL of the user's avatar image.
- `bio` (String): A short biography of the user.
#### Example Usage:
```vue
<template>
<UserProfile
name="John Doe"
avatar="http://example.com/johndoe.jpg"
bio="Frontend Developer"
/>
</template>
5. Refactoring Assistance
Refactoring can improve code maintainability but is often complex. GPT can provide guidance and suggestions for refactoring your Vue.js components.
Example:
If you have a component with duplicated logic and want to refactor, you can ask GPT:
How can I refactor this Vue.js component to remove duplicated logic? [provide component code]
GPT will analyze and suggest improvements, potentially abstracting common functionality into reusable methods or components.
Conclusion
Harnessing the principles of cloving, integrating GPT into your Vue.js development workflow can elevate your efficiency, improve code quality, and keep you abreast of best practices. Embrace this symbiotic relationship and discover how this innovative approach can transform your development process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.