Developing Cross-Platform Electron Apps with AI-Powered Code Generation
Updated on March 05, 2025

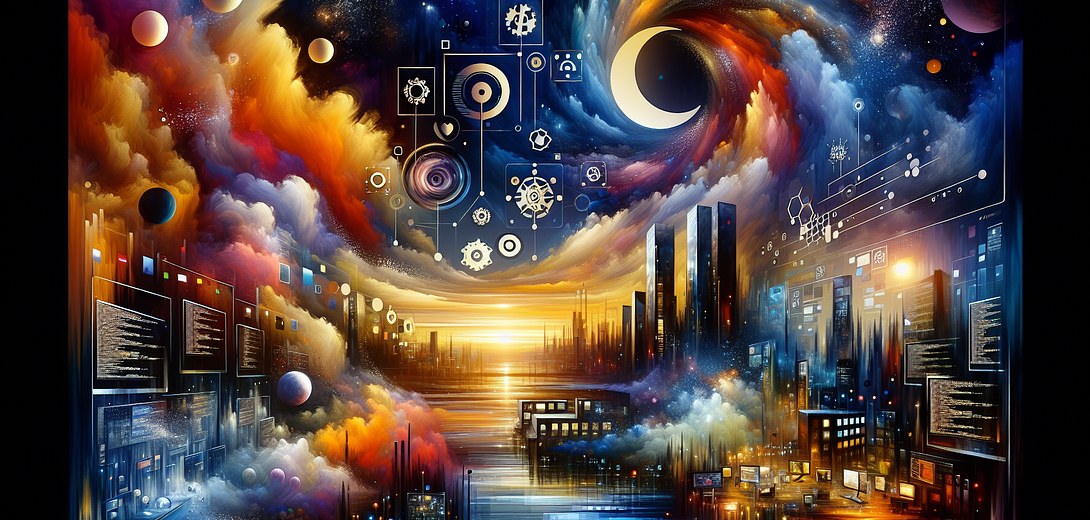
Streamlining Electron App Development with Cloving CLI
Creating cross-platform desktop applications efficiently is a challenge many developers face. With Electron, you can build desktop apps using web technologies (HTML, CSS, JavaScript), and your app will run seamlessly on Windows, macOS, and Linux. Pairing Electron with Cloving CLI – an AI-driven command-line tool – can supercharge your workflow, automate repetitive tasks, and help maintain code quality. In this tutorial, we’ll walk through how to use Cloving to generate, refine, and test code for Electron.
1. Introduction to Cloving CLI and Electron
Electron: A framework that allows you to build desktop applications with web technologies. Electron integrates Node.js and Chromium, enabling you to create native-like GUIs using familiar front-end stacks.
Cloving CLI: An AI-powered assistant that can:
- Generate boilerplate code and new features, saving time
- Automate testing by creating unit test stubs
- Perform code reviews and interactive debugging via chat
- Suggest commit messages to keep your repository clean and documented
By combining these two technologies, you harness both the flexibility of Electron and the productivity of AI-driven development.
2. Setting Up Your Environment
2.1 Prerequisites
- Node.js (latest LTS or current release)
- npm (bundled with Node.js)
2.2 Installing Cloving CLI
Install Cloving CLI globally:
npm install -g cloving@latest
2.3 Configuring Cloving
Set up Cloving with your API key and preferred AI model:
cloving config
Follow the prompts to specify your API key and select an AI model (e.g., GPT-3.5, GPT-4).
3. Initializing Your Electron Project
3.1 Create a New Electron App
Use the official Electron scaffolding tool or create-electron-app
:
npx create-electron-app my-electron-app
cd my-electron-app
This creates a basic Electron project structure in the my-electron-app
folder.
3.2 Integrate Cloving
Initialize Cloving in your Electron project:
cloving init
Cloving analyzes your directory structure and creates cloving.json
, storing metadata that helps it provide context-aware code generation.
4. Generating Code with Cloving
Once your project is set up, you can use Cloving’s code generation features to quickly add new Electron components or features.
