Enhancing React Component Performance with GPT-Centric Optimization
Updated on April 17, 2025

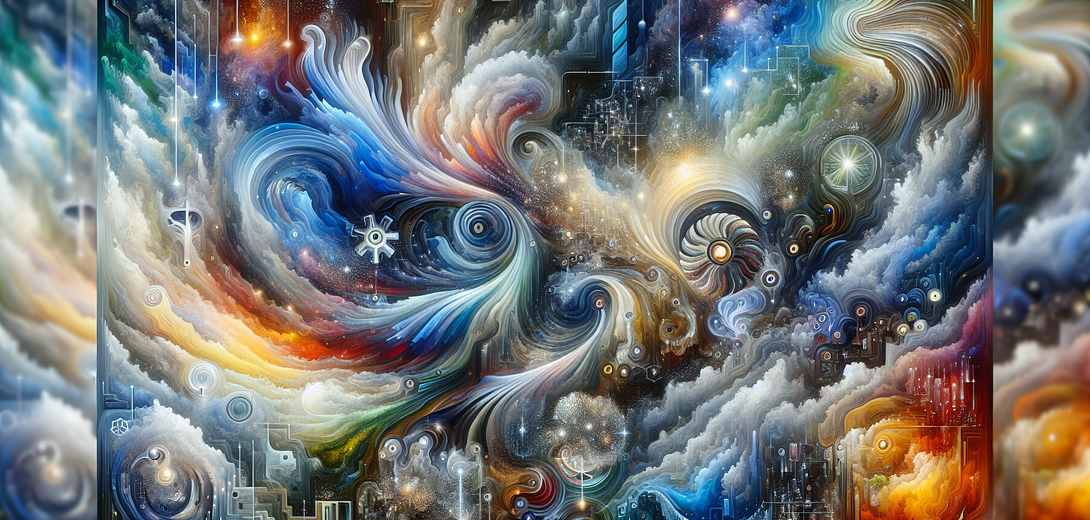
In the world of modern web development, optimizing your React components for performance is crucial to delivering a seamless user experience. The Cloving CLI tool, powered by AI, offers an innovative approach to optimizing your React components by leveraging the capabilities of GPT models. This post will guide you through integrating GPT-centric optimization into your React projects using Cloving CLI and demonstrate how it can enhance component performance effectively.
Cloving CLI: A Quick Introduction
Cloving is a robust command-line tool designed to seamlessly integrate AI into the developer workflow, acting as a powerful collaborator. It can help you generate optimized code, conduct code reviews, and much more, enhancing your overall productivity.
Getting Started with Cloving
Before we dive into optimization strategies, let’s set up Cloving in your development environment:
-
Installation:
Begin by installing Cloving globally with npm:
npm install -g cloving@latest
-
Configuration:
Configure Cloving to connect with your preferred AI model:
cloving config
Follow the prompts to set up your API key and choose your desired GPT model.
-
Project Initialization:
Initialize Cloving within your React project directory:
cloving init
This process sets up the necessary context for your project, enabling Cloving to understand the structure and dependencies of your React application.
Optimizing React Components with Cloving
To enhance the performance of your React components, you can leverage Cloving’s AI-driven capabilities to optimize code, generate efficient patterns, and conduct automated code reviews.
1. Code Optimization with Cloving
When working on a React component that could benefit from performance tuning, use Cloving to generate optimized code.
Example:
Suppose you have a React component that renders a list of items:
// src/components/ItemList.jsx
import React from 'react';
const ItemList = ({ items }) => {
return (
<div>
{items.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
};
export default ItemList;
To optimize this component, use Cloving to suggest improvements:
cloving generate code --prompt "Optimize React component to render a list of items efficiently" --files src/components/ItemList.jsx
The AI might suggest using React.memo
and efficient rendering techniques:
import React, { memo } from 'react';
const Item = memo(({ name }) => <div>{name}</div>);
const ItemList = ({ items }) => {
return (
<div>
{items.map(item => (
<Item key={item.id} name={item.name} />
))}
</div>
);
};
export default ItemList;
2. High-Level Component Reviews
Cloving can also perform a high-level code review to identify performance bottlenecks in your components.
Execute:
cloving generate review --files src/components/ItemList.jsx
This command will produce a review highlighting areas for improvement, such as memoization opportunities or state management optimizations.
3. Interactive Code Revisions
For further refinement, you can revise and polish the optimized code through an interactive session.
Start a Cloving Chat Session:
cloving chat -f src/components/ItemList.jsx
In this session, ask for further assistance:
cloving> Suggest best practices for handling large datasets in ItemList component
The AI will provide insights tailored to your specific scenario, helping you handle large datasets more effectively.
4. Unit Test Generation
Improving performance should always be accompanied by robust testing to ensure correctness. Cloving can aid in generating unit tests:
cloving generate unit-tests -f src/components/ItemList.jsx
You will receive comprehensive unit tests ensuring your optimizations do not introduce side-effects.
Conclusion
Integrating GPT-centric optimization techniques using Cloving CLI into your React workflow can significantly enhance component performance. By harnessing AI’s potential, you ensure that your React applications are efficient and responsive, all while streamlining your development process.
Leverage Cloving’s powerful features, such as code generation, code reviews, and chat-based assistance, to create high-performing React components that deliver excellent user experiences. As you incorporate these techniques, remember to continuously test and iterate, ensuring your optimizations align with your project goals.
For more detailed usages and capabilities, refer to the Cloving Documentation.
Happy coding and optimizing with Cloving! 🍀
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.