Debugging Asynchronous Code in JavaScript with GPT
Updated on June 27, 2024

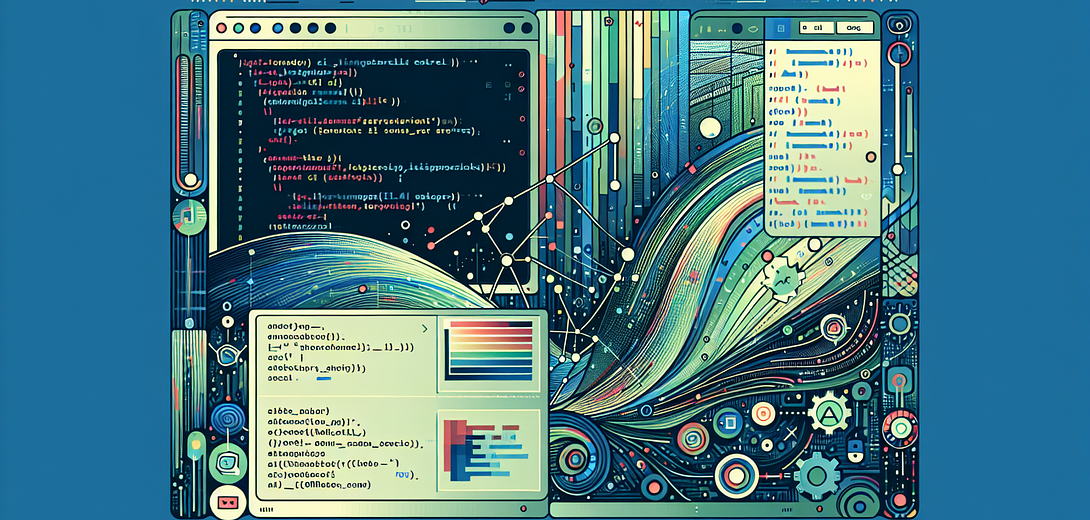
Debugging asynchronous code can sometimes be tricky due to its non-linear execution flow.
In this blog post, we will explore how AI tools like GPT can enhance your debugging experience with asynchronous code in JavaScript.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical capabilities to solve problems more effectively. It’s about creating a synergistic relationship where both human and machine strengths are utilized to achieve common goals more quickly and efficiently.
1. Automated Bug Detection
AI can help detect bugs in your asynchronous JavaScript code that might not be immediately obvious. By analyzing your code, GPT can point out potential issues and suggest fixes.
Example:
Suppose you are working with a promise chain, and you notice that a certain task is not getting executed as expected. You can describe the issue to GPT:
I have a promise chain in my JavaScript code, but the final `then` block is not being executed. How can I fix this?
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
processData(data);
})
.then(() => {
console.log('Process complete');
})
.catch(error => {
console.error('An error occurred:', error);
});
GPT might suggest checking if processData
throws an error or if there’s an issue with the HTTP request that could be causing the promise chain to break.
2. Code Completion and Suggestions
GPT can assist in writing efficient asynchronous JavaScript code by providing code completions and suggestions. This is particularly useful when dealing with complex callback structures.
Example:
If you need to perform multiple asynchronous operations in sequence and handle their results, you can ask GPT for assistance:
Write a function that fetches user data, processes it, and then fetches the user's related posts, all using async/await.
GPT will generate the following:
async function getUserAndPosts(userId) {
try {
const userData = await fetch(`https://api.example.com/users/${userId}`).then(res => res.json());
const processedData = processData(userData);
const userPosts = await fetch(`https://api.example.com/users/${userId}/posts`).then(res => res.json());
return {
userData: processedData,
posts: userPosts
};
} catch (error) {
console.error('An error occurred:', error);
}
}
3. Learning and Adapting Best Practices
Staying updated with best practices for writing asynchronous JavaScript can be time-consuming. GPT can be a useful guide in offering insights and explanations.
Example:
To learn about best practices for handling promises and asynchronous operations, you can ask GPT:
What are the best practices for handling promises and async/await in JavaScript in 2024?
GPT will provide a list of tips, such as always handling promise rejections, avoiding nested promises by using async/await
, and using tools like Promise.all
for parallel execution.
4. Generating Test Cases
Writing test cases for asynchronous code can be complex. GPT can help automate this by generating appropriate test cases.
Example:
If you need to write a test for an asynchronous function, you can prompt GPT:
Generate Mocha/Chai test cases for this asynchronous JavaScript function: [code snippet].
Code Snippet:
async function fetchData(url) {
const response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
return await response.json();
}
GPT will generate:
const { expect } = require('chai');
const sinon = require('sinon');
const fetch = require('node-fetch');
describe('fetchData', () => {
it('should fetch data successfully', async () => {
const mockResponse = { data: 'test' };
sinon.stub(fetch, 'Promise').returns(Promise.resolve({
ok: true,
json: () => Promise.resolve(mockResponse)
}));
const result = await fetchData('https://api.example.com');
expect(result).to.eql(mockResponse);
fetch.Promise.restore();
});
it('should throw an error if fetch fails', async () => {
sinon.stub(fetch, 'Promise').returns(Promise.resolve({
ok: false
}));
try {
await fetchData('https://api.example.com');
} catch (error) {
expect(error.message).to.equal('Network response was not ok');
}
fetch.Promise.restore();
});
});
5. Documentation and Explanation
Understanding and documenting asynchronous code can be challenging. GPT can help by generating clear explanations and documentation for your code.
Example:
When you need to document a complex asynchronous function, you can ask GPT:
Generate documentation for this asynchronous JavaScript function: [code snippet].
Code Snippet:
async function fetchWeatherData(city) {
const response = await fetch(`https://api.weather.com/v3/wx/forecast/${city}`);
if (!response.ok) {
throw new Error('Failed to fetch weather data');
}
const data = await response.json();
return data;
}
GPT will create detailed documentation:
/**
* Fetches weather data for a specified city.
*
* @param {string} city - The name of the city for which to fetch weather data.
* @returns {Promise<Object>} - A promise that resolves to the weather data.
* @throws {Error} - If the network request fails.
*/
async function fetchWeatherData(city) {
const response = await fetch(`https://api.weather.com/v3/wx/forecast/${city}`);
if (!response.ok) {
throw new Error('Failed to fetch weather data');
}
const data = await response.json();
return data;
}
Conclusion
Debugging asynchronous code in JavaScript can be significantly enhanced by integrating GPT into your workflow. By leveraging cloving, you can utilize AI’s analytical capabilities to complement your human creativity and intuition. This symbiotic relationship can help you detect bugs, write efficient code, stay updated with best practices, generate test cases, and create clear documentation, ultimately improving your productivity and effectiveness.
Bonus Follow-Up Prompts
To further enhance your workflow, here are a few bonus prompts you could use with GPT:
How can I optimize the performance of my asynchronous JavaScript code?
And another:
Generate utility functions for handling common asynchronous patterns in JavaScript.
And one more:
What are the latest JavaScript libraries for managing asynchronous code in 2024?
By embracing cloving, you can transform how you approach asynchronous programming and debugging in JavaScript, achieving a new level of efficiency and effectiveness.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.