Generating Robust Unit Tests for Java Applications with GPT Support
Updated on March 05, 2025

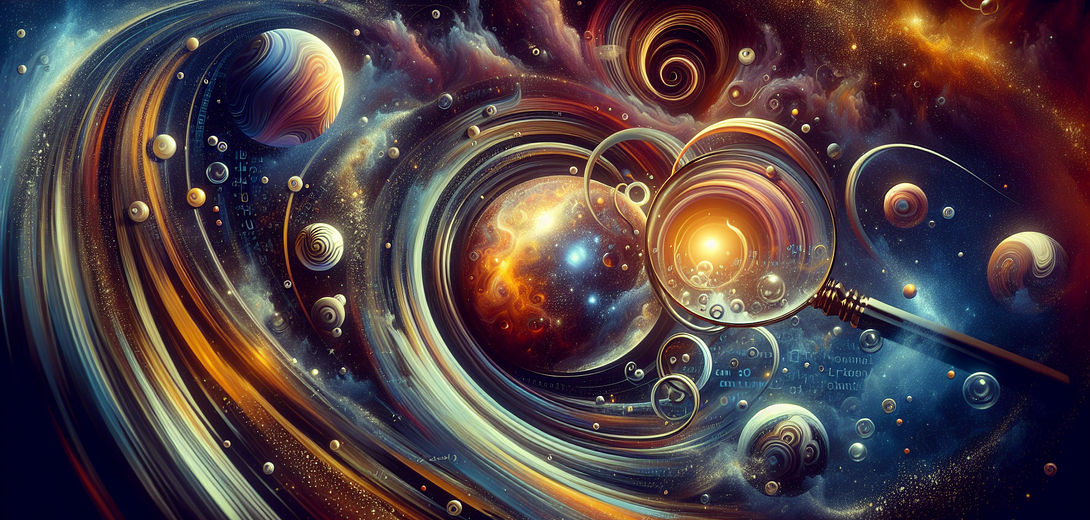
Streamlining Java Unit Test Generation with Cloving CLI
As a Java developer, ensuring code reliability and functionality through testing is a crucial aspect of your workflow. The Cloving CLI tool empowers developers to seamlessly integrate AI into the process of generating robust unit tests, reducing potential errors and improving code quality. In this blog post, we’ll explore how to effectively use Cloving CLI to generate unit tests for Java applications and enhance your testing process with AI assistance.
1. Setting Up Cloving for Java Projects
Before diving into test generation, it’s essential to have Cloving correctly set up with your Java project.
Installation:
First, ensure Cloving is installed globally via npm:
npm install -g cloving@latest
Configuration:
Configure your Cloving setup to leverage the AI model for test generation:
cloving config
Follow the interactive setup to input your API key and choose your preferred AI model.
2. Initializing Your Java Project with Cloving
Initialize Cloving in your Java project directory to acclimate the tool to your project’s environment:
cloving init
This command will analyze your project, creating a cloving.json
file that compiles metadata and default configurations for your application.
3. Generating Unit Tests
With Cloving correctly set up, let’s focus on generating unit tests.
Example (Basic Arithmetic Class):
Suppose you have a Java class, Calculator.java
, designed to perform basic arithmetic operations. Generating unit tests for this class can be accomplished using:
cloving generate unit-tests -f src/main/java/com/example/Calculator.java
Cloving will analyze the Calculator
class and generate corresponding JUnit tests that validate its functions. Below is a sample output:
// src/test/java/com/example/CalculatorTest.java
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class CalculatorTest {
@Test
void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
@Test
void testSubtraction() {
Calculator calc = new Calculator();
assertEquals(1, calc.subtract(4, 3));
}
// Add additional tests for multiplication and division
}
4. Advanced Examples
Cloving isn’t limited to trivial use cases. Below are a few scenarios where Cloving can help with more complex testing needs.
