Exploring Python Code Generation for Machine Learning Algorithms Using AI
Updated on February 06, 2025

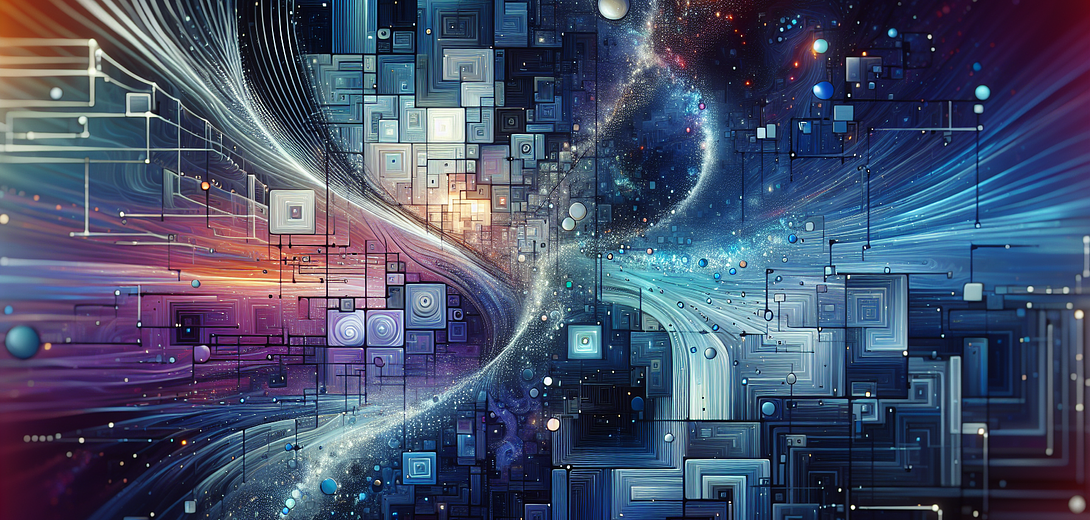
In the ever-evolving world of machine learning, efficiency and precision are paramount. The Cloving CLI tool simplifies and accelerates the development of machine learning algorithms by leveraging AI to generate high-quality code directly in your development environment. This post will guide you through using Cloving to effectively generate Python code for machine learning algorithms, optimizing your workflow.
Getting Started with Cloving
To harness the full potential of Cloving, begin by setting it up in your development environment.
1. Installation and Configuration
First, install Cloving:
npm install -g cloving@latest
Then configure it by setting up your API key and selecting your preferred AI model:
cloving config
Follow the prompts to complete the setup. This ensures Cloving is ready to assist you with generating Python code.
2. Initializing Your Machine Learning Project
Before Cloving can generate context-aware scripts, initialize your project using:
cloving init
This command configures Cloving to understand your project’s structure and context, creating a cloving.json
file that stores essential metadata.
Generating Python Code for Machine Learning
The Cloving CLI can generate Python code tailored for machine learning algorithms. Here’s how you can leverage Cloving for this purpose:
3. Generating a Machine Learning Model
Suppose you need to create a simple linear regression model using Scikit-learn. Use the cloving generate code
command with an appropriate prompt:
cloving generate code --prompt "Create a linear regression model using Scikit-learn in Python" --model python
Cloving analyzes your context and generates a Python script that implements a linear regression model:
import numpy as np
from sklearn.linear_model import LinearRegression
# Sample data
X = np.array([[1, 2], [2, 3], [3, 4], [4, 5]])
y = np.array([6, 8, 9, 11])
# Create and train the model
model = LinearRegression()
model.fit(X, y)
# Predictions
predictions = model.predict(np.array([[5, 6]]))
print("Predictions:", predictions)
4. Generating Code for Data Preprocessing
Data preprocessing is crucial in machine learning workflows. You can generate code snippets for common preprocessing tasks:
cloving generate code --prompt "Generate Python code to preprocess CSV data using Pandas"
The generated Python code might look like this:
import pandas as pd
# Load data
data = pd.read_csv('data.csv')
# Handle missing values
data.fillna(method='ffill', inplace=True)
# Feature encoding
data['category'] = data['category'].astype('category')
data['category_code'] = data['category'].cat.codes
print(data.head())
5. Creating Unit Tests for Machine Learning Code
Ensure code quality by generating unit tests for your machine learning scripts:
cloving generate unit-tests -f src/ml_model.py
This generates tests that validate model functionality, providing a robust framework for testing machine learning scripts.
import unittest
from sklearn.linear_model import LinearRegression
import numpy as np
class TestLinearRegression(unittest.TestCase):
def test_model_prediction(self):
model = LinearRegression()
X = np.array([[1, 2], [2, 3]])
y = np.array([6, 8])
model.fit(X, y)
prediction = model.predict(np.array([[3, 4]]))
self.assertAlmostEqual(prediction[0], 10, delta=0.1)
if __name__ == '__main__':
unittest.main()
Interactive Chat and Code Refinement
For more complex tasks, use Cloving’s chat feature to interact with AI and refine your code iteratively:
cloving chat -f src/ml_model.py
In chat mode, you can request code explanations, ask questions, or revise components interactively.
Best Practices and Tips
- Iterate Often: Leverage Cloving’s interactive modes to iteratively improve your ML scripts.
- Contextualize Commands: Use related files as context when generating code for more accurate outputs (
--files
option). - Experiment with Models: Different AI models might perform better depending on the complexity of the task. Explore Cloving’s available models using
cloving models
.
Conclusion
By integrating AI into your machine learning development workflow, Cloving significantly enhances productivity and efficiency. From generating code snippets and preprocessing functions to constructing entire ML models, Cloving’s capabilities streamline the coding process, allowing you to focus on innovation and analysis. Utilize Cloving CLI to revolutionize how you develop and test machine learning algorithms in Python and continuously achieve new milestones in efficiency and code quality.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.