Enhancing TypeScript Application Scalability with GPT
Updated on March 31, 2025

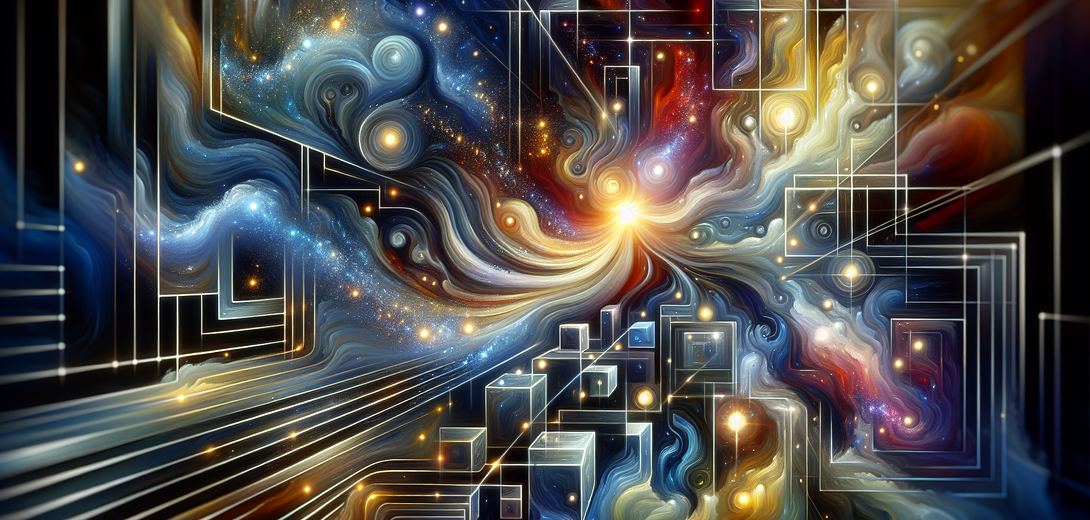
In today’s world of ever-evolving software applications, scalability is crucial, particularly for TypeScript developers. When dealing with scalability challenges, integrating AI into your workflow can significantly streamline the process. The Cloving CLI is an AI-powered command line interface that enhances productivity, offering features like code generation, code review, and more. In this blog post, we’ll explore how to utilize the Cloving CLI to enhance the scalability of TypeScript applications using AI models like GPT (Generative Pre-trained Transformer).
Setting Up Cloving for TypeScript Projects
Before diving into scalability enhancements, ensure Cloving is properly set up in your TypeScript project.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to use your preferred AI model, such as GPT, and set your API key:
cloving config
Follow the interactive prompts to select the AI model and input your API key.
Initialization:
Initialize Cloving in your TypeScript project directory to provide context:
cloving init
This command analyzes your project and creates a cloving.json
file with metadata about your application.
Enhancing Scalability with Cloving
One of the key benefits of using AI in your workflow is to facilitate code enhancements, enabling your TypeScript application to scale efficiently.
1. Refactoring for Scalability
Refactoring code is a critical step toward scalability. Use Cloving to refactor your TypeScript code with AI insights:
cloving generate code --prompt "Refactor the AuthService to improve scalability" --files src/services/AuthService.ts
The above command will analyze your AuthService file and generate refactored code, applying best practices for scalability.
Example Refactored Code:
export class AuthService {
// Improved user authentication mechanism using async/await
async authenticateUser(email: string, password: string): Promise<boolean> {
try {
// Simulate external authentication process
const isValidUser = await this.externalService.verifyUser(email, password);
return isValidUser;
} catch (error) {
console.error('Error during authentication:', error);
return false;
}
}
}
2. Scale Code Organization with Modularity
Organizing your code into clear, modular structures is crucial for maintaining scalability. Leverage the Cloving CLI to generate code that adheres to modularity principles:
cloving generate code --prompt "Create a module for handling database actions" --files src/database/DatabaseUtils.ts
This will generate a well-structured module that separates database actions from the main application logic.
Example of Modularized Code:
// src/database/DatabaseUtils.ts
export class DatabaseUtils {
static connect() {
// Logic to connect to database
}
static fetchRecord(id: string) {
// Logic to fetch a record by ID
}
}
3. Using Interactive Chat for Real-Time Solutions
When faced with complex scalability issues, you can use the Cloving chat feature for real-time, interactive solutions:
cloving chat -f src/app.ts
Enter the chat mode and work collaboratively with the AI to resolve scalability challenges, structuring conversations like:
cloving> How can I optimize the performance of data fetching in my app?
Let's improve the data-fetching mechanism by implementing caching using Redis...
4. Conducting AI-Powered Code Reviews
Ensure code quality with Cloving’s AI-powered code reviews:
cloving generate review -f src/**/*.ts
The AI model will provide insights and suggest improvements to enhance the scalability of your TypeScript application.
Sample Code Review Feedback:
# Code Review: Enhancements for Scalability
1. **Component Separation:** Consider separating logic into distinct components to reduce complexity.
2. **Asynchronous Performance:** Use asynchronous patterns (async/await) for non-blocking I/O operations.
3. **Caching Layer:** Implement a caching mechanism to improve response times for frequent queries.
Conclusion
Integrating the Cloving CLI into your TypeScript development workflow allows for seamless scalability enhancements. By leveraging its code generation, modularization, interactive chat, and code review features, you can effectively optimize your TypeScript applications. Embrace the power of AI to enhance productivity and scalability, unlocking the full potential of your applications through improved code structures and performance optimizations.
By incorporating these tools and practices, you can ensure your TypeScript applications grow efficiently and maintain high performance as they scale. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.