Generating Blazing-Fast Go Microservices with the Aid of GPT
Updated on March 07, 2025

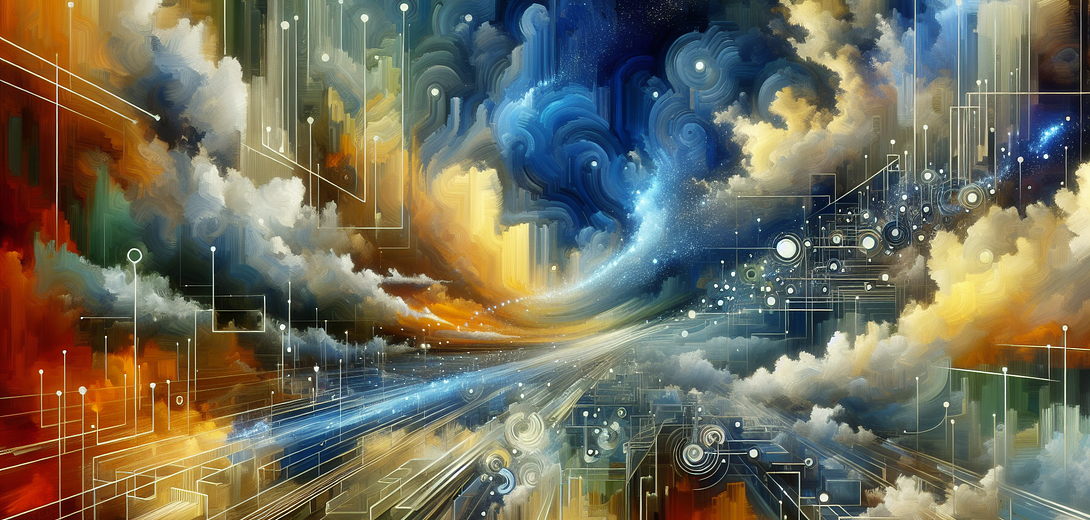
Building High-Performance Go Microservices with Cloving CLI
Go (Golang) has become a premier choice for microservice architectures, thanks to its concurrency model and robust performance. Even with Go’s strengths, there’s plenty of routine scaffolding and boilerplate involved in setting up microservices. Cloving CLI, powered by GPT-based models, integrates AI capabilities into your development workflow, helping you quickly generate, test, and optimize your Go code. This guide shows you how to enhance your microservice development by tapping into Cloving’s AI-driven features.
1. Understanding Cloving CLI
Cloving CLI is an AI-assisted command-line interface that helps developers by:
- Generating code in various languages, including Go
- Creating unit tests
- Reviewing code for best practices
- Refining code via an interactive chat environment
- Facilitating code commits with AI-suggested messages
By providing Cloving with your project context, you can produce or modify Go microservice code seamlessly.
2. Setting Up Cloving CLI
2.1 Installation
Install Cloving globally with npm:
npm install -g cloving@latest
2.2 Configuration
Configure Cloving by supplying your API key and selecting an AI model (GPT-3.5, GPT-4, etc.):
cloving config
Follow the interactive prompts. Once done, Cloving is ready to generate code for your Go microservices.
3. Initializing Your Go Microservice Project
Within your Go project directory (e.g., a folder containing your go.mod
file), run:
cloving init
Cloving scans your folder structure and existing codebase, generating a cloving.json
that stores metadata about your project. This step ensures AI-generated code aligns with your project’s structure and style.
4. Generating a Go Microservice
4.1 Example: User Authentication Service
Let’s say you need a microservice for user authentication with JWT tokens. Instead of writing everything from scratch, prompt Cloving:
cloving generate code --prompt "Generate a Go microservice for user authentication with JWT" --files main.go
Sample Output:
// main.go
package main
import (
"fmt"
"net/http"
"time"
"github.com/dgrijalva/jwt-go"
)
var jwtKey = []byte("my_secret_key")
func GenerateJWT(username string) (string, error) {
token := jwt.NewWithClaims(jwt.SigningMethodHS256, jwt.MapClaims{
"username": username,
"exp": time.Now().Add(time.Hour * 72).Unix(),
})
return token.SignedString(jwtKey)
}
func main() {
http.HandleFunc("/login", func(w http.ResponseWriter, r *http.Request) {
username := r.URL.Query().Get("username")
if username == "" {
http.Error(w, "Missing username", http.StatusBadRequest)
return
}
token, err := GenerateJWT(username)
if err != nil {
http.Error(w, "Error generating token", http.StatusInternalServerError)
return
}
fmt.Fprintf(w, "JWT Token: %s", token)
})
http.ListenAndServe(":8080", nil)
}
Notes:
- Cloving’s code includes JWT generation logic and a rudimentary HTTP handler.
