Developing Custom Middleware Functions in Express with GPT
Updated on March 05, 2025

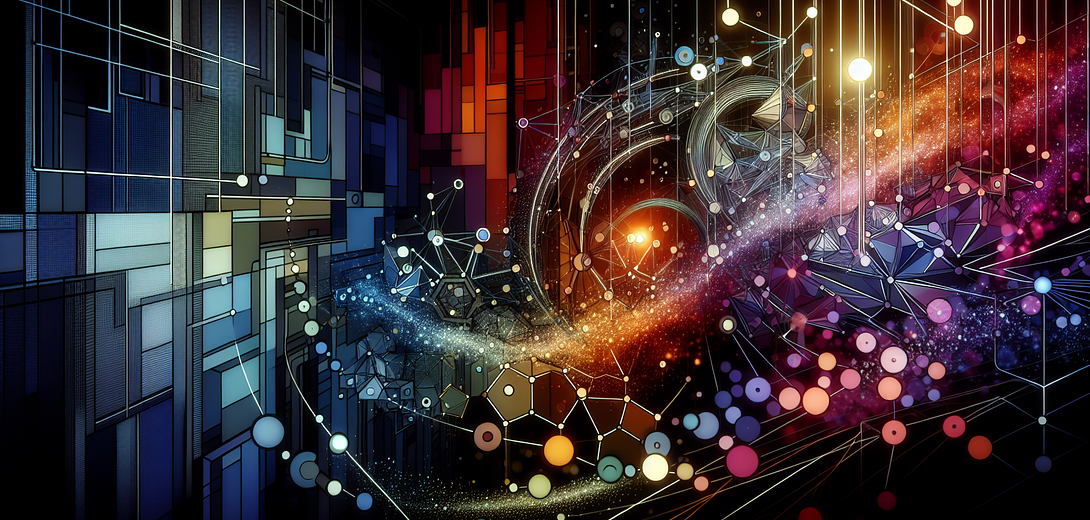
Streamlined Express Middleware Development with Cloving CLI
In the realm of Node.js, Express stands as one of the most popular frameworks for building web applications. A key advantage of Express is its middleware system, which allows you to modify request/response objects, handle authentication, log requests, and more. By pairing Express with an AI-powered tool such as Cloving CLI, you can greatly simplify and accelerate the process of writing these custom middleware functions.
In this tutorial, we’ll guide you through configuring Cloving, generating custom middleware, and integrating that middleware into your Express application. We’ll also show you how to refine and review code using Cloving’s built-in features.
1. Getting Started with Cloving CLI
1.1 Installation
First, install Cloving CLI globally with npm:
npm install -g cloving@latest
1.2 Configuration
After installation, configure Cloving with your API key and desired AI model:
cloving config
During this step, you’ll be prompted to enter your credentials and select an AI model (e.g., GPT-3.5, GPT-4).
1.3 Project Initialization
Set up Cloving within your Express project directory:
cd path/to/express/project
cloving init
This command creates a cloving.json
file that contains metadata about your project. By referencing this file, Cloving can generate more context-aware code.
2. Generating Custom Middleware with Cloving
Express middleware can handle tasks ranging from logging and authentication to rate limiting and error handling. Cloving CLI automates most of the boilerplate generation for these tasks.
2.1 Example: Request Logging Middleware
Suppose you want a request logging middleware that prints out the HTTP method and URL of incoming requests. Instead of writing it manually, let Cloving do the heavy lifting:
cloving generate code --prompt "Create an Express middleware function to log requests with method and URL" --files src/middleware/logger.js
Sample Output:
// src/middleware/logger.js
const logger = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
};
module.exports = logger;
2.2 Refining the Generated Code
Cloving typically offers a menu of options—e.g., revise, explain, or save. If you want to add certain features (like a timestamp) to the logger, simply request them:
Revise the logger middleware to prepend a timestamp to each log entry.
Cloving then modifies the middleware accordingly, inserting a timestamp in your logs.
