Debugging Real-time Applications with WebSockets Using GPT
Updated on June 27, 2024

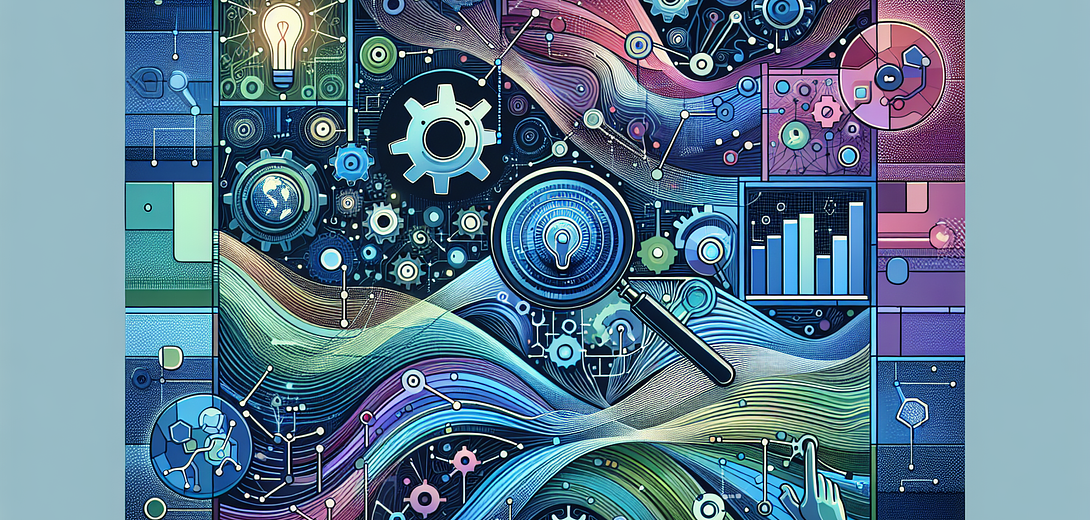
Debugging WebSocket-based applications can be a challenging and intricate task.
By leveraging the principles of cloving—harnessing the combined power of human creativity and the analytical prowess of artificial intelligence—programmers can streamline their workflows using AI tools like GPT.
In this post, we’ll dive into how you can incorporate GPT into your daily workflows to become more efficient and effective at debugging real-time applications using WebSockets.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical capabilities to solve problems more effectively. The goal is to create a collaborative relationship where humans and machines leverage each other’s strengths.
1. Diagnosing Connection Issues
AI can help identify and diagnose connection issues with WebSockets, providing suggestions and insights that might not be immediately apparent.
Example:
Suppose your WebSocket connection is dropping unexpectedly. You can describe the issue to GPT to get insights:
I have a WebSocket connection in my JavaScript app that drops unexpectedly after a few minutes. Here is a code snippet: [code snippet]. How can I fix this?
GPT may suggest checking for network stability, server-side configurations, or ensuring the WebSocket connection is being properly managed with heartbeats or reconnection logic.
const socket = new WebSocket('ws://example.com/socketserver');
socket.onopen = function(event) {
console.log('Connection established', event);
// Implementing a heartbeat to keep the connection alive
setInterval(() => {
if (socket.readyState === WebSocket.OPEN) {
socket.send(JSON.stringify({ type: 'ping' }));
}
}, 30000);
};
socket.onmessage = function(event) {
console.log('Message received', event.data);
// Handle incoming messages
};
socket.onerror = function(error) {
console.error('WebSocket Error:', error);
};
socket.onclose = function(event) {
console.log('Connection closed', event);
// Implementing reconnection logic
setTimeout(() => {
socket = new WebSocket('ws://example.com/socketserver');
}, 5000);
};
2. Analyzing Real-Time Data Flow
Debugging real-time data flow can be complex due to the asynchronous nature of WebSockets. GPT can help you trace and analyze the data flow to pinpoint issues.
Example:
If you suspect that messages are not being handled correctly, you can ask GPT for assistance in tracing the flow of data:
How can I implement a mechanism to trace real-time data flow in my WebSocket connection to ensure all messages are being handled properly? Here is my current implementation: [code snippet].
GPT may suggest adding extensive logging or creating a debugging middleware to trace messages.
const socket = new WebSocket('ws://example.com/socketserver');
socket.onmessage = function(event) {
console.log('Message received:', event.data); // Log all incoming messages
try {
const message = JSON.parse(event.data); // Assuming JSON-formatted messages
// Process message based on type
switch (message.type) {
case 'update':
console.log('Update received:', message.data);
break;
case 'notification':
console.log('Notification received:', message.data);
break;
default:
console.log('Unknown message type:', message.type);
}
} catch (error) {
console.error('Error processing message:', error);
}
};
3. Generating Test Scenarios
Writing comprehensive test scenarios for WebSocket interactions can be tedious. GPT can help you generate relevant test cases to ensure robust testing.
Example:
If you’ve implemented a new feature and need to test it, you can prompt GPT:
Generate test scenarios for a WebSocket chat application to ensure messages are delivered and displayed in real-time.
GPT will provide you with scenarios that cover various aspects of the feature:
1. Check if connection is properly established and maintained.
2. Test message sending and receiving under normal conditions.
3. Simulate network interruptions and test reconnection logic.
4. Validate message order and timestamp accuracy.
5. Test handling and display of different message types (text, image, file).
6. Verify security features such as authentication and message encryption.
4. Optimizing Performance
Performance is critical for real-time applications. GPT can help you identify potential performance bottlenecks and suggest optimizations.
Example:
If your WebSocket server is experiencing high latency, you can ask GPT for performance optimization tips:
What are best practices for optimizing the performance of a WebSocket server handling high-frequency real-time data?
GPT will provide useful recommendations, such as:
- Load balancing WebSocket connections across multiple servers.
- Implementing message compression to reduce payload size.
- Using efficient data structures and algorithms to process messages.
- Regularly profiling and monitoring server performance for bottlenecks.
5. Documentation and Explanation
Complex WebSocket implementations require clear documentation. GPT can assist in generating documentation that explains your code and architecture.
Example:
If you need to document a WebSocket-based real-time chat server, you can request:
Generate documentation for this real-time WebSocket chat server implementation: [code snippet].
GPT will create comprehensive documentation, helping you and your team understand and maintain the codebase.
## Real-Time WebSocket Chat Server
### Overview
This server facilitates real-time communication between clients using WebSocket technology.
### Features
- Establishes WebSocket connections with clients.
- Handles real-time message sending and receiving.
- Supports message types: text, image, file.
- Includes reconnection logic for network interruptions.
- Implements security measures for authentication and encryption.
### Code Breakdown
1. Server Initialization
2. Connection Management
3. Message Handling
4. Reconnection and Error Handling
5. Performance Optimizations
### Usage
To run the server:
```sh
node websocket-chat-server.js
Example Client Implementation
const socket = new WebSocket('ws://example.com/chatserver');
// Event listeners (similar to those provided earlier)
Conclusion
Debugging real-time applications with WebSockets using AI tools like GPT exemplifies the power of cloving—integrating human intuition and creativity with AI’s analytical capabilities. Incorporating GPT into your debugging workflow can enhance your productivity, reduce errors, and ensure robust performance and best practices.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.