Creating Scalable WebSocket Servers in Node.js Using GPT
Updated on March 05, 2025

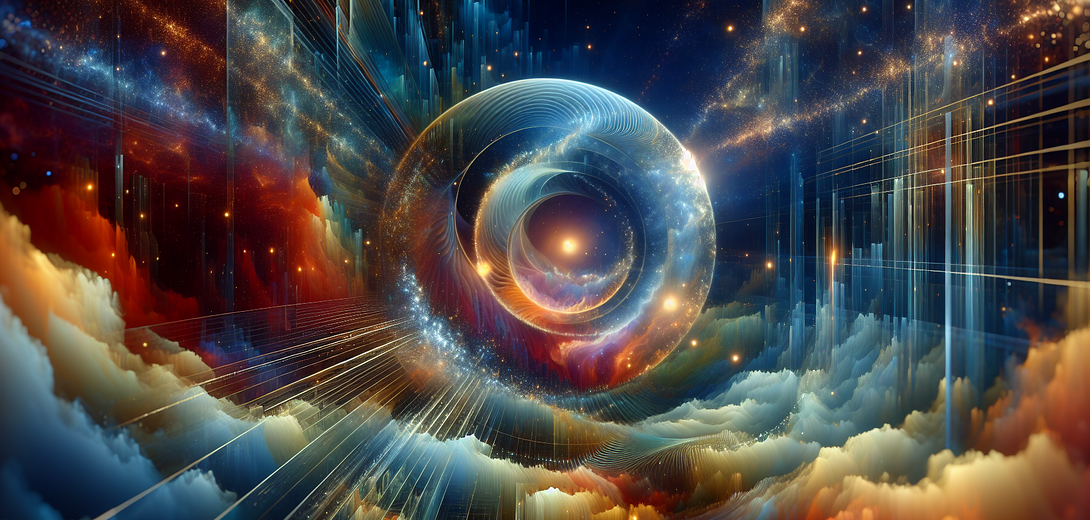
Building Scalable WebSocket Servers with Cloving CLI in Node.js
As web applications become increasingly real-time, developers need tools that make it easier to implement scalable WebSocket servers. With Cloving CLI, an AI-powered command-line interface, you can integrate AI capabilities into your workflow to boost productivity and elevate code quality. This post explores how you can leverage Cloving CLI—particularly its code generation and interactive chat features—to efficiently build scalable WebSocket servers in Node.js.
1. Overview of Cloving CLI
Cloving CLI is designed for developers aiming to accelerate their coding pipeline with AI assistance. It provides:
- Code Generation: Prompt-based creation of boilerplate or feature-specific scripts.
- Interactive Chat: Real-time AI assistance for debugging, refactoring, or brainstorming improvements.
- Customization: Configuration options for AI models, enabling you to tailor outputs to your project’s needs.
2. Preparation and Configuration
Before building your server, ensure your environment is prepared for Cloving.
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
Configure Cloving with your preferred AI model and API key:
cloving config
Follow the interactive prompts to select models and input your API key. Be sure to store your API credentials securely.
2.3 Initializing Your Project
Initiate Cloving within your project’s directory to set the stage for efficient code generation:
cloving init
This generates a cloving.json
file containing metadata about your project and configurations, helping Cloving understand your project’s context more effectively.
3. Generating a Scalable WebSocket Server
With Cloving set up, you can start by creating a basic WebSocket server using Node.js. Though we’ll highlight the ws library in our example, you can choose any library or framework.
3.1 Basic Boilerplate Generation
Example Command
cloving generate code --prompt "Generate a scalable WebSocket server in Node.js using the ws library." --files server.js
By providing a descriptive prompt, you guide Cloving to create relevant, contextual code. Below is an example output:
// server.js
const WebSocket = require('ws');
// Initialize a WebSocket server
const wss = new WebSocket.Server({ port: 8080 });
// Set up connection listener
wss.on('connection', (ws) => {
console.log('New client connected');
// Send a welcome message
ws.send('Welcome to the WebSocket server');
// Handle messages from clients
ws.on('message', (message) => {
console.log(`Received: ${message}`);
// Broadcast the message to all connected clients
wss.clients.forEach((client) => {
if (client.readyState === WebSocket.OPEN) {
client.send(`Echo: ${message}`);
}
});
});
// Handle client disconnection
ws.on('close', () => {
console.log('Client disconnected');
});
});
console.log('WebSocket server is running on ws://localhost:8080');
You now have a functional WebSocket server running on port 8080.
