Designing Modular Code Architecture in Rust with AI Guidance
Updated on January 28, 2025

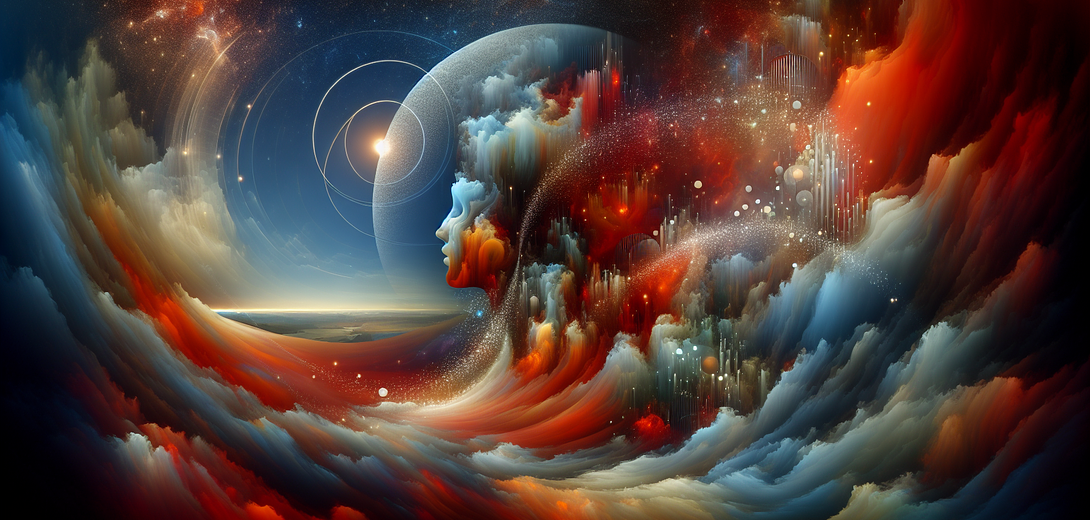
In software development, modular code architecture ensures maintainable, scalable, and efficient codebases. Rust, renowned for its safety and concurrency, is perfect for projects where performance and reliability are vital. However, designing a modular code architecture in Rust can be challenging, especially for complex applications. Enter Cloving CLI—a tool that integrates AI into your development workflow, assisting you in creating well-structured Rust projects. This post explores how to leverage Cloving to design modular architectures in Rust.
1. Setting Up Cloving for Your Rust Project
Before delving into modular design with Cloving, ensure the tool is set up correctly in your development environment.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to utilize your preferred AI model:
cloving config
Follow prompts to set your API key, preferred AI model, and other configurations.
2. Initializing Your Rust Project with Cloving
Cloving must understand your project to offer relevant suggestions. Initialize Cloving in your Rust project’s directory:
cloving init
This command analyzes your project and sets up necessary metadata to aid in code generation and suggestions.
3. Designing a Modular Architecture
Step 1: Defining Modules
Assume you’re starting a Rust project for a web service. You want to design modules such as authentication, database, API handlers, and logging.
Example:
To define a Rust module for user authentication and structure its components, use:
cloving generate code --prompt "Define a module for user authentication, including functions for login and logout" --files src/auth.rs
Generated Code Example:
pub mod auth {
pub fn login(username: &str, password: &str) -> Result<String, String> {
// Implement login logic here
if username == "user" && password == "pass" {
Ok("Login successful".to_string())
} else {
Err("Invalid credentials".to_string())
}
}
pub fn logout(user_id: u32) -> Result<(), String> {
// Implement logout logic here
Ok(())
}
}
This creates a basic authentication module with functions login
and logout
.
Step 2: Integrating Modules
Use Cloving to integrate these modules cohesively within your application architecture. Utilize AI guidance to interconnect modules, ensuring efficient data flow and function calls.
cloving generate code --prompt "Integrate auth module with database for user data validation" --files src/auth.rs, src/db.rs
Step 3: Adding Unit Tests
Testing is essential. Use Cloving to automatically generate unit tests for the defined modules, ensuring robustness.
cloving generate unit-tests -f src/auth.rs
Generated Tests Example:
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_login_success() {
assert_eq!(auth::login("user", "pass"), Ok("Login successful".to_string()));
}
#[test]
fn test_login_failure() {
assert_eq!(auth::login("wrong_user", "wrong_pass"), Err("Invalid credentials".to_string()));
}
}
4. Using Cloving Chat for Real-time Assistance
For ongoing guidance, use Cloving’s chat feature. It provides an interactive session to ask questions or seek code improvements.
$ cloving chat -f src/auth.rs
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
cloving> Improve the login function to use hashed passwords
Certainly! Here's an updated implementation:
...
This session guides you through refining and optimizing your code.
5. Commit with Contextual Messages
After making modifications, use Cloving to create meaningful commit messages:
cloving commit
This examines your changes and suggests contextual commit messages, aligning with your module updates and enhancements. Here are examples of potential commit messages that GPT might generate for a project like this:
- “Add initial user authentication module with login/logout functionality”
- “Integrate authentication module with database module for user validation”
- “Generate automatic unit tests for authentication model”
- “Enhance login logic to use secure password hashing”
- “Refactor auth and db modules for improved modularity and data flow”
Conclusion
Designing modular code architecture in Rust is streamlined with Cloving’s AI capabilities. By leveraging Cloving, you can design, implement, and integrate modules effectively, enhancing productivity and ensuring a maintainable codebase. Rust projects benefit significantly from such guidance, enabling developers to focus on core functionalities while Cloving handles auxiliary tasks.
Embrace Cloving in your Rust workflow to improve code quality, structure, and efficiency—making your modular architecture a breeze to manage.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.