4.1. Classes With External Dependencies
Consider a UserService.java
that depends on a UserRepository.java
interface for database operations. Cloving can generate skeleton tests that use mocking frameworks (like Mockito) to handle external dependencies.
cloving generate unit-tests -f src/main/java/com/example/UserService.java
Sample Output (With Mockito):
// src/test/java/com/example/UserServiceTest.java
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
class UserServiceTest {
@Mock
private UserRepository userRepository;
private UserService userService;
@BeforeEach
void setUp() {
MockitoAnnotations.openMocks(this);
userService = new UserService(userRepository);
}
@Test
void testFindUserById() {
when(userRepository.findById("123")).thenReturn(new User("123", "Alice"));
User user = userService.findUserById("123");
assertEquals("Alice", user.getName());
}
// Additional tests for user creation, updates, etc.
}
Cloving automatically infers potential mocking strategies based on the class structure and injected dependencies. You can then refine the generated tests via Cloving’s chat interface.
4.2. Spring Boot Controllers
If you have a Spring Boot controller, such as HelloController.java
, Cloving can generate test templates leveraging Spring’s testing utilities:
cloving generate unit-tests -f src/main/java/com/example/HelloController.java
Sample Output (With SpringBootTest):
// src/test/java/com/example/HelloControllerTest.java
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
@SpringBootTest
@AutoConfigureMockMvc
class HelloControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
void testHelloEndpoint() throws Exception {
mockMvc.perform(get("/hello"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World!"));
}
}
5. Refining and Optimizing Test Cases
Cloving doesn’t just generate tests; it allows you to refine them further. Once tests are generated, you can ask Cloving chat for enhancements or explanations to ensure your tests cover all edge cases.
Example:
Open Cloving chat to refine tests:
cloving chat -f src/test/java/com/example/CalculatorTest.java
You might interact with Cloving like this:
cloving> Could you add test cases to handle division by zero?
Certainly! I'll add a test case for division by zero:
Sample Updated Test Case:
@Test
void testDivisionByZero() {
Calculator calc = new Calculator();
Exception exception = assertThrows(ArithmeticException.class, () -> {
calc.divide(5, 0);
});
assertEquals("/ by zero", exception.getMessage());
}
6. Saving and Integrating Unit Tests
After verifying and modifying the generated tests to your liking, seamlessly save them using Cloving commands. If you use the --save
option while generating, the tests will be automatically saved:
cloving generate unit-tests -f src/main/java/com/example/Calculator.java --save
Additionally, use the review
command to get AI-powered insights into how your tests could be improved further:
cloving generate review
This generates a comprehensive review of your test strategy, potentially highlighting overlooked scenarios or suggesting optimizations.
7. Using Cloving in CI/CD Pipelines
For teams that rely heavily on Continuous Integration/Continuous Deployment (CI/CD), Cloving can be integrated into your pipeline for automated test generation or review:
name: Java CI with Cloving
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Java
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Install Cloving
run: npm install -g cloving@latest
- name: Generate Unit Tests
run: cloving generate unit-tests -f src/main/java/com/example/*.java --save
- name: Run Tests
run: ./gradlew test
By automating Cloving commands, you ensure new code changes are promptly supplied with relevant tests or test reviews.
8. Best Practices for Using Cloving in Unit Test Generation
- Contextual Setup: Always initialize your Java project with
cloving init
to provide optimal context for code and test generation. - Leverage Interactive Features: Use the Cloving chat for comprehensive explanations or further test enhancements.
- Utilize AI Insights: Regularly employ the
generate review
feature to ensure your test cases are thorough. - Mocking and Stubbing: When dealing with external services or databases, let Cloving guide you in adding Mockito (or other frameworks) to handle dependencies cleanly.
- Regular Updates: Keep Cloving updated to utilize the latest AI capabilities in test generation.
- Combine With Code Coverage Tools: Integrate code coverage tools like JaCoCo to highlight untested parts of your code, then ask Cloving to generate or suggest additional tests for those specific areas.
Conclusion
By incorporating Cloving CLI into your Java development cycle, you can remarkably streamline the process of generating robust unit tests—even for classes with external dependencies, Spring Boot controllers, or advanced mocking scenarios. This not only enhances productivity but also significantly elevates the reliability and quality of your codebase. Embrace the power of Cloving CLI to transform your development workflow with AI-enhanced capabilities.
As with any tool, Cloving is designed to assist rather than replace your expertise. Utilize it to augment your skills, ensuring your Java applications are top-notch. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.