Developing High-Performance Golang Microservices with GPT Assistance
Updated on January 28, 2025

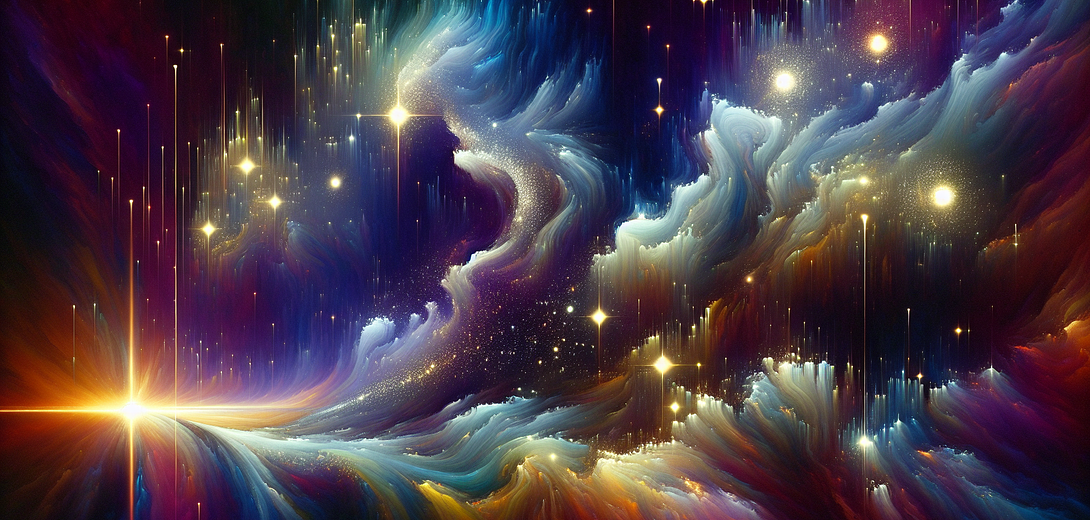
In the ever-evolving world of software development, leveraging modern tools and technologies can substantially enhance productivity and code quality. One such tool is the Cloving CLI, an AI-powered command-line interface that seamlessly integrates with your development workflow. In this post, we will explore how Cloving CLI can help you develop high-performance Golang microservices using GPT assistance to elevate your coding experience.
Understanding the Cloving CLI
The Cloving CLI is your AI-powered pair programmer for the command line. With commands to generate code, facilitate interactive chat, and tailor context-specific suggestions, Cloving can enhance your development process.
1. Setting Up Cloving
Before developing Golang microservices, ensure that Cloving is set up in your environment.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to access your preferred AI models:
cloving config
Follow the interactive prompts to set up your API key, select your models, and adjust any preferences.
2. Initializing Your Golang Project
To provide Cloving with context about your project, initialize it within your working directory:
cloving init
This command will create a cloving.json
file with metadata, enabling Cloving to understand your Golang project structure.
3. Generating Golang Code
Using the Cloving CLI, you can prompt the AI to generate Golang code that meets the needs of your microservices architecture.
Example:
Imagine you’re building a service to manage user data. Use Cloving to generate a Golang handler function for a simple REST API endpoint:
cloving generate code --prompt "Create a Golang handler to get user details from a PostgreSQL database" --files services/user_service.go
Cloving will analyze your context and produce relevant Golang code, such as:
package services
import (
"database/sql"
"encoding/json"
"net/http"
_ "github.com/lib/pq"
)
func GetUserDetailsHandler(db *sql.DB) http.HandlerFunc {
return func(w http.ResponseWriter, r *http.Request) {
userID := r.URL.Query().Get("id")
if userID == "" {
http.Error(w, "Missing user ID", http.StatusBadRequest)
return
}
var user struct {
ID string `json:"id"`
Name string `json:"name"`
// other fields
}
if err := db.QueryRow("SELECT id, name FROM users WHERE id = $1", userID).Scan(&user.ID, &user.Name); err != nil {
http.Error(w, "User not found", http.StatusNotFound)
return
}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(user)
}
}
4. Interactive Code Refinement
Once Cloving generates code, you can review and refine it interactively, ensuring it aligns with your requirements.
To further revise the handler function, initiate a Cloving chat session:
cloving chat -f services/user_service.go
Engage in a conversational flow to request tweaks, such as:
Refactor the handler to include logging and error checking
5. Generating Unit Tests
Ensuring code quality is critical, and Cloving assists in generating unit tests specific to your Golang files.
Example:
Generate unit tests for the newly created user service:
cloving generate unit-tests -f services/user_service.go
This command will yield unit tests such as:
package services_test
import (
"net/http"
"net/http/httptest"
"testing"
"database/sql"
_ "github.com/lib/pq"
"github.com/stretchr/testify/assert"
"yourpackage/services"
)
func TestGetUserDetailsHandler(t *testing.T) {
db, _ := sql.Open("postgres", "user=postgres dbname=mydb sslmode=disable")
handler := services.GetUserDetailsHandler(db)
req, _ := http.NewRequest(http.MethodGet, "/user?id=1", nil)
rr := httptest.NewRecorder()
handler.ServeHTTP(rr, req)
assert.Equal(t, http.StatusOK, rr.Code)
// add more assertions
}
6. AI-Powered Commit Messages
Cloving can enhance your commit messages by analyzing changes and generating concise summaries.
Instead of git commit
, use:
cloving commit
Cloving generates a meaningful message reflecting your code update, which you can edit or approve.
Conclusion
Developing high-performance Golang microservices with GPT assistance using the Cloving CLI facilitates a productive and efficient coding process. By harnessing AI for code generation, unit testing, and intelligent recommendations, Cloving empowers you to focus on building robust and scalable applications.
Remember, while Cloving accelerates your workflow and enhances code quality, it serves as a valuable companion to your programming expertise. Embrace Cloving to transform your development experience and achieve new heights in software craftsmanship.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.