Developing Server-Rendered Apps with Next.js Using GPT Insights
Updated on March 05, 2025

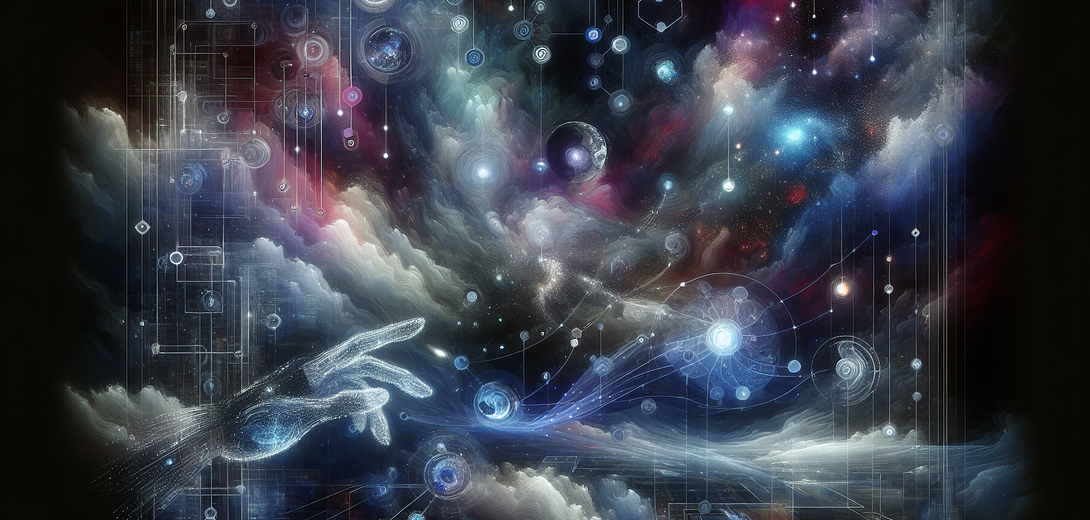
Supercharging Next.js Development with Cloving CLI
Next.js is a powerful React framework for building server-rendered applications, offering features like server-side rendering (SSR) and static site generation (SSG). While Next.js simplifies much of the boilerplate, there are still plenty of tasks – from scaffolding pages to reviewing code – that can eat up development time. Cloving CLI, an AI-powered command-line tool, steps in to streamline your workflow, boost productivity, and help maintain code quality.
1. Understanding Cloving CLI
Cloving acts as an AI-enhanced pair programmer, helping you:
- Generate new code: components, pages, routes, etc.
- Review existing code for potential improvements.
- Assist with iterative development via an interactive chat.
- Propose meaningful commit messages based on recent changes.
By harnessing GPT-based models, Cloving adapts to your project’s structure and coding style, delivering relevant and context-aware solutions.
2. Setup and Configuration
2.1 Installing Cloving
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuring Cloving
Run the configuration command:
cloving config
Follow the interactive prompts to enter your API key and select the AI model you prefer (e.g., GPT-3.5, GPT-4). Cloving will store these settings, enabling it to generate code aligned with your project needs.
3. Initializing Your Next.js Project
With Cloving installed, navigate to your Next.js project’s root and run:
cloving init
This step analyzes your codebase, creating a cloving.json
file. It contains essential metadata allowing Cloving to understand the context (e.g., Next.js structure, directories) for more accurate code generation and suggestions.
4. Generating Server-Rendered Components
One of Next.js’s standout features is server-side rendering (SSR). Let’s explore how Cloving can help you scaffold SSR pages with minimal effort.
4.1 Example: Creating a Blog Post Page
Suppose you’re building a blog application and need a page to display an individual blog post fetched from an external API. Use the generate code
command:
cloving generate code --prompt "Create a Next.js server-rendered page component to display a blog post" --files pages/post/[id].tsx
Sample Output:
// pages/post/[id].tsx
import { GetServerSideProps } from 'next'
import React from 'react'
interface PostProps {
post: {
id: string
title: string
content: string
}
}
const Post: React.FC<PostProps> = ({ post }) => (
<div>
<h1>{post.title}</h1>
<div>{post.content}</div>
</div>
)
export const getServerSideProps: GetServerSideProps = async ({ params }) => {
const response = await fetch(`https://your-api.com/posts/${params?.id}`)
const post = await response.json()
return {
props: {
post,
},
}
}
export default Post
Notes:
- Cloving references Next.js’s server-side rendering pattern, using
getServerSideProps
. - Customize the fetch logic, error handling, or data shape to fit your API.
