Generating Robust GraphQL APIs in Node.js Using AI Technology
Updated on March 07, 2025

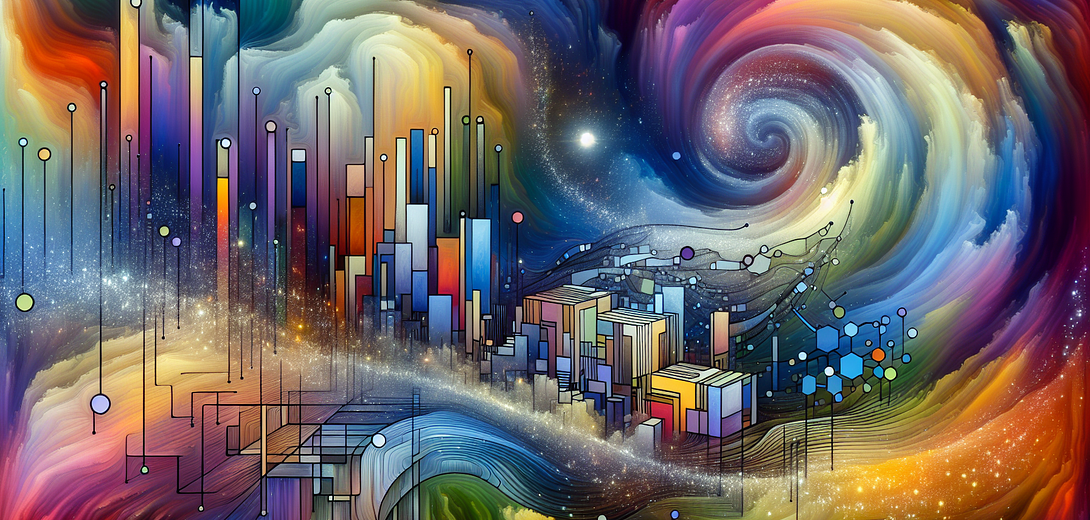
Supercharging GraphQL API Development with Cloving CLI
GraphQL has revolutionized how developers structure and query data. While it offers great flexibility and efficiency, building high-quality GraphQL schemas and resolvers can still be time-consuming – especially for large or complex applications. Cloving CLI, an AI-powered command-line tool, steps in to automate common tasks, from generating GraphQL schemas and resolvers to providing AI-driven code reviews and test coverage. In this post, we explore how to use Cloving CLI to build robust GraphQL APIs in Node.js.
1. Introduction to Cloving CLI
Cloving CLI integrates AI (e.g., GPT-based models) into your development workflow to:
- Generate code (schemas, resolvers, boilerplate, etc.)
- Review existing code for improvements
- Iterate via a chat-based environment
- Automate commits and other tasks
By providing your project’s context, Cloving can produce relevant, efficient code that aligns with your style and architecture.
2. Getting Started with Cloving CLI
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
After installing, configure Cloving with your API key and AI model:
cloving config
Follow the prompts to finalize your credentials. Once set up, Cloving can generate code and run advanced tasks for your GraphQL projects.
3. Initializing Your Node.js Project
To allow Cloving to tailor outputs to your codebase, navigate to your Node.js project root and run:
cloving init
Cloving analyzes the folder structure, packages, and any existing GraphQL files, creating a cloving.json
with metadata. This context ensures the AI can produce code that fits your project’s structure.
4. Generating GraphQL Schema and Resolvers
4.1 Example: Bookstore GraphQL
Suppose you’re building a GraphQL API for a bookstore. You need a schema to handle typical book operations. Prompt Cloving:
cloving generate code --prompt "Generate GraphQL schema and resolvers for managing books in a bookstore" --files src/schema/index.js
Sample Output:
// src/schema/index.js
const { gql } = require('apollo-server-express');
// Define GraphQL schema
const typeDefs = gql`
type Book {
id: ID!
title: String!
author: String!
publishedYear: Int
}
type Query {
books: [Book!]!
book(id: ID!): Book
}
type Mutation {
addBook(title: String!, author: String!, publishedYear: Int): Book
removeBook(id: ID!): Boolean
}
`;
const resolvers = {
Query: {
books: async () => {
// Fetch all books
},
book: async (parent, args) => {
// Fetch a single book by ID
},
},
Mutation: {
addBook: async (parent, args) => {
// Logic to add a book
},
removeBook: async (parent, args) => {
// Logic to remove a book
},
},
};
module.exports = { typeDefs, resolvers };
Notes:
- Cloving sets up basic GraphQL type definitions (typeDefs).