3. Integrating Middleware into Your Express App
After generating your middleware, integrate it in your main Express file (e.g., app.js
or index.js
).
// app.js
const express = require('express');
const logger = require('./src/middleware/logger');
const app = express();
// Use the generated logger middleware
app.use(logger);
app.get('/', (req, res) => {
res.send('Hello, world!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
When you run your server, you’ll see console output for each request in the format specified by your middleware.
4. Additional Examples of Middleware Generation
Cloving CLI isn’t limited to simple request logging. Here are a few more middleware scenarios you can automate.
4.1 Authentication Middleware
To create a middleware that verifies JSON Web Tokens (JWT) before allowing access to routes:
cloving generate code --prompt "Create an Express middleware to authenticate requests using JWT" --files src/middleware/auth.js
A sample output might look like:
// src/middleware/auth.js
const jwt = require('jsonwebtoken');
const auth = (req, res, next) => {
const token = req.headers.authorization?.split(' ')[1];
if (!token) {
return res.status(401).json({ message: 'No token provided' });
}
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
req.user = decoded;
next();
} catch (err) {
res.status(401).json({ message: 'Invalid token' });
}
};
module.exports = auth;
4.2 Error-Handling Middleware
Express allows you to write specialized error-handling middleware that handles exceptions or sends custom error responses:
cloving generate code --prompt "Create an Express error-handling middleware that logs errors and responds with a JSON object" --files src/middleware/errorHandler.js
Cloving could produce:
// src/middleware/errorHandler.js
const errorHandler = (err, req, res, next) => {
console.error(err.stack);
res.status(err.statusCode || 500).json({
message: err.message || 'Internal Server Error',
});
};
module.exports = errorHandler;
5. Generating Code Reviews with Cloving
It’s best practice to review your generated code to ensure it meets your project’s needs and best practices. You can use Cloving’s review feature:
cloving generate review
This provides AI-driven insights about potential improvements, style consistency, or performance considerations in your code.
5.1 Interactive Chat for Deeper Analysis
For more in-depth inquiries, use:
cloving chat -f src/middleware/logger.js
In this interactive environment, you can ask for architectural patterns, alternative solutions, or even best practices for integration testing. Cloving’s chat-based approach mimics a real-time conversation with an AI pair programmer.
6. Tips & Best Practices
- Limit Middleware Scope: Keep each middleware function focused on a single concern (e.g., logging, auth, error handling).
- Use Environmental Variables: For tokens, database URLs, or secrets, reference them from
process.env
to keep your code secure and flexible. - Test Thoroughly: Cloving can also generate or refine unit tests for your middleware. For instance:
This helps ensure your middleware works as expected under various conditions.cloving generate unit-tests -f src/middleware/logger.js
- Combine Tools: Pair Cloving with libraries like Morgan, Helmet, or Rate Limiters to quickly produce more advanced or integrated middleware solutions.
- Iterate: If your needs change (e.g., adding IP logging or user-agent), use Cloving’s revision prompts to adapt existing middleware, maintaining a consistent code style.
7. Committing Changes with AI Assistance
Once you’re satisfied with your middleware changes, let Cloving suggest a descriptive commit message:
cloving commit
You’ll receive a commit message summarizing what’s changed, such as:
Add custom request-logging middleware and integrate it into the Express application
You can accept or edit the message before finalizing your commit, streamlining your commit history.
8. Conclusion
By leveraging Cloving CLI in your Express workflows, you can radically streamline the process of creating, refining, and reviewing custom middleware. AI-driven code generation removes the tedium of boilerplate writing while code reviews ensure your logic remains efficient, safe, and maintainable.
While Cloving provides a significant productivity boost, it’s ultimately your expertise that steers code quality and design decisions. Treat Cloving as an AI-powered partner, and you’ll find building robust Express middleware becomes simpler and more enjoyable.
Try out Cloving CLI for your next Express project, and see how quickly you can transform your middleware stack to meet evolving application demands!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.