Enhancing Code Quality in Python Projects with GPT
Updated on June 26, 2024

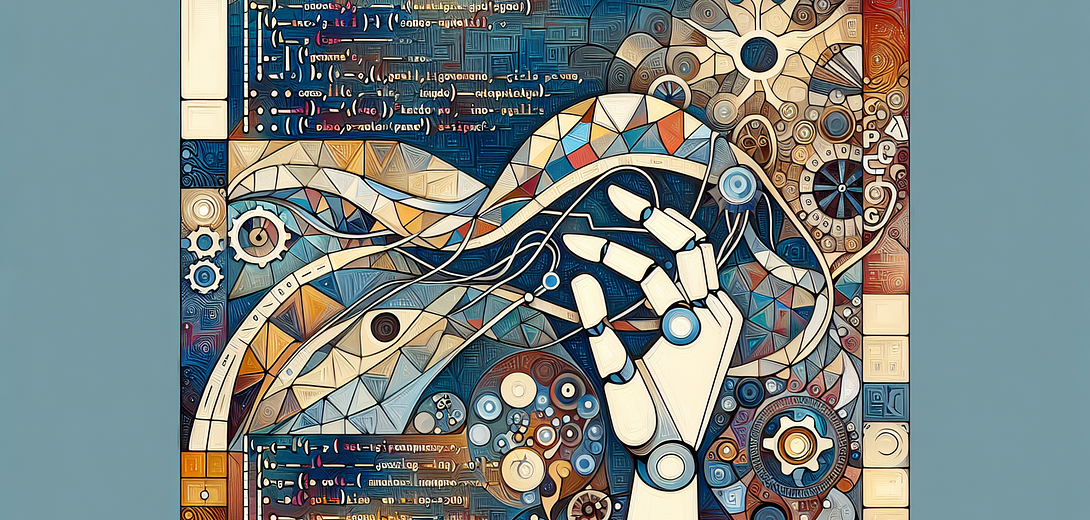
Quality code is the cornerstone of robust applications. Ensuring your code is clean, efficient, and free from bugs can be a daunting task, especially when deadlines loom. By embracing the principles of cloving—integrating human creativity and intuition with the processing power of artificial intelligence (AI)—programmers can significantly improve their code quality and productivity.
One such AI tool is GPT (Generative Pre-trained Transformer), which can assist you in various aspects of your development workflow. In this blog post, we’ll delve into how you can incorporate GPT into your Python projects to enhance code quality and efficiency.
Understanding Cloving
Cloving is about creating a symbiotic relationship between human and machine, allowing them to leverage each other’s strengths. As a developer, you bring creativity, intuition, and domain knowledge to the table. Meanwhile, AI like GPT offers unparalleled analytical capabilities and can process vast amounts of data quickly.
1. Automated Code Reviews
One of the key aspects of maintaining high code quality is regular code reviews. GPT can assist by providing automated code reviews, highlighting potential issues, and suggesting improvements.
Example:
Suppose you want to review a Python function that processes user data. You can prompt GPT for a code review:
Review this Python function for any potential issues or improvements:
```python
def process_user_data(user_data):
processed_data = {}
for key, value in user_data.items():
if isinstance(value, str):
processed_data[key] = value.strip().lower()
elif isinstance(value, int):
processed_data[key] = value + 1
else:
processed_data[key] = value
return processed_data
GPT will analyze the function and might suggest improvements such as handling edge cases, enhancing performance, or improving readability.
2. Code Completion and Suggestions
GPT can help you write Python code more efficiently by providing code completions and suggestions. This can be particularly useful when you’re exploring new libraries or implementing complex logic.
Example:
If you need to write a function to filter a list of users by their registration date, you can prompt GPT:
Write a Python function to filter a list of users by their registration date, keeping only users who registered in the last 30 days.
GPT will generate a function that meets your requirements, saving you time and reducing the likelihood of errors.
from datetime import datetime, timedelta
def filter_recent_users(users):
thirty_days_ago = datetime.now() - timedelta(days=30)
recent_users = [user for user in users if user['registration_date'] >= thirty_days_ago]
return recent_users
3. Learning and Adapting Best Practices
Staying updated with best practices and new advancements in Python can be challenging. GPT can serve as a knowledgeable guide, offering insights and explanations.
Example:
To learn about the current best practices for writing efficient Python code, you can ask GPT:
What are the best practices for writing efficient and maintainable Python code in 2024?
GPT will provide you with a comprehensive list of tips and best practices, helping you stay current with industry standards.
4. Generating Unit Tests
Testing is crucial for ensuring code quality, but writing unit tests can be repetitive. GPT can help automate this process by generating relevant test cases based on your code.
Example:
If you have written a new function and need to create tests, you can prompt GPT:
Generate unit tests for this Python function:
```python
def add_numbers(a, b):
return a + b
GPT will produce a set of unit tests, ensuring your function is thoroughly tested and helping you catch any potential bugs early.
import unittest
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add_numbers(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
5. Documentation and Explanation
Understanding and documenting complex code can be challenging. GPT can assist by generating clear explanations and documentation for your code.
Example:
When you need to document a complex class or method, you can ask GPT:
Generate documentation for this Python class:
```python
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def get_contact_info(self):
return {'name': self.name, 'email': self.email}
GPT will create detailed documentation, making it easier for you and your team to understand and maintain the code.
class User:
"""
A class to represent a user.
Attributes:
-----------
name : str
The name of the user.
email : str
The email address of the user.
Methods:
--------
get_contact_info():
Returns a dictionary with the user's name and email address.
"""
def __init__(self, name, email):
"""
Constructs all the necessary attributes for the user object.
Parameters:
-----------
name : str
The name of the user.
email : str
The email address of the user.
"""
self.name = name
self.email = email
def get_contact_info(self):
"""
Returns a dictionary with the user's contact information.
Returns:
--------
dict
A dictionary with the user's name and email address.
"""
return {'name': self.name, 'email': self.email}
Conclusion
Enhancing code quality in Python projects with the assistance of GPT epitomizes the concept of cloving. By fusing human creativity and intuition with AI’s processing capabilities, you can significantly improve the efficiency and effectiveness of your development workflow. Embrace cloving and discover how this synergistic approach can transform your programming experience, leading to cleaner, more robust code.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the processes described above:
How can I configure GitHub to run these tests automatically for me?
And here is another:
Generate accompanying factories, for example, data for these unit tests.
And one more:
What are other GPT prompts I could use to make this more efficient?
By integrating these prompts and the principles of cloving into your daily routine, you’ll find yourself coding smarter and faster, with GPT by your side.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.