4. Interactive Code Revision and Customization
One of the standout features of Cloving CLI is the interactive chat, allowing you to refine and customize the server based on evolving requirements.
4.1 Using the Chat Feature
cloving chat -f server.js
Within this session, you can request modifications, such as:
Revise the server to include connection throttling and rate-limited message broadcasts to prevent overload.
Cloving might respond by adding rate limiting for broadcasts or discussing potential concurrency strategies (e.g., using worker threads or third-party libraries like bottleneck).
4.2 Scaling Beyond a Single Instance
Node.js can handle a high volume of connections, but you may need to scale further:
- Cluster Mode: Deploy multiple instances of your Node.js server on different CPU cores, sharing a load balancer in front.
- Docker/Kubernetes: Containerize the application and scale horizontally.
- Redis/MQTT: Offload certain real-time messaging tasks to specialized brokers for multi-instance communication.
You can instruct Cloving to refactor your code for these setups using the chat feature or by providing additional prompts. For instance:
cloving chat -f server.js
Refactor this server to use Redis for pub/sub broadcasting among multiple Node.js instances.
Cloving can then generate or adapt your code to integrate Redis, ensuring messages are broadcast to all servers in your cluster.
5. Enhancing Your Server with Unit Tests
A robust codebase needs tests. Cloving CLI’s AI-driven test generation can kickstart your test suite.
5.1 Generating Tests
cloving generate unit-tests -f server.js
Cloving will produce scaffolding for your tests, such as:
// server.test.js
const WebSocket = require('ws');
const { createServer } = require('http');
const { expect } = require('chai');
describe('WebSocket Server', () => {
let server;
let address;
before((done) => {
server = createServer();
server.listen(() => {
const { port } = server.address();
address = `ws://localhost:${port}`;
done();
});
});
it('should connect and receive welcome message', (done) => {
const client = new WebSocket(address);
client.on('open', () => {
client.on('message', (message) => {
expect(message).to.equal('Welcome to the WebSocket server');
client.close();
done();
});
});
});
after(() => {
server.close();
});
});
You can expand these tests to handle message broadcasting, rate limiting, and edge cases (e.g., high traffic scenarios).
6. Practical Considerations for Scalable WebSocket Architecture
- Load Balancing: If you run multiple server instances, ensure session stickiness or handle session data in a shared datastore to keep communication consistent.
- Security: Consider HTTPS or WSS if sensitive data is transmitted. Use secure tokens or authentication/authorization layers.
- Monitoring and Metrics: Tools like PM2, New Relic, or Grafana can help track CPU/memory usage, active connections, and more.
- Error Handling and Logging: Implement robust error handling and structured logs to keep track of anomalies, disconnections, or broadcast failures.
You can ask Cloving to refine your logging strategy or incorporate popular logging libraries (e.g., winston, pino) with a simple prompt:
Add structured logging with pino to our WebSocket server.
7. Committing AI-Generated Code
After reviewing your changes, let Cloving help you create informative commit messages:
cloving commit
Cloving reviews your staged changes and suggests a descriptive commit message. Feel free to adjust it before committing:
Implement WebSocket server with basic broadcast functionality and rate limiting
This ensures your commit history is both clear and contextual.
8. Next Steps and Best Practices
- Continuous Refinement: Use Cloving’s interactive chat regularly to implement new features or optimizations.
- Documentation: Ask Cloving to generate Markdown docs or a README that describes how to set up and run your WebSocket server.
- Integration with Other Tools: Combine Cloving with CI/CD platforms (GitHub Actions, Jenkins, GitLab) to automate code generation, testing, and deployments.
- Stay Current: Keep your Node.js, dependencies, and Cloving updated. Emerging libraries and frameworks often reshape best practices for real-time architectures.
9. Conclusion
Using Cloving CLI, you can build scalable WebSocket servers with ease and confidence. From automatically generating server scripts and tests to revising code interactively, Cloving boosts your productivity and code quality—transforming how you approach Node.js development. The synergy of AI assistance with your expertise ensures an efficient, reliable real-time application stack.
Whether you’re implementing a chat app, a real-time dashboard, or any high-volume streaming service, Cloving streamlines the process, freeing you to focus on domain-specific challenges and user experience. Remember that Cloving is a collaborative tool. Your skills and design decisions remain paramount to shaping the final product and ensuring a robust, secure application.
Happy coding, and welcome to the AI-assisted future of building real-time apps with WebSockets and Node.js!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.