Developing Secure Microservices in .NET Using GPT-Based Strategies
Updated on February 06, 2025

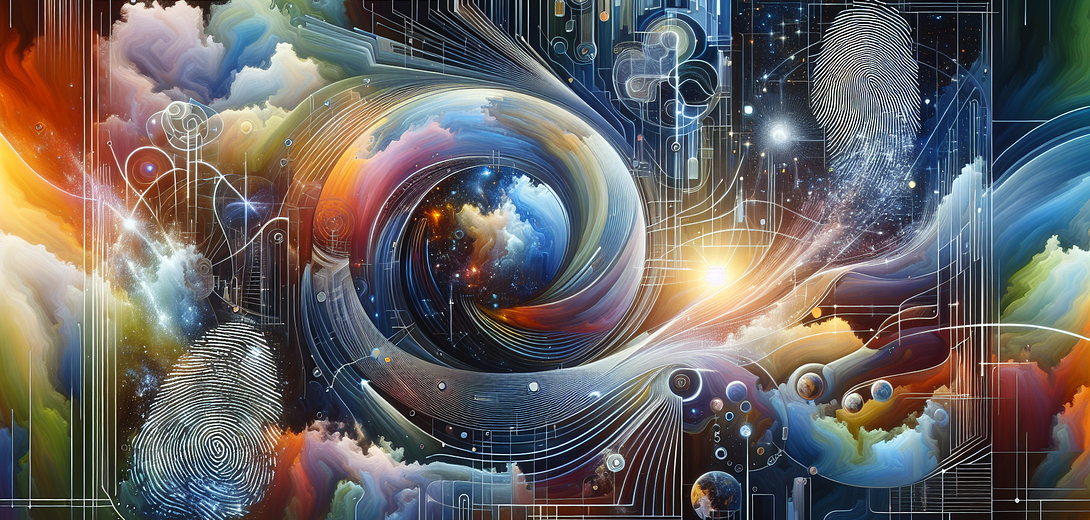
The development of microservices has revolutionized the way we build scalable and maintainable software applications. However, ensuring security within this architecture is an absolute necessity. This blog post will guide you in using the Cloving CLI tool to enhance your security practices while developing microservices in .NET. By integrating AI-driven strategies, the Cloving CLI can provide valuable insights and automate mundane tasks, allowing you to focus on the core aspects of your system’s integrity.
Setting Up Cloving for .NET Projects
Ensuring that your Cloving CLI is properly configured is the first crucial step toward developing secure microservices.
Installation
Install the Cloving CLI globally using npm:
npm install -g cloving@latest
Configuration
Configure Cloving with your API key and preferred models:
cloving config
Follow the prompt to enter your API key and choose the models that suit your project needs.
Initializing Your .NET Project
Navigate to your .NET project directory and initialize Cloving to ensure it understands your project context:
cloving init
This setup allows Cloving to analyze your project’s structure and configuration files effectively.
Generating Secure Code
One of Cloving’s unique strengths is its ability to generate code snippets that conform to security best practices. Let’s generate a secure API endpoint for user authentication in a microservice architecture.
Example: Secure Authentication Endpoint
Use the cloving generate code
command to create a secure authentication endpoint:
cloving generate code --prompt "Create a .NET Core API endpoint for user authentication with JWT" --files Controllers/AuthController.cs
This will generate a scaffold for an authentication endpoint that incorporates JWT for secure token-based authentication.
Example Code
// Controllers/AuthController.cs
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
using YourProject.Services;
using YourProject.Models;
namespace YourProject.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class AuthController : ControllerBase
{
private readonly IAuthService _authService;
public AuthController(IAuthService authService)
{
_authService = authService;
}
[HttpPost("login")]
public async Task<IActionResult> Login([FromBody] LoginRequest request)
{
var token = await _authService.Authenticate(request);
if (token == null)
return Unauthorized();
return Ok(new { Token = token });
}
}
}
Reviewing and Modifying Generated Code
Cloving provides a prompt to review, revise, or save the generated code. You can further refine the authentication logic or request explanatory comments for any complex security mechanisms.
Unit Testing for Security
Generating unit tests is essential for verifying that your security logic works as intended. Cloving can automatically create unit tests:
cloving generate unit-tests -f Controllers/AuthController.cs
This command will generate comprehensive unit tests focusing on authentication scenarios, ensuring robust handling of different edge cases.
Using Cloving Chat for Security Analysis
In complex scenarios where you need a deeper security analysis, Cloving chat can guide you through intricate security reviews.
Starting a Chat Session
Initiate Cloving chat to discuss potential vulnerabilities:
cloving chat -f Controllers/AuthController.cs
During this interactive session, you can ask Cloving for insights into potential security vulnerabilities, such as SQL injection, data exposure, or improper session handling. You can also inquire about security patches and updates.
Automating Security Reviews
Automated code reviews are a great way to maintain a security-first approach. Use Cloving’s generate review
command:
cloving generate review
This command will provide an AI-generated review that highlights security flaws or recommends improvements in your existing microservice setup.
Best Practices for Secure Microservices
- Implement Authentication and Authorization: Use JWT tokens for secure authentication and attribute-based role checks for authorization.
- Encrypt Communications: Ensure all data transmitted between microservices is encrypted, preferably with TLS.
- Limit Data Exposure: Use DTOs (Data Transfer Objects) to prevent overexposing internal data structures.
- Regular Security Audits: Use Cloving’s automated code reviews to regularly audit your codebase.
Conclusion
By using Cloving CLI, you can leverage AI to enhance security practices in your .NET microservice architecture. From generating secure code snippets to facilitating comprehensive security reviews, Cloving can be an invaluable partner in building robust, secure systems. Integrate Cloving into your daily workflow and lead the way in secure .NET development.
As always, remember that AI tools are there to augment your expertise, not replace it. Utilize Cloving to enhance your skills and ensure the security of your microservices.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.