Enhancing C# Game Development Pipelines with GPT-Driven Automation
Updated on January 06, 2025

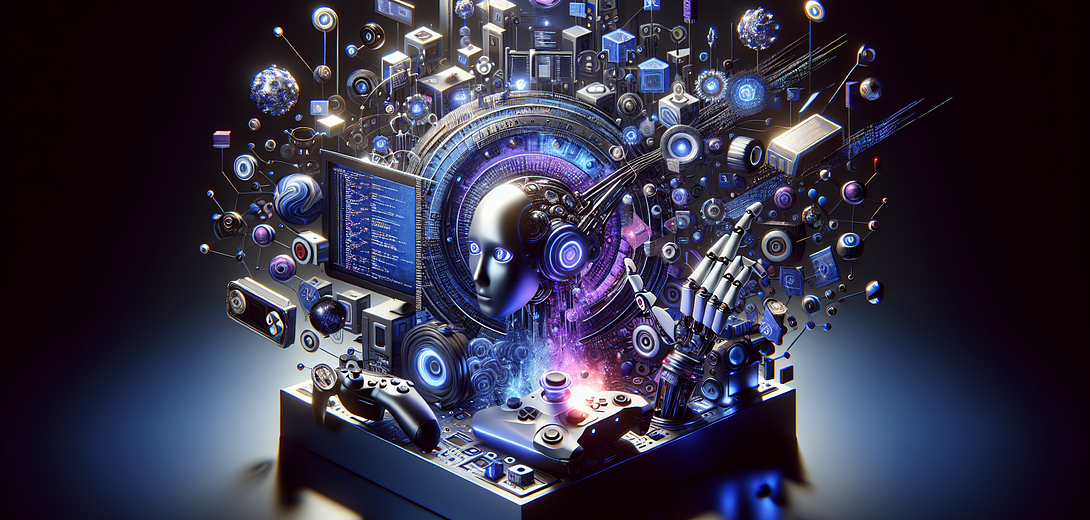
As the game development industry continues to evolve, developers are constantly on the lookout for tools that can enhance their workflows, increase productivity, and maintain code quality. The Cloving CLI, an AI-powered command-line interface, is the perfect companion for C# game developers aiming to automate repetitive tasks, generate high-quality code, and streamline their development pipelines. In this post, we’ll dive into how the Cloving CLI can revolutionize your game development process.
Cloving CLI: Your AI Assistant
Before we delve into specific workflows, let’s ensure Cloving is properly set up in your environment.
Setting Up Cloving
To get started, you’ll need to install Cloving globally. Using npm, the Node.js package manager, follow these steps:
npm install -g cloving@latest
Configuring Cloving
After installation, set up Cloving to interact with your preferred AI model. This step ensures that Cloving operates with the necessary permissions and configurations to optimize its capabilities for your workflow.
cloving config
During configuration, you’ll be prompted to enter your API key and select from the list of available AI models. This setup ensures Cloving is tuned to your specific requirements and ready for integration.
Initializing Your Project
For Cloving to effectively interact with your C# game project, initialize Cloving in your project directory to understand the context of your codebase:
cloving init
This command analyzes your project and sets up a cloving.json
file that provides a detailed context about your application, setting the stage for seamless AI-assisted development.
Leveraging Cloving in Your Workflow
Armed with its powerful AI capabilities, Cloving offers a range of commands to automate various development tasks:
1. Automate C# Code Generation
For game developers, generating new C# classes, scripts, or components can often lead to repetitive coding tasks. Use Cloving to automate code generation with an AI-driven prompt:
cloving generate code --prompt "Create a C# component for player health management" --files Assets/Scripts/Player.cs
Suppose you’re developing a health management system for a player in Unity using C#. This command results in an AI-generated script that handles health management - a fundamental aspect of game design:
using UnityEngine;
public class PlayerHealth : MonoBehaviour
{
public int MaxHealth = 100;
private int currentHealth;
void Start()
{
currentHealth = MaxHealth;
}
public void TakeDamage(int damage)
{
currentHealth -= damage;
if (currentHealth <= 0)
{
currentHealth = 0;
Die();
}
}
void Die()
{
Debug.Log("Player is dead.");
// Here you could add game over logic or respawning
}
}
2. Generate Unit Tests
Testing your game scripts is crucial for maintaining code quality. Cloving can assist by automatically generating unit tests for your C# scripts:
cloving generate unit-tests -f Assets/Scripts/Player.cs
The generated unit tests ensure that your code behaves as expected, greatly reducing debugging time:
using NUnit.Framework;
public class PlayerHealthTests
{
[Test]
public void TestPlayerTakesDamage()
{
PlayerHealth playerHealth = new PlayerHealth();
playerHealth.TakeDamage(10);
Assert.AreEqual(90, playerHealth.currentHealth);
}
[Test]
public void TestPlayerDiesAtZeroHealth()
{
PlayerHealth playerHealth = new PlayerHealth();
playerHealth.TakeDamage(100);
Assert.AreEqual(0, playerHealth.currentHealth);
}
}
3. Conduct Code Reviews
For collaborative projects, conducting code reviews ensures that new code adheres to project standards and is free from common errors. With Cloving, you can leverage AI to generate code reviews:
cloving generate review
The AI analyses your recent changes and provides insightful feedback, aiding in peer reviews and improving code quality.
4. Interactive Chat for Complex Integrations
Complex game mechanics often require deep discussions and explorations. Use Cloving’s interactive chat to brainstorm and prototype:
cloving chat -f Assets/Scripts/Player.cs
Once in the interactive session, you can ask the AI for specific code snippets or advice on intricate systems. For example, you might request a function to save the player’s state, leading the LLM to generate something like:
void SavePlayerState() {
// Example function to save player state
PlayerPrefs.SetInt("PlayerHealth", currentHealth);
PlayerPrefs.Save();
Debug.Log("Player state saved.");
}
This code snippet illustrates the kind of utility functions an LLM might draft to assist with your development tasks.
Conclusion
Integrating the Cloving CLI into your C# game development workflow can vastly enhance productivity and maintain high code standards. Embrace the power of GPT-driven automation to streamline repetitive tasks, generate unit tests, conduct reviews, and explore new AI-generated solutions—transforming your game development pipeline with cutting-edge AI technology.
Leverage Cloving to augment your skills and work more efficiently, letting you focus on the creative aspects of game development while the AI handles routine coding tasks. Dive into the future of game development and redefine your coding standards with Cloving CLI!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.