Designing Scalable APIs: Tips and Tricks from GPT
Updated on October 13, 2024

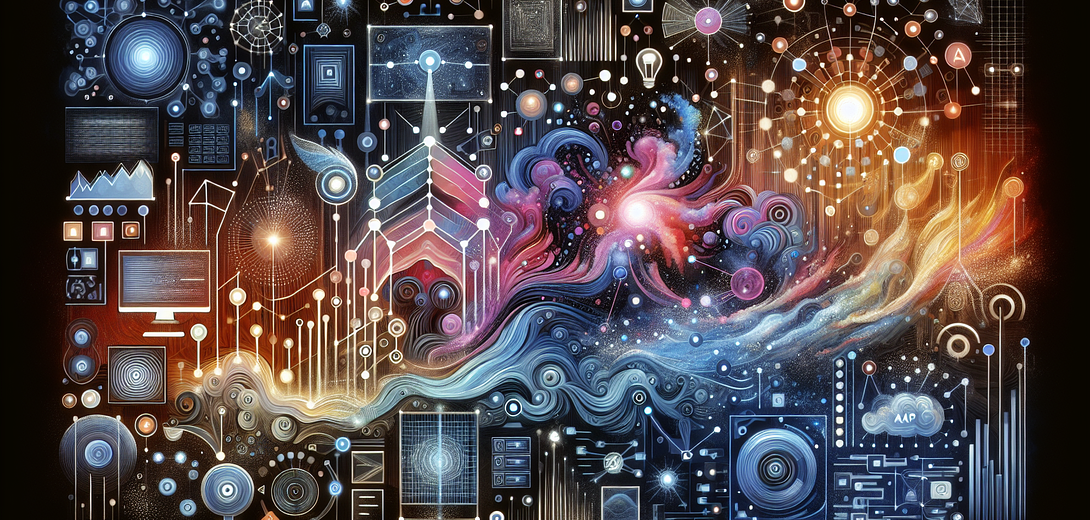
In the rapidly evolving world of web development, building scalable APIs is crucial for ensuring that your applications can handle growing user demands without compromising performance. The Cloving CLI tool, with its AI-powered capabilities, offers a robust solution for enhancing your API design and implementation workflows. In this post, we’ll explore how to leverage Cloving CLI to design scalable APIs efficiently.
Understanding the Cloving CLI
Cloving CLI is a free and open-source command-line tool that integrates AI to assist with code generation, testing, and more. It acts like an AI-enhanced pair programmer to streamline your development process.
1. Setting Up Cloving
Before diving into API design, let’s get Cloving up and running.
Installation:
Install Cloving globally via npm:
npm install -g cloving@latest
Configuration:
Configure Cloving with your chosen AI model and preferences:
cloving config
Follow the prompts to enter your AI model details and API key.
2. Initializing Your Project
For Cloving to provide optimal assistance, initialize your project:
cloving init
This creates a cloving.json
file with project-specific metadata and context.
3. Designing API Endpoints
Cloving can assist in designing clean and consistent API endpoints.
Example:
Imagine you’re designing an API for a book store. To generate an endpoint for fetching a list of books, use:
cloving generate code --prompt "Design a RESTful API endpoint to get a list of books" --files routes/books.js
Cloving will understand your project’s context and produce a well-structured endpoint. For example:
// routes/books.js
const express = require('express');
const router = express.Router();
// Mock book data
const books = [
{ id: 1, title: '1984', author: 'George Orwell' },
{ id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee' },
];
// Get a list of books
router.get('/books', (req, res) => {
res.json(books);
});
module.exports = router;
4. Generating Middleware
Middleware can be vital for tasks such as logging, authentication, and data validation. Cloving can generate middleware with ease.
Example: For generating middleware to validate incoming book data:
cloving generate code --prompt "Create middleware to validate book data" --files middleware/validateBook.js
The result could be:
// middleware/validateBook.js
function validateBook(req, res, next) {
const { title, author } = req.body;
if (!title || !author) {
return res.status(400).json({ error: 'Title and author are required' });
}
next();
}
module.exports = validateBook;
5. Testing Your API
To ensure your API is scalable and reliable, it’s essential to have robust testing. Cloving can help generate unit and integration tests.
Example: To generate tests for your book endpoints:
cloving generate unit-tests -f routes/books.js
This command will craft unit tests tailored to your endpoints:
// tests/routes/books.test.js
const request = require('supertest');
const app = require('../../app');
describe('GET /books', () => {
it('should return a list of books', async () => {
const res = await request(app).get('/books');
expect(res.statusCode).toEqual(200);
expect(res.body).toHaveLength(2);
});
});
6. Using Cloving Chat for Strategy and Guidance
For more sophisticated design strategies or troubleshooting, leverage Cloving’s chat tool:
$ cloving chat
Engage with it to refine your API design, optimize performance, or seek advice on best practices.
7. Implementing Caching and Rate Limiting
To enhance scalability, consider implementing caching and rate-limiting strategies.
Example: For generating a caching middleware:
cloving generate code --prompt "Create a caching middleware for book requests" --files middleware/cache.js
8. Deployment and Continuous Integration
Deploy your API while ensuring continuous integration and deployment with automated scripts. Use Cloving to generate shell scripts:
cloving generate shell --prompt "Create a deployment script for Node.js API"
Conclusion
Designing scalable APIs requires a balance between robust architecture and efficient tools. By harnessing the AI capabilities of Cloving CLI, you can streamline your development process, ensuring high-quality, scalable API solutions. Implement these techniques into your workflow and watch your productivity soar.
Explore Cloving CLI and redefine your API design experience with AI-powered assistance, ensuring your applications are ready to scale seamlessly.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.