Exploring Advanced C# Features with GPT Guidance for Enterprise Apps
Updated on January 02, 2025

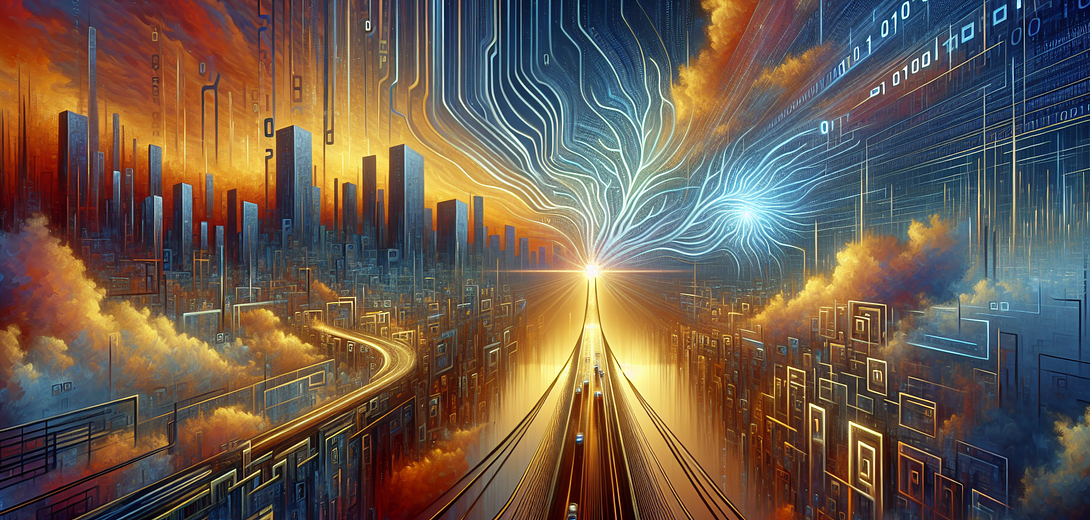
In enterprise app development, using advanced C# features can greatly enhance code efficiency, maintainability, and performance. By integrating AI, specifically the Cloving CLI, into your workflow, you can intuitively explore and implement these features, thus leveraging the full power of C# in your projects. In this blog post, we’ll dive into how you can use the Cloving CLI to effectively navigate and apply advanced C# features in your enterprise applications.
Setting Up Cloving for C#
Before exploring C# with GPT’s guidance, ensure that Cloving is appropriately set up in your development environment.
Installation and Configuration
Install Cloving CLI:
Set up Cloving globally using npm:
npm install -g cloving@latest
Configure Cloving:
Start the configuration process to link your API key and preferred GPT model:
cloving config
This setup ensures you’re ready to leverage GPT’s power for C# insights.
Initializing Your Project
To allow Cloving to understand the context of your project, initiate it within your project’s root directory:
cloving init
This command creates a cloving.json
file, which holds metadata that enhances contextual understanding for the AI.
Mastering Advanced C# Features
Let’s look at how you can explore advanced C# features with the assistance of Cloving, improving your enterprise application development process.
1. Async and Await
Asynchronous programming is critical in enterprise applications for responsive and efficient code. You can use Cloving to generate code snippets that implement async and await patterns.
Example:
Suppose you want to fetch data from multiple APIs concurrently. Use Cloving’s code generation feature:
cloving generate code --prompt "Demonstrate async and await to fetch data from multiple APIs concurrently" --files src/Program.cs
Generated Code:
// src/Program.cs
public async Task FetchDataFromApisAsync()
{
var api1Task = HttpClient.GetStringAsync("https://api.example.com/data1");
var api2Task = HttpClient.GetStringAsync("https://api.example.com/data2");
await Task.WhenAll(api1Task, api2Task);
Console.WriteLine("API1 Data: " + api1Task.Result);
Console.WriteLine("API2 Data: " + api2Task.Result);
}
2. Nullable Reference Types
Ensure null safety for reference types to prevent runtime null reference exceptions, critical in large-scale applications.
Example:
To understand and apply nullability annotations, request Cloving for a clear demonstration:
cloving generate code --prompt "Implement nullable reference types in a C# class" --files src/Employee.cs
Generated Code:
// src/Employee.cs
public class Employee
{
public string? Name { get; set; }
public string Department { get; set; } = string.Empty;
public Employee(string? name, string department)
{
Name = name;
Department = department;
}
}
3. Pattern Matching Enhancements
Utilize pattern matching for clean, readable, and maintainable code critical for complex business logic. Ask Cloving to provide an example:
cloving generate code --prompt "Use C# pattern matching enhancements in switch expressions" --files src/PolicyEvaluator.cs
Generated Code:
// src/PolicyEvaluator.cs
public static string EvaluatePolicy(object policy)
{
return policy switch
{
PolicyType.Exceptional => "Handle with care",
PolicyType.Normal => "Standard procedure",
int hours when hours < 10 => "Urgent attention required",
_ => "Unknown policy type"
};
}
4. Records for Immutable Data
Records simplify the creation of immutable, data-centric entities - a common need in enterprise apps.
Example:
Ask Cloving to illustrate a record for a transaction entity:
cloving generate code --prompt "Define a record for a financial transaction" --files src/Transaction.cs
Generated Code:
// src/Transaction.cs
public record Transaction
{
public int Id { get; init; }
public decimal Amount { get; init; }
public string Currency { get; init; }
public DateTime Date { get; init; }
}
Advanced Cloving Chat for C# Queries
For complex C# tasks or troubleshooting, use Cloving’s chat feature to interactively explore solutions and insights:
cloving chat -f src/YourModule.cs
This feature allows you to ask specific questions, request feature deep-dives, or guide through debugging sessions.
Generating Unit Tests with Cloving
Ensure your advanced C# features are reliable by generating comprehensive unit tests. For instance, to verify the integrity of your transaction records:
cloving generate unit-tests -f src/Transaction.cs
Enhance Your Git Commits
Utilize Cloving to create well-structured commit messages reflective of your advanced C# feature implementations:
cloving commit
Conclusion
Integrating Cloving CLI into your development workflow provides a robust AI-powered support system, enhancing your exploration and implementation of advanced C# features. This integration facilitates efficient, quality-focused development of enterprise applications. Leverage Cloving to not only increase your productivity but to make your code more maintainable and scalable.
Remember, while AI can guide and assist, it should complement your deep understanding and expertise in coding advanced C# features. Embrace this synergy for maximum impact in your enterprise projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.