4.1 Example: Creating a Settings Window
Suppose you need a settings window in your Electron application. Instead of manually coding everything, prompt Cloving to generate it:
cloving generate code --prompt "Create a new settings window for an Electron app" --files src/main.js
Sample Output:
// src/settingsWindow.js
const { BrowserWindow } = require('electron');
function createSettingsWindow() {
const settingsWindow = new BrowserWindow({
width: 400,
height: 300,
webPreferences: {
nodeIntegration: true
}
});
settingsWindow.loadFile('src/settings.html');
return settingsWindow;
}
module.exports = createSettingsWindow;
Notes:
- Cloving automatically chooses relevant Electron APIs (like
BrowserWindow
). - You can refine window size, position, or advanced features (e.g., security settings) by adjusting your prompt or code after generation.
4.2 Integrating the Settings Window
In your src/main.js
or equivalent main process script, import the function:
const createSettingsWindow = require('./settingsWindow');
// Example usage:
function createMainWindow() {
// ...
}
5. Interactive Development with Cloving Chat
Complex tasks often benefit from iterative coding. Cloving’s chat feature allows you to refine code in real time, ask questions, and explore alternate solutions.
5.1 Example: Adding Dark Mode Support
cloving chat -f src/main.js
In the chat prompt, you might say:
cloving> How can I add dark mode support to my Electron app?
Cloving can suggest:
- Using
nativeTheme
in Electron - Toggling styles or class names in the renderer
- Setting up an IPC channel to coordinate between the main and renderer processes
This back-and-forth approach ensures you get tailored AI help rather than generic code snippets.
6. Enhancing Code with Unit Tests
Testing is crucial for ensuring application stability. Cloving can generate unit tests for Electron modules to catch regressions or confirm new features work as intended.
6.1 Example: Testing the Settings Window
cloving generate unit-tests -f src/settingsWindow.js
Sample Output:
// test/settingsWindow.test.js
const assert = require('assert');
const { BrowserWindow } = require('electron');
const createSettingsWindow = require('../src/settingsWindow');
describe('Settings Window', () => {
it('should create a new browser window for settings', () => {
const window = createSettingsWindow();
assert.ok(window instanceof BrowserWindow);
const [width, height] = window.getSize();
assert.strictEqual(width, 400);
assert.strictEqual(height, 300);
});
});
Notes:
- Tests might need special mocks or context for Electron’s
BrowserWindow
. - Cloving can provide a starting point, and you can adapt it to your project’s test environment (e.g., Jest, Mocha, or AVA).
7. Generating Commit Messages with Cloving
Maintaining meaningful commit messages helps you and your team track changes. After staging files, let Cloving propose a commit message:
cloving commit
Cloving inspects your changes and suggests something like:
Add a new settings window for the Electron app with basic tests
You can accept or modify this before committing. This AI-driven approach ensures your Git history remains clear and descriptive.
8. Best Practices for Using Cloving with Electron
-
Provide Context
Use the--files
orcloving init
to ensure Cloving sees your relevant code structure—this yields more precise code generation. -
Iterate in Small Steps
Tackle large tasks (like theming, advanced preferences, or multi-window setups) in increments, verifying each step with Cloving’s chat or code generation prompts. -
Leverage Electron Docs
Combine Cloving with official Electron documentation to ensure best practices, such as enabling secure defaults (contextIsolation
,nodeIntegration=false
) in production. -
Secure Your App
For distribution, confirm you follow Electron’s security guidelines (disabling remote modules, checkingprocess.env.NODE_ENV
, etc.). Cloving can help set up these checks if prompted. -
Test Thoroughly
Use unit, integration, and end-to-end tests for robust coverage—Cloving can generate basic test stubs, but you’ll likely refine them further.
9. Conclusion
Incorporating Cloving CLI into your Electron development pipeline can drastically reduce boilerplate, ensure code quality, and provide an AI-assisted coding experience. From generating new windows to writing tests and commit messages, Cloving streamlines your workflow, freeing up time to focus on creative or business-critical aspects of your desktop application.
Remember: Cloving is an assistant, not a replacement. While it automates repetitive tasks, your expertise remains key to designing a secure, performant, and user-friendly Electron app. Embrace Cloving, and watch your cross-platform desktop development become faster, cleaner, and more enjoyable.
Happy coding with Cloving and Electron!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.