Enhancing Android App Development Workflow with Automated Code Generation
Updated on January 30, 2025

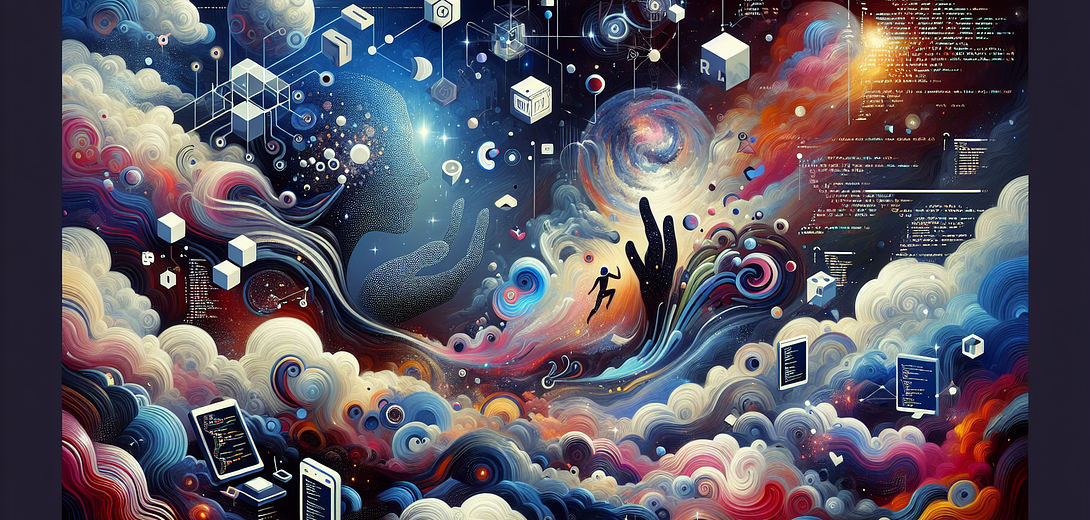
Android app development can be complex, with its multitude of guidelines, styles, and APIs. The Cloving CLI tool simplifies these complexities by integrating AI into your development workflow. This blog post will guide you through using Cloving’s automated code generation to streamline your Android app development process, allowing you to focus on delivering high-quality apps efficiently.
Getting Started with Cloving
Before diving into automated code generation, let’s ensure Cloving is all set up in your environment.
Installation
To start using Cloving, install it globally with npm:
npm install -g cloving@latest
Configuration
Configure Cloving to recognize your API key and the AI model you wish to use:
cloving config
Follow the on-screen prompts to set up, including inputting your API key and selecting the desired AI model.
Initializing Your Android Project
To allow Cloving to understand your Android project’s context, initialize it in your project directory:
cloving init
This command will create a cloving.json
file, which houses metadata relevant to your application.
Generating Android Code
With Cloving configured and ready, let’s explore how to generate Android code, enhancing your app development workflow.
Example: Creating a UI Component
Suppose you’re building a UI component for user login. You can use the Cloving generate
command to create the necessary Android code:
cloving generate code --prompt "Generate a Kotlin activity for user login with fields for email and password"
The AI will process your request and generate relevant code:
// src/main/java/com/example/myapp/LoginActivity.kt
package com.example.myapp
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import android.widget.EditText
import android.widget.Button
import android.widget.Toast
class LoginActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_login)
val emailField = findViewById<EditText>(R.id.emailField)
val passwordField = findViewById<EditText>(R.id.passwordField)
val loginButton = findViewById<Button>(R.id.loginButton)
loginButton.setOnClickListener {
val email = emailField.text.toString()
val password = passwordField.text.toString()
// Example login logic
if (email.isNotEmpty() && password.isNotEmpty()) {
Toast.makeText(this, "Login Successful", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(this, "Please fill in all fields", Toast.LENGTH_SHORT).show()
}
}
}
}
Revising Generated Code
If the generated code requires revising, use Cloving’s interactive mode for further enhancements:
cloving generate code -i --prompt "Include email validation in LoginActivity"
You can iteratively improve the code until it meets your requirements.
Generating Unit Tests
To ensure the stability of your application, leverage Cloving’s ability to generate unit tests:
cloving generate unit-tests -f src/main/java/com/example/myapp/LoginActivity.kt
This command produces unit tests tailored to the LoginActivity
, ensuring your activity functions as expected:
// src/test/java/com/example/myapp/LoginActivityTest.kt
package com.example.myapp
import org.junit.Test
import org.junit.Assert.*
class LoginActivityTest {
@Test
fun testEmailValidation() {
val validEmail = "[email protected]"
val invalidEmail = "test.example.com"
assertTrue(isValidEmail(validEmail))
assertFalse(isValidEmail(invalidEmail))
}
// Add more tests here
}
Using Cloving Chat for Live Assistance
For complex tasks or real-time assistance, initiate an interactive chat session with Cloving:
cloving chat -f src/main/java/com/example/myapp/LoginActivity.kt
Interact with the AI by asking specific questions or requesting previews of additional features. For example you could ask it:
- Complete a code review of the following file and create a revised version based on your findings that includes comments noting the changes.
After the AI has generated your new revised file and you have reviewed it you can use the commit command to generate a descriptive and thorough commit message for your changes. This feature especially can dramatically save you time and make your code much more maintainable in the future.
Best Practices
- Understand Generated Context: Always ensure the AI-generated code fits well within your project’s context and architecture.
- Iterative Development: Use Cloving’s interactive options to iteratively refine generated code.
- Review and Test: Always review generated code and write tests to cover your base functionality, ensuring reliability.
Conclusion
By incorporating the Cloving CLI into your Android development workflow, you can streamline the creation of robust UI components, automate testing, and reduce development time. This powerful AI integration adds value by generating boilerplate code, letting you focus on crafting unique features and optimizing user experience.
Remember, while Cloving aids in automation, your expertise is key to crafting engaging and well-thought-out applications. Use Cloving to augment your skills and elevate your Android development processes.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.