- You can refine or replace library references (like
dgrijalva/jwt-go
togithub.com/golang-jwt/jwt
if you prefer more recent forks) in your prompts or code.
5. Testing Your Go Microservice
5.1 Generating Unit Tests
Once you have the core logic, let Cloving scaffold tests:
cloving generate unit-tests -f main.go
Sample Output:
// main_test.go
package main
import (
"net/http"
"net/http/httptest"
"testing"
)
func TestGenerateJWT(t *testing.T) {
token, err := GenerateJWT("testuser")
if err != nil {
t.Fatalf("Error generating token: %v", err)
}
if token == "" {
t.Error("Expected a valid token, but got an empty string")
}
}
func TestLoginHandler(t *testing.T) {
req, _ := http.NewRequest("GET", "/login?username=testuser", nil)
rr := httptest.NewRecorder()
handler := http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
username := r.URL.Query().Get("username")
if username == "" {
http.Error(w, "Missing username", http.StatusBadRequest)
return
}
token, err := GenerateJWT(username)
if err != nil {
http.Error(w, "Error generating token", http.StatusInternalServerError)
return
}
w.WriteHeader(http.StatusOK)
w.Write([]byte("JWT Token: " + token))
})
handler.ServeHTTP(rr, req)
if rr.Code != http.StatusOK {
t.Errorf("Expected status %v, got %v", http.StatusOK, rr.Code)
}
if rr.Body.String() == "" {
t.Error("Expected token in response body, got empty")
}
}
Notes:
- The snippet includes tests for
GenerateJWT
and the/login
handler. - Expand coverage for edge cases, e.g. missing or invalid parameters, expired tokens, etc.
6. Interactive Enhancement and Optimization
For more complex scenarios (e.g., linking to a database, concurrency patterns, or advanced security checks), open Cloving chat:
cloving chat -f main.go
In the interactive session, you might say:
Add a database call to validate user credentials before generating a token
Cloving will generate code that queries a database (like a mock DB call or referencing a known library), which you can refine further.
7. AI-Driven Commit Messages
Once your changes are staged, let Cloving propose a commit message:
cloving commit
Cloving inspects the changes to your microservice and suggests something like:
Implement user authentication with JWT and basic unit tests
You can accept or tweak before committing to your version control.
8. Best Practices for Cloving + Go Microservices
- Initialize in Each Project
If you maintain multiple microservices, runcloving init
in each. This helps the AI adapt to differences (dependencies, folder structures). - Iterate in Chat
Large features might need multiple steps. Use the chat session to refine or add new endpoints, concurrency patterns, or architecture improvements. - Review
If needed, runcloving generate review
to have Cloving highlight potential pitfalls (like missing error checks or concurrency race conditions). - Test Thoroughly
The initial generated tests from Cloving can be expanded for more complex scenarios, ensuring robust coverage. - Performance
If performance is critical, mention it in your prompt. Cloving can produce code or suggestions for concurrency (goroutines), load balancing, or advanced caching.
9. Example Workflow
A typical approach to using Cloving for Go microservices might be:
- Initialize:
cloving init
in your microservice’s root. - Generate: e.g.
cloving generate code --prompt "Create a simple user registration microservice in Go" -f main.go
. - Test:
cloving generate unit-tests -f main.go
. - Refine:
cloving chat -f main.go
for advanced logic or performance improvements (e.g. concurrency patterns). - Commit:
cloving commit
for an AI-generated summary of changes.
10. Conclusion
Cloving CLI can greatly accelerate your Go microservice development by auto-generating code, tests, and facilitating iterative improvements. By integrating AI into your daily workflow, you can focus on higher-level architecture and problem-solving, rather than boilerplate or repetitive tasks.
Remember: Cloving’s AI outputs are a starting point – always review them for domain-specific correctness, performance, and best practices. With the synergy of Go’s efficiency and Cloving’s AI-driven approach, your microservices can reach new heights of reliability and speed.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.