Exploring AI-Driven C++ Code Generation
Updated on November 06, 2024

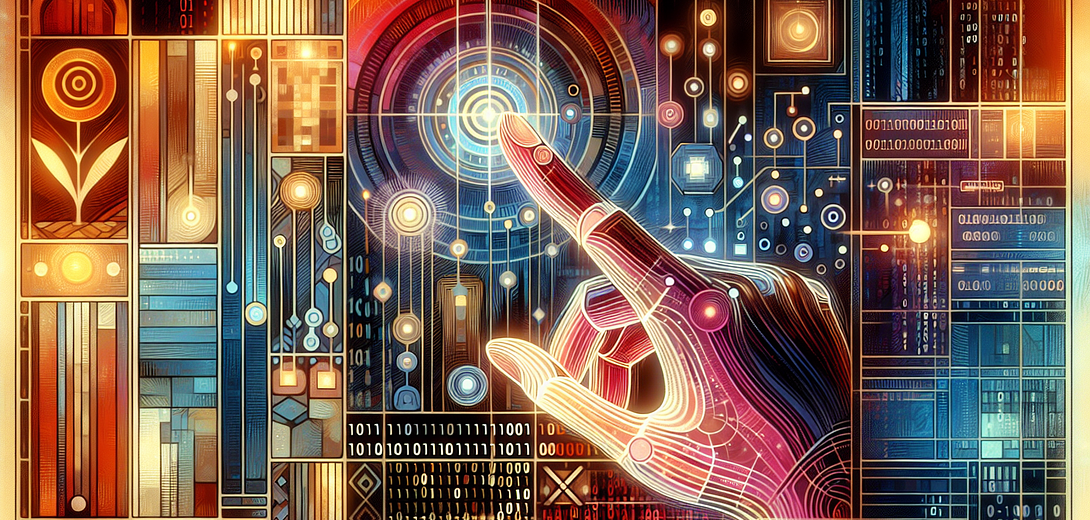
In the world of C++ programming, AI-driven tools like the Cloving CLI can be game-changers. By integrating AI into your development workflow, Cloving enhances productivity by generating code snippets, unit-tests, and even offering real-time interaction through its varied features. This blog post will guide you through the process of leveraging Cloving CLI for effective C++ code generation, with practical examples and best practices along the way.
Getting Started with Cloving CLI
To make the most of Cloving CLI, you need to set it up correctly in your development environment. Here’s how:
Step 1: Installation
First, install Cloving globally using npm:
npm install -g cloving@latest
Step 2: Configuration
Configure Cloving to work seamlessly with your preferred AI model by running:
cloving config
This command will prompt you to set up your API key, model preferences, and other relevant configurations.
Step 3: Initialize Your Project
Navigate to the root directory of your C++ project and initialize Cloving:
cloving init
This will create a cloving.json
file, capturing your project settings and context for improved AI understanding.
Generating C++ Code with Cloving
Once your setup is complete, it’s time to experience AI-assisted code generation for C++.
Example 1: Basic Code Generation
Suppose you need a C++ function to calculate the factorial of a number. Use Cloving to generate it with ease:
cloving generate code --prompt "Create a C++ function to calculate factorial"
Cloving processes your request and context, outputting a function like this:
// factorial.cpp
#include <iostream>
int factorial(int n) {
if (n < 0) return -1; // Invalid for negative numbers
if (n == 0) return 1;
return n * factorial(n - 1);
}
Example 2: Interactive Code Revision
If you need to fine-tune the generated factorial function to handle larger numbers or include error checking, use the interactive feature:
cloving generate code -i --prompt "Optimize factorial function for large numbers"
This command allows you to revise the code iteratively until it meets your requirements.
Generating Unit Tests
Properly tested code is robust and reliable. Cloving can generate unit tests for your C++ code, ensuring quality and correctness.
cloving generate unit-tests -f factorial.cpp
This generates a corresponding test file:
// factorial_test.cpp
#include "factorial.cpp"
#include <iostream>
#include <cassert>
void testFactorial() {
assert(factorial(0) == 1);
assert(factorial(1) == 1);
assert(factorial(5) == 120);
assert(factorial(-1) == -1); // Edge case
}
int main() {
testFactorial();
std::cout << "All tests passed!" << std::endl;
return 0;
}
Utilizing Cloving Chat
For complex tasks requiring real-time assistance, leverage Cloving’s interactive chat feature:
cloving chat -f main.cpp
This opens a session where you can communicate with Cloving AI, request explanations, or generate specific components of your application. The chat is adept at juggling multiple interactions, making it ideal for nuanced coding challenges.
Best Practices for Using Cloving CLI
-
Set Context with Initial Files: Always use
--files
to provide context files, helping Cloving generate more relevant code. -
Leverage Interactive Mode: Utilize the interactive mode for code generation and revision, ensuring your output aligns with your expectations.
-
Commit with AI Assistance: Use
cloving commit
to generate insightful commit messages, improving your repositories’ documentation quality. -
Model Selection: Choose the most suitable AI model for your project using
--model
, as this can significantly affect output quality. -
Explore Cloving’s Documentation: Keep the Cloving documentation handy for command references and tips.
Conclusion
Integrating Cloving CLI into your C++ development workflow harnesses the power of AI to streamline tasks, generate high-quality code, and ensure software reliability. By following the practices outlined here, you can unlock the full potential of AI-driven programming with Cloving. Embrace the future of coding and enhance your productivity today!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.