Generating AI-Powered Node.js REST API Services with GPT
Updated on March 30, 2025

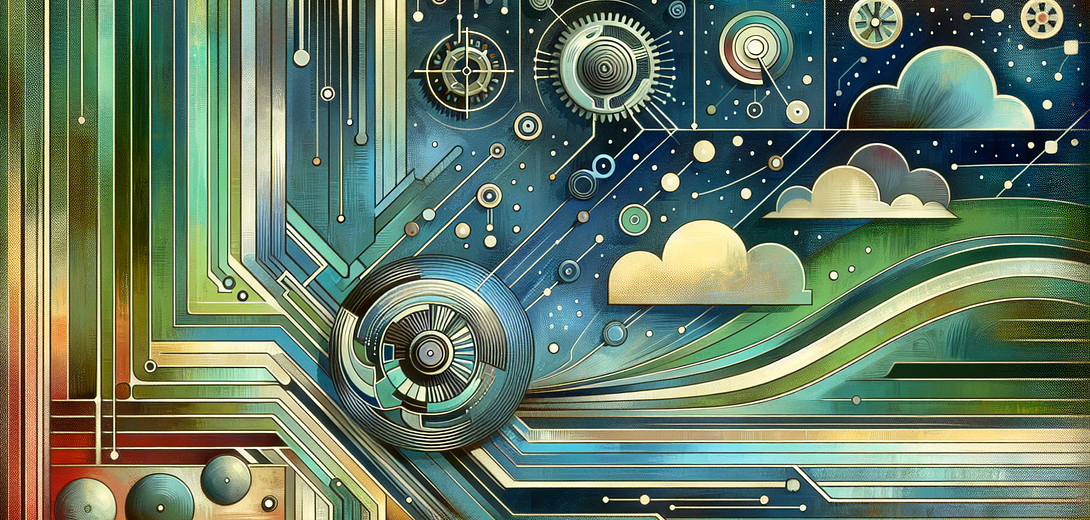
Speeding Up Node.js REST API Development with Cloving CLI
Node.js has become a fundamental platform for building efficient and scalable REST APIs. Yet, as your codebase grows, handling repetitive tasks or boilerplate can hinder productivity. Cloving CLI, an AI-powered tool leveraging GPT-based models, streamlines your workflow by generating and refining server code, unit tests, and more. In this tutorial, we’ll demonstrate how you can harness Cloving CLI to rapidly produce high-quality Node.js REST APIs.
1. Introduction to Cloving CLI
Cloving CLI functions as an AI-driven pair programmer, assisting in:
- Generating or enhancing JavaScript code for Node.js
- Creating unit tests for code coverage
- Refining code logic and architecture through an interactive chat
- Reviewing existing code to spot potential improvements
- Crafting informative Git commit messages
By incorporating context from your project, Cloving can adapt the AI-generated code to your existing structures and conventions.
2. Setting Up Your Environment
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
After installing, configure Cloving by specifying your API key and AI model:
cloving config
Follow the interactive setup (e.g., GPT-3.5, GPT-4) to finalize your environment. Once set up, Cloving is ready to generate or refine Node.js code.
2.3 Project Initialization
Within your Node.js project directory—where package.json
and your main application files typically reside—run:
cloving init
Cloving creates a cloving.json
file, analyzing your project layout and storing context so the AI can produce relevant code generation.
3. Generating a Node.js REST API Service
3.1 Example: User Data Management
Let’s suppose you need a REST API to manage user data (create, retrieve, update, delete). Prompt Cloving:
cloving generate code --prompt "Create a Node.js REST API service for handling user data with endpoints for user creation, retrieval, updating, and deletion" --files src/server.js
Sample Output:
// src/server.js
const express = require('express');
const app = express();
app.use(express.json());
const users = [];
app.post('/users', (req, res) => {
const user = req.body;
users.push(user);
res.status(201).send(user);
});
app.get('/users', (req, res) => {
res.send(users);
});
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === req.params.id);
if (user) {
res.send(user);
} else {
res.status(404).send({ error: 'User not found' });
}
});
app.put('/users/:id', (req, res) => {
const index = users.findIndex(u => u.id === req.params.id);
if (index !== -1) {
users[index] = req.body;
res.send(users[index]);
} else {
res.status(404).send({ error: 'User not found' });
}
});
app.delete('/users/:id', (req, res) => {
const index = users.findIndex(u => u.id === req.params.id);
if (index !== -1) {
users.splice(index, 1);
res.status(204).send();
} else {
res.status(404).send({ error: 'User not found' });
}
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Notes:
- Cloving automatically sets up standard CRUD operations (POST, GET, PUT, DELETE).
- Adjust the data store from an array to a DB or an external service if desired.
3.2 Refining the Generated Code
If you want to add schema validation or handle advanced error cases, mention them in your prompt or utilize the interactive chat for iterative improvements.
4. Code Refinement with Cloving Chat
4.1 The Chat Feature
When you need to expand or optimize the generated code, open an interactive session:
cloving chat -f src/server.js
In the chat environment, you can:
- Ask for input validation or advanced logging
- Refine concurrency patterns (if using event loops or external APIs)
- Integrate data layers (e.g., Mongoose, Sequelize)
Example:
cloving> Add validation so that user objects require an email field
Cloving modifies or suggests code that checks for an email
property before insertion.
5. Generating Unit Tests
5.1 Example: Testing with Jest or Mocha
Ensuring a robust Node.js service often includes test coverage. Let Cloving produce tests for your newly generated endpoints:
cloving generate unit-tests -f src/server.js
Sample Output:
// src/server.test.js
const request = require('supertest');
const app = require('./server.js');
describe('User API', () => {
it('creates a new user', async () => {
const response = await request(app)
.post('/users')
.send({ id: '1', name: 'Alice' });
expect(response.statusCode).toBe(201);
expect(response.body.name).toBe('Alice');
});
it('retrieves all users', async () => {
const response = await request(app).get('/users');
expect(response.statusCode).toBe(200);
});
// Additional tests...
});
Notes:
- Cloving references typical Node testing frameworks like Jest or Mocha.
- If your code uses a custom framework, mention it in the prompt or have an existing test reference for style continuity.
6. Streamlining Git Commits
6.1 Generating Commit Messages
After finalizing or refining code, skip writing commit messages manually:
cloving commit
Cloving scans your staged changes, proposing an informative commit message (e.g., “Add user CRUD endpoints and initial test coverage in server.js”). You can edit or accept it before committing.
7. Best Practices for Node.js + Cloving
- Initialize in the Project Root
Runningcloving init
in each microservice or Node project ensures Cloving can parse your package.json, local imports, etc. - Iterate
For advanced scenarios—like hooking up an ORM or asynchronous flows—use the chat environment multiple times to refine code. - Review
Always check AI-generated code or runcloving generate review
for a quick AI-based scan of potential improvements. - Expand Tests
Cloving’s test scaffolding is a starting point—add negative cases, concurrency checks, or more complex integration tests as needed. - Mention Tools
If you prefer TypeScript, mention it in your prompt. If you use advanced frameworks (like NestJS or Koa), specify them.
8. Conclusion
By integrating Cloving CLI into your Node.js development workflow, you can harness AI to quickly generate REST APIs, test coverage, and iterative improvements. Cloving’s GPT-based intelligence reduces boilerplate and fosters a more efficient code review process, letting you invest time in deeper architectural and performance considerations.
Remember: Cloving is an assistant, not a replacement for your expertise. Always validate the final code for domain-specific correctness, security considerations, and performance constraints. Embrace AI to streamline your Node.js development, creating robust, well-tested APIs with minimal overhead.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.