Creating Python Decorators with the Help of GPT
Updated on January 07, 2025

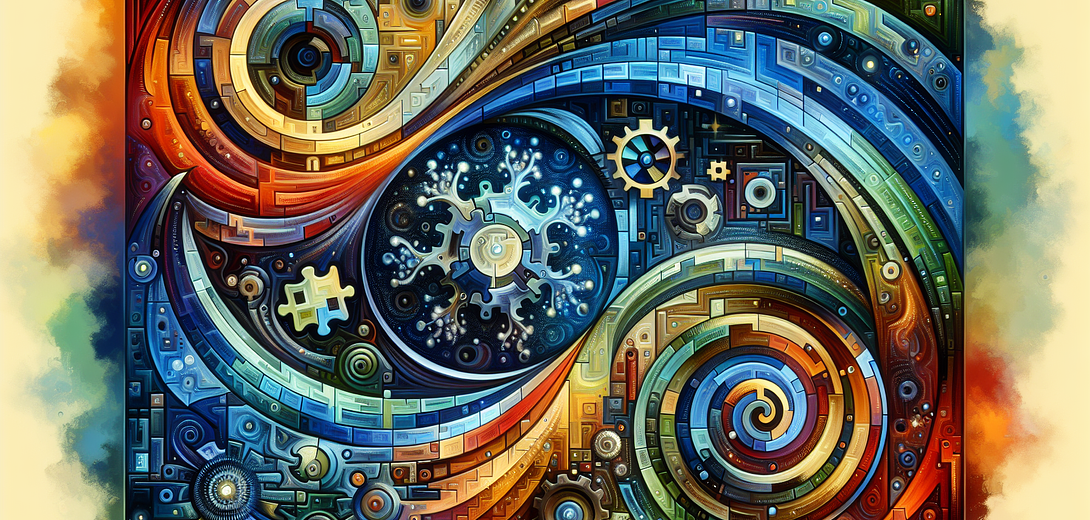
Python decorators are a powerful feature that allows programmers to extend and modify the functionality of functions and methods without altering their underlying code. While creating decorators can sometimes be challenging, the Cloving CLI tool, with its AI-powered assistance, can simplify the process significantly. In this blog post, we’ll explore how to leverage Cloving to create Python decorators more efficiently using GPT models.
Understanding Decorators
Before diving into the practical aspect of using Cloving, it’s essential to understand what decorators are in Python. Decorators are a way to modify the behavior of a function or method. They take another function as an argument and extend its behavior, often wrapping it with another function. Here’s a simple example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
# Output:
# Something is happening before the function is called.
# Hello!
# Something is happening after the function is called.
In the example above, my_decorator
is a decorator applied to say_hello
.
Setting Up Cloving Environment
To start using Cloving for creating Python decorators, we first need to set it up in our environment.
Installation
First, ensure Cloving is installed globally on your system:
npm install -g cloving@latest
Configuring Cloving
Configure Cloving to use your preferred AI model:
cloving config
Follow the interactive prompts to set up your AI model, API key, and preferences.
Initializing Your Project
Initialize Cloving in your project directory to provide it with context:
cloving init
This analyzes your project and creates a cloving.json
file that helps Cloving understand your project better.
Using Cloving to Create Decorators
With Cloving set up, we can now start using it to create Python decorators more efficiently.
Generating Decorators
Let’s say you want to create a logging decorator that logs the execution of a function. You can use Cloving to help you with this task:
cloving generate code --prompt "Create a Python decorator that logs function execution" --files utils/decorators.py
After running this command, Cloving analyzes the context and generates the following code snippet:
def logging_decorator(func):
def wrapper(*args, **kwargs):
print(f"Executing '{func.__name__}' with arguments {args} and keyword arguments {kwargs}")
result = func(*args, **kwargs)
print(f"'{func.__name__}' returned {result}")
return result
return wrapper
@logging_decorator
def add(a, b):
return a + b
add(2, 3)
# Output:
# Executing 'add' with arguments (2, 3) and keyword arguments {}
# 'add' returned 5
This code demonstrates a decorator that logs every call to the add
function, showing the function’s name, arguments, and return value.
Revising Generated Decorators
Cloving allows you to refine and revise generated code. For example, if you want to add a timestamp to the logging messages, you can do so directly from an interactive chat:
cloving chat -f utils/decorators.py
cloving> Revise the logging decorator to include a timestamp in the log messages
Cloving will update the decorator code with the desired changes:
import time
def logging_decorator(func):
def wrapper(*args, **kwargs):
timestamp = time.strftime("%Y-%m-%d %H:%M:%S", time.gmtime())
print(f"[{timestamp}] Executing '{func.__name__}' with arguments {args} and keyword arguments {kwargs}")
result = func(*args, **kwargs)
print(f"[{timestamp}] '{func.__name__}' returned {result}")
return result
return wrapper
@logging_decorator
def add(a, b):
return a + b
add(2, 3)
# Output:
# [YYYY-MM-DD HH:MM:SS] Executing 'add' with arguments (2, 3) and keyword arguments {}
# [YYYY-MM-DD HH:MM:SS] 'add' returned 5
Generating Unit Tests for Decorators
It’s crucial to test decorators to ensure they perform as expected. Use Cloving to generate unit tests for the decorator you’ve just created:
cloving generate unit-tests -f utils/decorators.py
This command will generate meaningful unit tests for your decorators, ensuring reliability and code quality.
Conclusion
Creating Python decorators can enhance your code’s functionality without altering the core logic. With Cloving, you can efficiently generate, revise, and test decorators, leveraging the power of AI to streamline your development process.
By mastering the use of Cloving CLI and its capabilities, you can increase your productivity and code quality, leveraging AI as your powerful coding assistant. Whether you are generating code snippets, reviewing code, or writing commit messages, Cloving is a tool worth incorporating into your Python development workflow.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.