Debugging CORS Policy Errors in Ruby on Rails With GPT
Updated on June 26, 2024

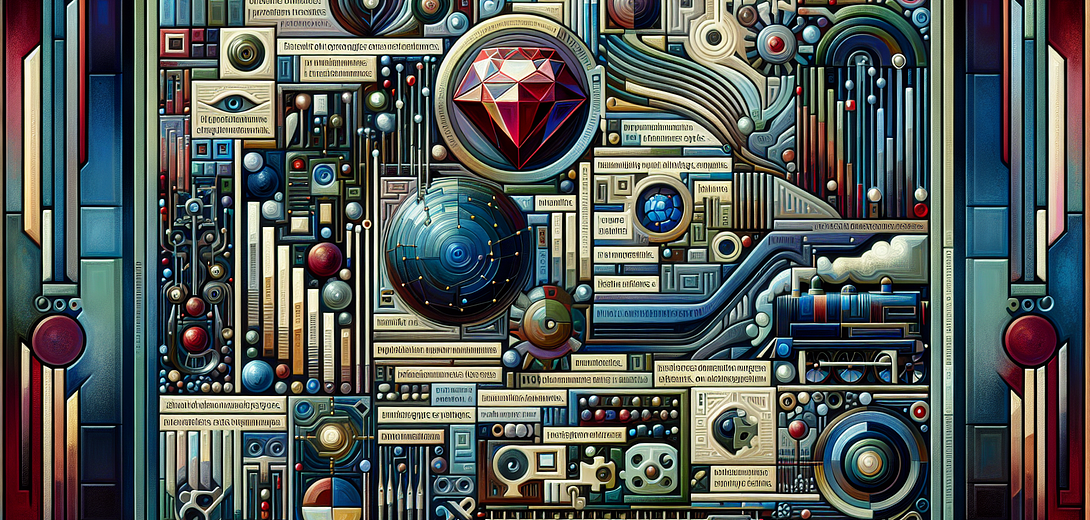
Cloving combines AI’s analytical power with human creativity and problem-solving skills to achieve better and faster results. In the context of debugging, this means using AI to assist in identifying, diagnosing, and resolving issues while taking advantage of one’s own understanding and intuition.
1. Identifying CORS Errors
Before diving into debugging, it’s essential to identify the exact nature of the CORS error. You can describe the problem to GPT to get a better understanding of what’s going wrong.
Example:
Suppose you’re receiving a CORS policy error while making an AJAX request to your Rails backend. You can prompt GPT:
I'm getting a CORS policy error when making an AJAX request to my Ruby on Rails API. The error message says, 'Access to XMLHttpRequest has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.' Here's my Rails server configuration: [code snippet].
GPT will analyze your configuration and may suggest potential causes and initial steps to investigate, such as checking your CORS middleware settings.
2. Configuring CORS Middleware
GPT can help you correctly configure the CORS middleware in your Rails application. This can be particularly helpful if you’re not familiar with the finer details of setting up the CORS policy.
Example:
You can ask GPT for the proper way to configure CORS in a Rails application:
How do I configure CORS in a Ruby on Rails application to allow requests from specific origins?
GPT might provide you with a snippet like this:
# config/initializers/cors.rb
Rails.application.config.middleware.insert_before 0, Rack::Cors do
allow do
origins 'http://example.com' # Replace this with your allowed origins
resource '*',
headers: :any,
methods: [:get, :post, :put, :patch, :delete, :options, :head]
end
end
3. Diagnosing Issues with Headers
CORS issues often stem from improper headers being sent or received. You can use GPT to generate code that inspects and verifies the headers in your Rails application.
Example:
You might ask GPT to help you check the headers being sent in your Rails response:
How can I inspect the headers in my Rails response to ensure the 'Access-Control-Allow-Origin' is correctly set?
GPT may suggest:
class ApplicationController < ActionController::Base
before_action :set_cors_headers
private
def set_cors_headers
headers['Access-Control-Allow-Origin'] = 'http://example.com' # Adjust as needed
end
end
4. Generating Comprehensive Test Cases
Testing is essential to ensure that your CORS configuration works across different scenarios. GPT can help automate the generation of test cases to cover various situations.
Example:
If you need to create tests for your CORS configuration, you can ask GPT:
Generate RSpec tests to verify the CORS headers in my Rails application.
GPT may provide a set of tests like this:
# spec/requests/cors_spec.rb
require 'rails_helper'
RSpec.describe 'CORS', type: :request do
describe 'OPTIONS /api/resource' do
it 'returns the correct CORS headers' do
options '/api/resource', headers: { 'Origin' => 'http://example.com' }
expect(response.headers['Access-Control-Allow-Origin']).to eq('http://example.com')
expect(response.headers['Access-Control-Allow-Methods']).to include('GET', 'POST', 'PUT', 'DELETE', 'OPTIONS')
end
end
end
5. Providing Detailed Documentation and Explanation
Clear documentation is crucial for maintaining and understanding your CORS configuration. GPT can assist in generating detailed documentation for your setup.
Example:
When you need to document your CORS configuration, you can ask GPT:
Generate documentation for the CORS configuration in my Ruby on Rails application.
GPT might generate something like this:
# CORS Configuration
The CORS configuration is set up in `config/initializers/cors.rb`. It allows requests from specific origins to access the API resources.
### Example Configuration
```ruby
Rails.application.config.middleware.insert_before 0, Rack::Cors do
allow do
origins 'http://example.com'
resource '*',
headers: :any,
methods: [:get, :post, :put, :patch, :delete, :options, :head]
end
end
Testing CORS Headers
RSpec tests are located in spec/requests/cors_spec.rb
to verify that the correct headers are returned for OPTIONS requests.
RSpec.describe 'CORS', type: :request do
describe 'OPTIONS /api/resource' do
it 'returns the correct CORS headers' do
options '/api/resource', headers: { 'Origin' => 'http://example.com' }
expect(response.headers['Access-Control-Allow-Origin']).to eq('http://example.com')
expect(response.headers['Access-Control-Allow-Methods']). to include('GET', 'POST', 'PUT', 'DELETE', 'OPTIONS')
end
end
end
Conclusion
Debugging CORS policy errors in Ruby on Rails can be a daunting task, but by leveraging the power of cloving—combining human intuition with the analytical capabilities of AI like GPT—you can streamline and improve your workflow. Whether it’s identifying issues, configuring middleware, checking headers, generating tests, or documenting your setup, GPT can assist at every step, enhancing your productivity and effectiveness. Embrace cloving to transform your programming experience and deliver robust, error-free applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.