5. Interactive Coding with Cloving Chat
For more complex tasks or iterative development, Cloving’s chat feature is invaluable:
cloving chat -f ./pages/post/[id].tsx
In the interactive session, you can:
- Request code revisions (e.g., add error handling if the fetch fails).
- Ask for performance optimizations.
- Get clarifications on code generation logic.
This fosters a conversational approach, letting you refine or expand code seamlessly.
6. Enhancing Code Quality with AI-Powered Reviews
Maintaining consistent coding standards is crucial. Let Cloving assess your code for potential improvements:
cloving generate review -f pages/post/[id].tsx
Cloving will highlight possible refactors or best practices (e.g., handling edge cases, implementing type safety, or adopting recommended Next.js patterns).
7. Efficient Commit Messages
Commit messages are vital for clarity in your development history. Cloving can generate messages by analyzing staged changes:
cloving commit
Cloving suggests a context-aware summary, like:
Add dynamic blog post page with server-side rendering for Next.js
You can accept or tweak the suggestion for a clear, descriptive commit history.
8. Further Examples and Use Cases
8.1 Static Site Generation (SSG)
If you prefer static site generation for certain pages, prompt Cloving specifically:
cloving generate code --prompt "Create a Next.js page with static generation for a products list" --files pages/products/index.tsx
Cloving might output a snippet with getStaticProps
and getStaticPaths
if needed.
8.2 API Routes
Next.js includes an API routes feature for building serverless functions. Generate a route:
cloving generate code --prompt "Create a Next.js API route for retrieving user data" --files pages/api/users/[id].ts
Sample Output:
// pages/api/users/[id].ts
import { NextApiRequest, NextApiResponse } from 'next';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const { id } = req.query;
if (req.method === 'GET') {
try {
const response = await fetch(`https://your-api.com/users/${id}`);
const user = await response.json();
return res.status(200).json(user);
} catch (error) {
return res.status(500).json({ error: 'Failed to fetch user' });
}
} else {
return res.status(405).json({ error: 'Method not allowed' });
}
}
9. Best Practices for Cloving + Next.js
- Initialize Early
Runcloving init
at the root of your Next.js project to provide full context to Cloving. - Specific Prompts
Tailor your prompt text. The more detail you provide, the more accurate the code generation. - Iterative Refinement
Use the chat mode or multiple generate commands to refine code incrementally (e.g., add error handling, custom headers, or advanced data fetching logic). - Testing
Cloving can also generate unit or integration tests. Combine Next.js’s recommended testing frameworks (e.g., Jest, React Testing Library) with Cloving’s code generation for robust coverage. - Review Regularly
Runningcloving generate review
after major additions helps catch potential pitfalls early.
10. Example Cloving Workflow with Next.js
- Initialize:
cloving init
in your Next.js project root. - Generate Page:
cloving generate code --prompt "Create a Next.js SSR page for user profiles" -f pages/user/[id].tsx
- Refine: Use
cloving chat -f pages/user/[id].tsx
to add error handling or advanced logic. - Review:
cloving generate review -f pages/user/[id].tsx
for suggestions. - Commit:
cloving commit
to get a summarizing commit message.
11. Conclusion
Cloving CLI integrates AI to streamline your Next.js development. From generating SSR pages and code reviews to interactive refinements, Cloving helps deliver robust, high-quality applications with less repetitive coding. Whether you’re building a blog, e-commerce site, or enterprise dashboard, Cloving can optimize your workflow and reduce potential errors.
Remember: Cloving complements, rather than replaces, your expertise. You maintain design and architectural decisions while Cloving accelerates tedious coding tasks and ensures consistent standards. Embrace AI-powered development and watch your Next.js projects flourish!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.