Generating Unit Tests in Ruby on Rails with GPT
Updated on June 26, 2024

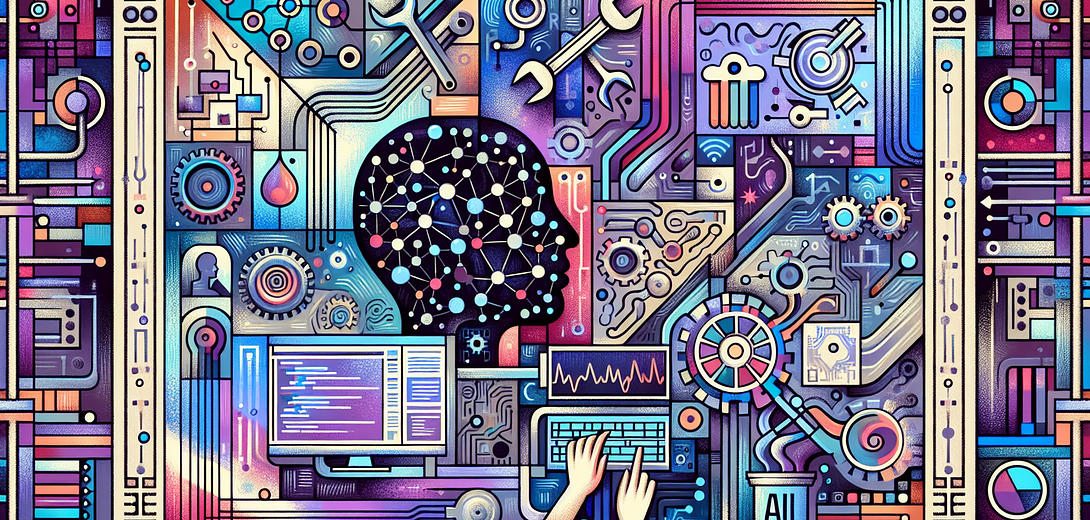
In the evolving landscape of software development, efficient testing is critical to ensuring your application works as intended.
By embracing cloving—the integration of human creativity and intuition with artificial intelligence (AI) processing capabilities—you can supercharge your unit testing workflow in Ruby on Rails.
This post will guide you through practical techniques to incorporate GPT, a powerful AI text generation tool, into your daily programming tasks, making you more efficient and effective.
Understanding Cloving
Cloving is more than just using AI tools; it’s about creating a synergy where the analytical strengths of AI complement the human touch in decision-making and creativity. This approach can help streamline various aspects of unit testing in Ruby on Rails.
1. Generating Basic Test Cases
One of the most time-consuming aspects of unit testing is writing out the initial test cases. GPT can assist by generating skeletal test cases based on your model code, leaving you to fine-tune and expand them.
Example:
Suppose you have a User
model in your Rails application. You can prompt GPT to generate a basic unit test for it:
Generate RSpec tests for the following Ruby on Rails User model:
class User < ApplicationRecord
validates :email, presence: true, uniqueness: true
validates :password, presence: true, length: { minimum: 6 }
end
GPT would provide you with a basic test scaffold:
# spec/models/user_spec.rb
require 'rails_helper'
RSpec.describe User, type: :model do
it "is valid with valid attributes" do
user = User.new(email: "[email protected]", password: "password")
expect(user).to be_valid
end
it "is not valid without an email" do
user = User.new(email: nil, password: "password")
expect(user).not_to be_valid
end
it "is not valid without a password" do
user = User.new(email: "[email protected]", password: nil)
expect(user).not_to be_valid
end
it "is not valid with a duplicate email" do
User.create(email: "[email protected]", password: "password")
user = User.new(email: "[email protected]", password: "password123")
expect(user).not_to be_valid
end
it "is not valid with a password less than 6 characters" do
user = User.new(email: "[email protected]", password: "short")
expect(user).not_to be_valid
end
end
2. Enhancing Test Coverage
GPT can help you identify edge cases or additional scenarios you may have missed. This ensures broader test coverage and higher code reliability.
Example:
For the User
model above, you can ask GPT to suggest additional test cases:
Suggest additional RSpec tests for edge cases in the User model, which includes email and password validations.
GPT might suggest:
# spec/models/user_spec.rb
# Additional edge cases
RSpec.describe User, type: :model do
# previous test cases...
it "is not valid with an improperly formatted email" do
user = User.new(email: "invalid-email", password: "password")
expect(user).not_to be_valid
end
it "is valid with an email of varying case but unique" do
User.create(email: "[email protected]", password: "password")
user = User.new(email: "[email protected]", password: "password")
expect(user).to be_valid
end
end
3. Speeding Up Test-Driven Development (TDD)
GPT supports the TDD approach by quickly generating tests before writing code, ensuring your development stays focused on meeting specifications from the start.
Example:
When adding a new feature, you can prompt GPT:
Generate RSpec tests for a new feature that sends a welcome email to the user after creation.
GPT will generate skeleton tests:
# spec/models/user_spec.rb
RSpec.describe User, type: :model do
describe "callbacks" do
it "sends a welcome email after creation" do
user = User.create(email: "[email protected]", password: "password")
expect(UserMailer).to have_received(:welcome_email).with(user) # Assuming a mailer stub is configured
end
end
end
4. Automating Documentation
Understanding and maintaining a comprehensive test suite can be daunting. GPT can auto-generate documentation for your tests, making it easier to onboard new team members or revisit the tests later.
Example:
You can ask GPT to document the generated tests:
Generate documentation for the RSpec tests provided for the User model.
GPT might produce:
# Documentation for User Model Tests
This test suite ensures the User model validations and callbacks are functioning correctly.
## Tests Included:
1. Validity with valid attributes
2. Invalidity without email
3. Invalidity without password
4. Invalidity with duplicate email
5. Invalidity with short password
6. Invalidity with improperly formatted email
7. Validity with email of varying case (for uniqueness)
8. Sends a welcome email after creation
Each test case aims to cover essential scenarios and edge cases, enhancing the reliability and robustness of the User model.
Conclusion
Embracing cloving by integrating GPT into your unit testing workflow in Ruby on Rails can significantly enhance your productivity and code quality. By allowing GPT to handle repetitive and initial tasks, you can focus on more complex and creative aspects of development. This harmonious collaboration between human and AI exemplifies the future of software engineering, making your job as a programmer more efficient and enjoyable.
So, next time you embark on creating unit tests in your Rails application, remember to leverage the power of GPT and experience the transformative potential of cloving in your development process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.