Enhancing JavaScript Package Development Using AI-Driven Code Generation
Updated on March 30, 2025

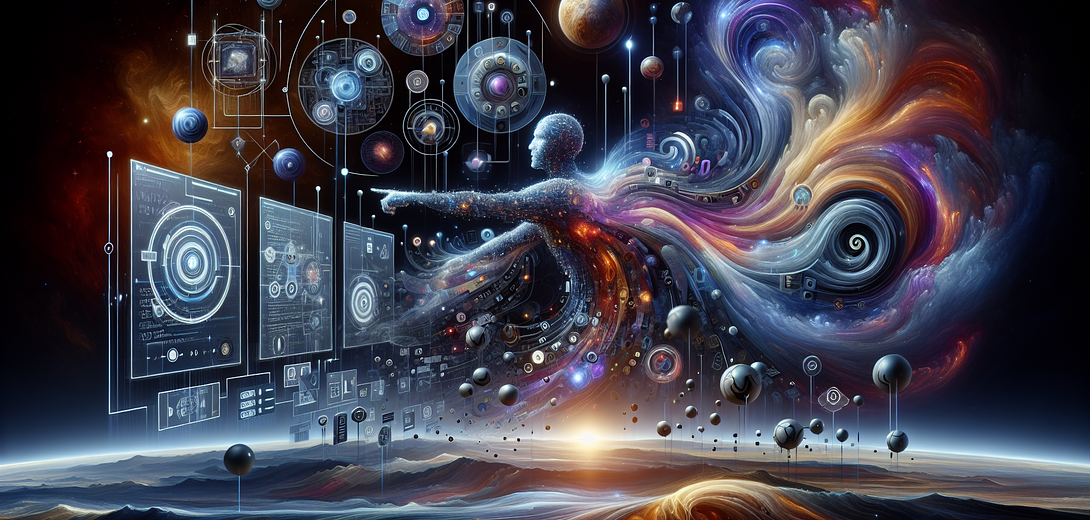
Accelerating JavaScript Package Development with Cloving CLI
JavaScript is at the heart of modern web development, powering everything from front-end frameworks to back-end services. As the ecosystem grows, developers are under increasing pressure to build high-quality packages with minimal overhead. Cloving CLI, an AI-driven command-line interface, simplifies this process by generating code, tests, and even commit messages – freeing you to focus on higher-level logic and innovation. In this post, we’ll explore how to integrate Cloving CLI into your JavaScript package development workflow.
1. Understanding Cloving CLI
Cloving CLI is designed to act like an AI “pair programmer,” assisting with:
- Code generation – from small utility functions to entire modules
- Test creation – automatically scaffolding test files for your code
- Interactive refinement – adjusting generated code via a chat-based environment
- Code reviews – scanning for potential improvements
- Commit messages – generating meaningful, contextual commit messages
By providing your project context, Cloving can tailor its outputs, aligning with your package’s structure and style.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
2.2 Configuration
Configure Cloving with your API key and AI model (e.g., GPT-3.5, GPT-4):
cloving config
Follow the interactive prompts to finalize your environment. Once complete, Cloving can generate code and test stubs for your JavaScript package.
2.3 Project Initialization
Inside your JavaScript package’s root (where package.json
typically resides), run:
cloving init
Cloving analyzes your project, storing metadata in cloving.json
. This helps it produce relevant code aligned with your existing setup.
3. Generating JavaScript Code with Cloving
3.1 Example: Creating Utility Functions
Imagine you need a utility function to compute the difference between two arrays. Let Cloving handle the boilerplate:
cloving generate code --prompt "Write a utility function to find the difference between two arrays" --files utils.js
Sample Output:
// utils.js
function difference(arr1, arr2) {
return arr1.filter(x => !arr2.includes(x));
}
Notes:
- Cloving generates a concise function – if you prefer TypeScript or advanced checks, mention them in your prompt or refine afterward.
3.2 Additional Examples
If you want a function to deeply merge two objects or parse an .env file, simply prompt Cloving accordingly:
cloving generate code --prompt "Create a function to deep merge two JavaScript objects" --files utils.js
Cloving might produce a snippet that recursively merges nested keys, handling arrays or special cases if you specify them.
4. Generating Unit Tests
4.1 Example: Testing the Utility Function
After generating difference()
, you’ll want tests to ensure correctness:
cloving generate unit-tests -f utils.js
Sample Output:
// utils.test.js
const { difference } = require('./utils');
describe('difference', () => {
it('should return the elements present in the first array but not in the second', () => {
expect(difference([1, 2, 3], [2, 3, 4])).toEqual([1]);
});
it('should return an empty array when both arrays have the same elements', () => {
expect(difference([1, 2], [1, 2])).toEqual([]);
});
});
Notes:
- Cloving references popular JavaScript testing patterns, typically using
jest
style. - If you prefer mocha or other frameworks, mention them in your prompt or keep an example test to guide Cloving’s output.
5. Interacting with Cloving Chat
5.1 Chat-Based Refinement
For advanced tasks—like adding concurrency logic or migrating from CommonJS to ES modules—open Cloving chat:
cloving chat -f utils.js
In the chat environment, you can:
- Ask for performance optimizations (e.g., rewriting filters for large data sets).
- Refine code to handle edge cases (like nested arrays).
- Get guidance on versioning or cross-package usage.
Example:
cloving> Modify the difference function to handle nested arrays gracefully
Cloving updates your function, possibly adding recursion or additional checks.
6. Optimizing Git Workflow
6.1 Generating Commit Messages
When you’re ready to commit changes, skip generic messages. Let Cloving propose an AI-driven message:
cloving commit
Cloving reads your staged changes (like new code in utils.js
plus test updates) and suggests a descriptive commit message – e.g. “Add difference function with initial test coverage.” You can accept or edit it before finalizing the commit.
7. Best Practices for Using Cloving with JavaScript
- Initialize in Each Project
If you manage multiple packages, runcloving init
in each relevant directory so Cloving knows the context. - Refine Iteratively
If your code is complex, break tasks into smaller prompts or rely oncloving chat
to refine step by step. - Review
Let Cloving help but always confirm the final code. Usecloving generate review
for a high-level check (like unused imports or potential pitfalls). - Leverage Testing
Re-runcloving generate unit-tests -f yourFile.js
whenever you add or modify logic, ensuring your test coverage evolves with your code. - Customize
If your project uses advanced tooling (e.g., Babel, ES modules, or specialized linting rules), mention these in your prompt so the AI outputs are aligned with your environment.
8. Example Workflow
Here’s a typical sequence for adopting Cloving in your JavaScript package development:
- Initialize:
cloving init
in your project root. - Generate:
cloving generate code --prompt "Write a function to parse config files" --files src/config.js
. - Refine: If you need expansions (like handling multiple file formats), open chat with
cloving chat -f src/config.js
. - Test:
cloving generate unit-tests -f src/config.js
for coverage. - Review:
cloving generate review
if you want an AI-driven code scan. - Commit: Summarize changes with
cloving commit
.
9. Conclusion
By integrating Cloving CLI into your JavaScript package development, you tap into an AI-powered approach that accelerates code generation, ensures robust testing, and refines your workflow. Cloving’s GPT-based intelligence helps handle repetitive tasks, letting you focus on architectural decisions, performance tuning, or creative problem solving.
Remember: Cloving’s outputs serve as a foundation. Always review AI-generated code for domain-specific correctness, performance, and style. By combining Cloving’s automation with your expertise, you’ll maintain a high-quality JavaScript package ecosystem while speeding up development and improvements.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.