Generating Efficient Data Models for Ruby on Rails Using GPT
Updated on July 01, 2024

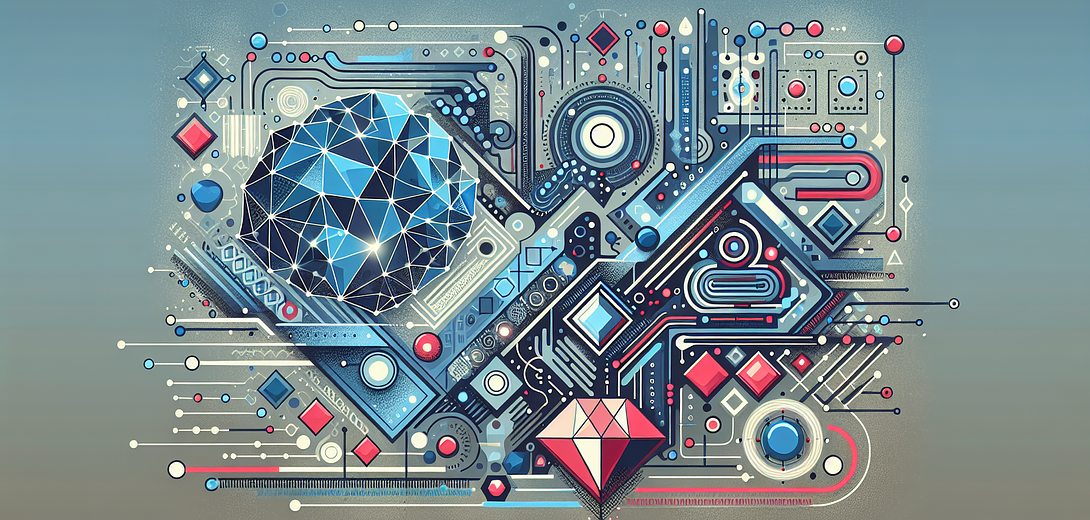
In the dynamic world of software development, the integration of human creativity with artificial intelligence (AI) is revolutionizing workflows. This innovative approach, known as cloving, harnesses the processing power of AI to complement human intuition and creativity, making tasks more efficient and precise.
In this blog post, we’ll explore how programmers can effectively incorporate AI, specifically GPT, into their daily workflows to generate efficient data models for Ruby on Rails applications. By using GPT, you can streamline your development process, ensuring robust, scalable, and maintainable models.
Understanding Cloving
Cloving is the practice of blending human ingenuity with AI capabilities. It’s not merely about employing AI tools but cultivating a symbiotic relationship where both human and machine strengths are optimized to achieve better outcomes faster.
1. Generating Rails Scaffolding: Models, Controllers, and Views
Creating scaffolding that accurately represents your application’s domain is pivotal. GPT can assist by generating well-structured scaffolding based on your requirements.
Example:
Suppose you need to create scaffolding for a blogging application with various relationships. You can provide GPT with a prompt:
Generate the Rails generate command for scaffolding a Blog that includes attributes for title (string), content (text), published_at (datetime), and belongs to a User.
GPT’s response:
rails generate scaffold Blog title:string content:text published_at:datetime user:references
2. Generating Model Structures with Custom Logic
Even after creating scaffolding, you might need to add specific business logic to your models. GPT can help by generating custom model structures.
Example:
For a Blog model with validation and custom methods, you can prompt GPT:
Create a Ruby on Rails model for a Blog that includes attributes for title, content, published_at, and belongs to a User, with validation and a method to publish the blog.
GPT’s response:
class Blog < ApplicationRecord
belongs_to :user
validates :title, presence: true
validates :content, presence: true
scope :published, -> { where.not(published_at: nil) }
def publish
update(published_at: Time.current)
end
end
3. Optimizing Database Migrations
GPT can assist in optimizing your database migrations, ensuring your database schema aligns perfectly with your models and application requirements.
Example:
After generating the initial scaffolding, you might need to ask GPT about additional migrations for performance enhancements such as adding indexes to frequently queried fields. Provide GPT with a prompt:
Are there any additional migrations I should consider for performance benefits or indexing for the Blog model?
GPT’s response:
class AddIndexesToBlogs < ActiveRecord::Migration[6.0]
def change
add_index :blogs, :published_at
add_index :blogs, :user_id
end
end
Adding these indexes improves query performance, especially on columns that are often used in search conditions or as foreign keys.
4. Enhancing Validations and Callbacks
Validations and callbacks are crucial for ensuring data integrity and implementing business logic. GPT can assist in suggesting best practices and code snippets.
Example:
For adding a uniqueness validation to the blog title, you could prompt GPT:
Add a uniqueness validation for the title attribute in the Blog model.
GPT’s response:
class Blog < ApplicationRecord
belongs_to :user
validates :title, presence: true, uniqueness: true
validates :content, presence: true
scope :published, -> { where.not(published_at: nil) }
def publish
update(published_at: Time.current)
end
end
5. Creating Efficient Associations
Associations between models define how they interact. Proper use of associations is critical for data consistency and query efficiency. GPT can help you set up these associations correctly.
Example:
To create a one-to-many association between User and Blog models, provide GPT with a prompt:
Define a one-to-many association between User and Blog models in Ruby on Rails.
GPT’s response:
class User < ApplicationRecord
has_many :blogs, dependent: :destroy
end
class Blog < ApplicationRecord
belongs_to :user
validates :title, presence: true, uniqueness: true
validates :content, presence: true
scope :published, -> { where.not(published_at: nil) }
def publish
update(published_at: Time.current)
end
end
6. Generating Factories for Testing
Testing is an essential part of development. GPT can help you generate factories, which are vital for setting up test data.
Example:
To generate a factory for the Blog model using FactoryBot, prompt GPT:
Generate a FactoryBot factory for the Blog model.
GPT’s response:
FactoryBot.define do
factory :blog do
sequence(:title) { |n| "Blog Post #{n}" }
content { "This is the content of the blog post." }
published_at { nil }
association :user
trait :published do
published_at { Time.current }
end
end
end
Conclusion
Integrating GPT into your Ruby on Rails development workflow exemplifies the power of cloving, where AI complements human skills to create efficient and maintainable data models. By leveraging GPT’s capabilities to generate scaffolding, model structures, migrations, validations, associations, and test factories, programmers can enhance productivity, reduce errors, and adhere to best practices seamlessly. Embrace cloving and discover how this synergy can transform your programming experience, leading to robust and scalable applications.
Bonus Follow-Up Prompts
Here are a few additional prompts you can use to refine and extend the capabilities discussed above:
How can I configure GitHub to run these tests automatically for me?
Generate accompanying factories for example data.
What are other GPT prompts I could use to make this more efficient?
Note:
Whenever you see: [code snippet], replace it with an appropriate snippet in the language in question.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.