- You can refine the resolvers (e.g. hooking up to a database) or add custom logic.
4.2 Refining Schema and Resolvers
Should you want additional fields or methods (like a updateBook
mutation), you can instruct Cloving to refine the code. For instance:
Revise the schema to include a mutation for updating book details
Cloving updates the typeDefs
to include an updateBook
mutation and modifies the resolvers
accordingly.
5. Interacting with Cloving Chat for Complex Logic
5.1 Example: Advanced Filtering
If you require advanced logic (e.g., filtering books by author, date range, or partial text search), open a chat session:
cloving chat -f src/schema/index.js
In the interactive session, you might say:
cloving> Add a query to filter books by a range of publishedYear
Cloving can respond with an updated query definition and a matching resolver (like booksByYearRange
). You can refine it further or add performance hints.
6. AI-Powered Code Reviews
Maintaining code quality is crucial for a stable API. Let Cloving review your code:
cloving generate review
Cloving inspects your staged changes, providing a summary of potential improvements or best practices (e.g., error handling, input validation, or performance considerations).
7. Generating Tests for Your GraphQL API
7.1 Test Generation
A robust GraphQL API includes tests verifying queries, mutations, and error scenarios. Cloving can generate these tests:
cloving generate unit-tests -f src/schema/index.js
Sample Output:
// src/schema/tests/index.test.js
const { graphql } = require('graphql');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const { typeDefs, resolvers } = require('../index');
const schema = makeExecutableSchema({ typeDefs, resolvers });
describe('GraphQL API', () => {
it('fetches all books', async () => {
const query = `{ books { id title author publishedYear } }`;
const result = await graphql({ schema, source: query });
expect(result.errors).toBeUndefined();
expect(result.data.books).toBeDefined();
});
it('adds a new book', async () => {
const mutation = `
mutation {
addBook(title: "Test Book", author: "Test Author", publishedYear: 2021) {
id
title
author
}
}
`;
const result = await graphql({ schema, source: mutation });
expect(result.errors).toBeUndefined();
expect(result.data.addBook.title).toBe("Test Book");
});
});
Notes:
- Adjust or extend the tests for negative scenarios (e.g., missing fields, invalid ID).
- Integrate the tests with your CI pipeline (e.g., run
npm test
).
8. Streamlining Commits
Use Cloving to generate commit messages describing the changes:
cloving commit
Cloving inspects staged files (like new resolvers, schema expansions, or test additions) and suggests a commit message, e.g.:
Add GraphQL schema and resolvers for bookstore with test coverage
You can accept or refine as needed.
9. Best Practices for Node.js + GraphQL + Cloving
- Initialize Early
Runcloving init
so the AI recognizes your Node.js structure (e.g., package.json, .env, or existing code). - Be Explicit
Provide enough detail in your prompts (e.g., “Include date filtering for books in Query type”). - Iterate
Start with a base schema. Use the chat to refine or expand step by step (e.g., add sorting, pagination). - Leverage Tools
Combine Cloving with popular GraphQL libraries (Apollo Server, GraphQL Yoga) or schema-first approaches. Cloving can generate the boilerplate, letting you focus on business logic. - Error Handling & Validation
Expand or refine resolvers to handle invalid inputs. Cloving can also produce custom error messages or validations if prompted.
10. Example Workflow
Below is a typical approach to building a Node.js GraphQL API with Cloving:
- Initialize:
cloving init
in your project root. - Generate: e.g.,
cloving generate code --prompt "Create a GraphQL schema for an e-commerce app" -f src/schema/index.js
. - Test:
cloving generate unit-tests -f src/schema/index.js
. - Refine:
cloving chat -f src/schema/index.js
for advanced logic or performance improvements. - Review:
cloving generate review
to catch potential design or security issues. - Commit:
cloving commit
for an AI-generated summary of changes.
11. Conclusion
Cloving CLI provides an AI-augmented approach to building GraphQL APIs in Node.js, covering everything from schema creation and resolver logic to test generation and code reviews. By offloading repetitive tasks to an AI assistant, you can devote more energy to designing robust, user-centric features and refining your backend architecture.
Remember: Cloving’s code generation and suggestions are a starting point. Always review the AI-generated output, ensuring it aligns with your domain logic, performance constraints, and best practices. As you adopt Cloving, you’ll discover how AI-driven development can accelerate your GraphQL workflow, making it more efficient and enjoyable.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.