Designing Interactive Frontend Components with AI and GPT for Vue.js
Updated on January 20, 2025

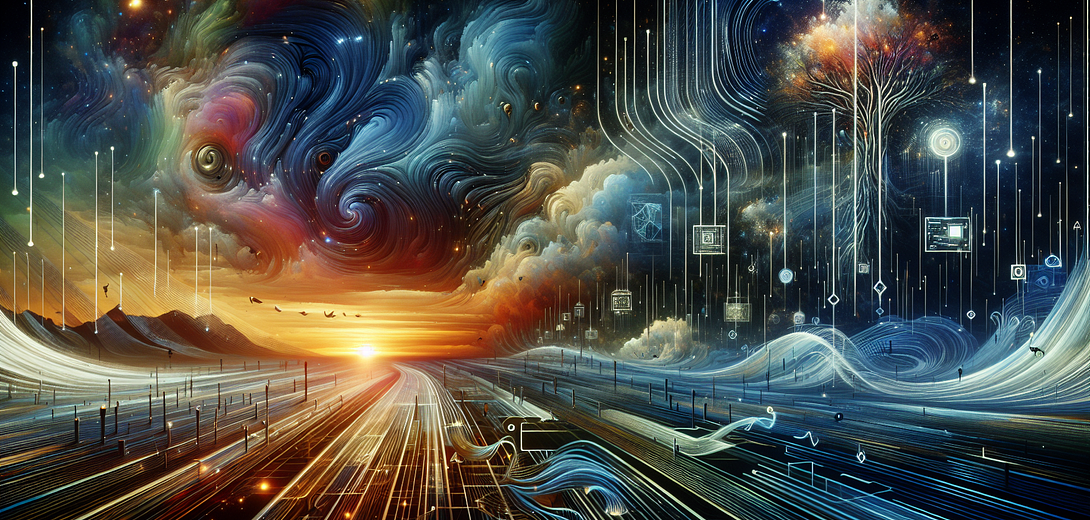
In the rapidly evolving world of frontend development, designing interactive components efficiently is paramount. Enter Cloving CLI: an AI-powered command-line interface tool that helps developers supercharge their productivity by integrating GPT-driven assistance directly into their workflows. This tutorial will guide you on using Cloving CLI to design interactive Vue.js components effortlessly.
Getting Started with Cloving CLI
Installation
Begin by installing Cloving CLI globally on your system:
npm install -g cloving@latest
Configuration
Once installed, configure Cloving to work with your preferred AI models and API key:
cloving config
Follow the prompts to set up your AI model and API key. This ensures Cloving generates contextually relevant code suggestions for your project.
Initializing Your Vue.js Project
To benefit from Cloving’s AI insights, initialize your Vue.js project with Cloving:
cloving init
This command will analyze your project settings and create a cloving.json
file. This file captures essential metadata, allowing Cloving to tailor its suggestions appropriately.
Generating Vue.js Components with Cloving
Let’s say you want to create a Vue.js component for an interactive user profile card. You can rely on Cloving to assist with this task:
Generating the Component
Invoke Cloving’s code generation feature:
cloving generate code --prompt "Create a Vue.js component for an interactive user profile card" --files src/components/UserProfile.vue
This command guides Cloving to analyze your project context and draft a component suited to your needs. An example output might resemble the following:
<template>
<div class="user-profile">
<img :src="user.image" alt="Profile Picture">
<h2>{{ user.name }}</h2>
<p>{{ user.bio }}</p>
<button @click="toggleFollow">{{ isFollowing ? 'Unfollow' : 'Follow' }}</button>
</div>
</template>
<script>
export default {
name: 'UserProfile',
props: {
user: {
type: Object,
required: true
}
},
data() {
return {
isFollowing: false
};
},
methods: {
toggleFollow() {
this.isFollowing = !this.isFollowing;
}
}
};
</script>
<style scoped>
.user-profile {
border: 1px solid #ccc;
padding: 20px;
display: flex;
flex-direction: column;
align-items: center;
}
</style>
Improving and Refining
Once the component is generated, take advantage of Cloving’s interactive refinement feature:
cloving generate code --interactive
This launches an interactive session where you can suggest enhancements, such as adding animations or adjusting styling. Cloving will provide revised code based on your feedback.
Ensuring Quality with Unit Tests
Cloving can also help you establish robust unit tests to ensure your component functions as intended:
cloving generate unit-tests -f src/components/UserProfile.vue
This command automates the creation of unit tests, contributing to higher code reliability. You might get something like:
import { mount } from '@vue/test-utils';
import UserProfile from './UserProfile.vue';
describe('UserProfile.vue', () => {
it('renders user name and bio', () => {
const user = { name: 'Jane Doe', bio: 'Software Developer' };
const wrapper = mount(UserProfile, {
propsData: { user }
});
expect(wrapper.text()).toContain('Jane Doe');
expect(wrapper.text()).toContain('Software Developer');
});
it('toggles follow state on button click', async () => {
const user = { name: 'Jane Doe', bio: 'Software Developer' };
const wrapper = mount(UserProfile, {
propsData: { user }
});
const button = wrapper.find('button');
await button.trigger('click');
expect(wrapper.text()).toContain('Unfollow');
await button.trigger('click');
expect(wrapper.text()).toContain('Follow');
});
});
Discussing and Iterating with Cloving Chat
For detailed clarifications or brainstorming coding strategies, engage in an interactive chat with Cloving:
cloving chat -f src/components/UserProfile.vue
This session allows you to communicate directly with your AI pair programmer, offering suggestions, advice, or explanations on complex coding queries.
Crafting Meaningful Commit Messages
When you’re ready to commit your changes, employ Cloving to generate insightful commit messages:
cloving commit
This utility ensures your commit messages accurately reflect the changes made, improving collaboration and version control.
Conclusion
By integrating Cloving CLI into your Vue.js development workflow, you harness the power of AI to design interactive frontend components with increased efficiency and precision. Cloving enhances your coding experience, from component generation to ongoing revisions and testing. Embrace this AI-powered tool to elevate your development process to new heights.
Remember, while Cloving serves as an intelligent assistant, your creative input and engineering skills remain at the core of producing extraordinary software. Dive in, explore Cloving’s capabilities, and redefine how you approach frontend component development.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.