Developing Scalable Serverless Solutions with AWS Lambda and GPT
Updated on January 02, 2025

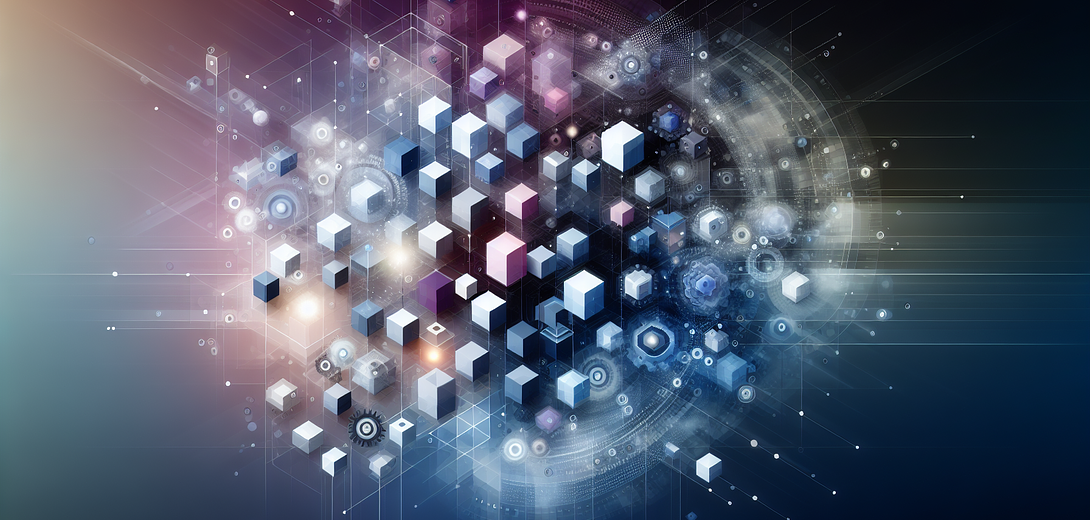
In the realm of modern software development, building scalable, serverless solutions can significantly enhance your application’s efficiency and performance. Combining AWS Lambda’s elasticity with the power of GPT models allows for creating robust and intelligent applications with ease. The Cloving CLI tool streamlines this process by integrating AI directly into your development workflow. This post will guide you through leveraging Cloving CLI to develop scalable serverless solutions on AWS Lambda using GPT models effectively.
Getting Started with Cloving CLI
Firstly, let’s set up Cloving CLI in your environment to start using it for your serverless solutions.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to use your preferred AI model:
cloving config
Follow the prompts to set up your API key, preferred models, and other configurations to suit your project needs.
Setting Up AWS Lambda with Cloving
Before diving into GPT integrations, set up your serverless function using AWS Lambda. Cloving can assist and streamline each step of the process.
Initializing Your Project
Create a new Lambda project directory and initialize Cloving:
mkdir my-lambda-project
cd my-lambda-project
cloving init
This command prepares the project directory for Cloving by creating necessary context files and configurations.
Example: Writing an AWS Lambda Function
Suppose you’re building a serverless function to handle email processing via AWS Lambda. You can make use of Cloving’s code generation capabilities to set up a basic handler function:
cloving generate code --prompt "Create an AWS Lambda handler function to process incoming emails"
Generated Code: Example Node.js AWS Lambda handler
// handler.js
exports.handler = async (event) => {
const emailContent = event.body.email;
// Process email content here
console.log('Email received:', emailContent);
return {
statusCode: 200,
body: JSON.stringify({
message: 'Email processed successfully',
}),
};
};
Integrating GPT with Lambda
To make your Lambda function smarter, integrate GPT models for tasks like language processing or data analysis. Use Cloving to streamline GPT model integration.
Adding GPT Model Integration
Let’s say you want to process and analyze the sentiment of incoming emails using a GPT model. You can initiate a Cloving chat session within your project directory to receive step-by-step code generation:
cloving chat -f handler.js
In the chat prompt, request:
Integrate GPT model to analyze the sentiment of received email content
Enhanced Code with GPT Model:
const fetch = require('node-fetch');
async function analyzeSentiment(emailContent) {
const response = await fetch('https://api.openai.com/v1/engines/gpt-3.5-turbo/completions', {
method: 'POST',
headers: {
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json',
},
body: JSON.stringify({
prompt: `Analyze the sentiment of the following email content: "${emailContent}"`,
max_tokens: 60,
}),
});
const data = await response.json();
return data.choices[0].text.trim();
}
exports.handler = async (event) => {
const emailContent = event.body.email;
const sentiment = await analyzeSentiment(emailContent);
console.log('Email sentiment:', sentiment);
return {
statusCode: 200,
body: JSON.stringify({
message: 'Email processed successfully',
sentiment,
}),
};
};
Best Practices with Cloving CLI
To harness the full capability of Cloving within serverless architectures, consider the following best practices:
-
Frequent Commits: Use
cloving commit
to regularly commit your code changes with AI-generated informative commit messages to keep track of version history. -
Code Reviews: Use the
generate review
command to conduct AI-assisted code reviews, ensuring high-quality code and adherence to best practices. -
Collaborative Sessions: When working in teams, leverage
cloving chat
for brainstorming sessions, AI-driven insights, and instant feedback. -
Cloud Deployment: During deployment, ensure your Lambda functions have proper IAM roles and policies to securely access GPT API keys and other AWS resources.
Conclusion
Leveraging Cloving CLI in your AWS Lambda development workflow enables a more productive, efficient, and intelligent approach to building serverless solutions. By integrating AI through GPT models, you can enhance functionality and provide scalable solutions to complex problems effortlessly. Embrace the integration of AWS Lambda and GPT with the support of Cloving CLI, and transform your development workflow to achieve seamless and impactful applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.