Creating ORM Models in Django with the Help of GPT
Updated on March 07, 2025

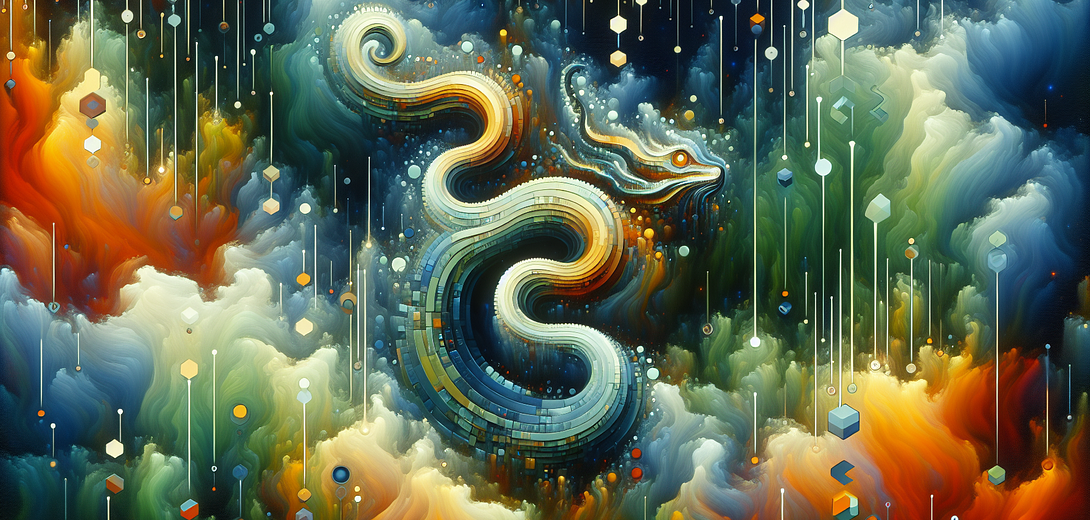
Streamlining Django ORM Model Creation with Cloving CLI
Django is known for its powerful Object-Relational Mapping (ORM), allowing developers to interact with databases using Python objects. Even though Django’s ORM is intuitive, creating and managing models for complex applications can still be time-consuming. Cloving CLI, an AI-driven command-line tool, helps automate and refine these tasks using GPT-based models. In this guide, we’ll show you how to integrate Cloving into your Django projects to quickly and reliably generate ORM models.
1. Introduction to Cloving CLI
Cloving CLI acts as an AI-powered pair programmer, assisting you in:
- Generating new code (e.g., Django models)
- Reviewing existing code for best practices
- Refining code via interactive chat
- Automating tasks like commit message generation
By leveraging GPT-based intelligence, Cloving can parse your project’s structure and produce relevant models or updates in line with your code style and architectural needs.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
After installing, configure Cloving by providing your API key and AI model (e.g., GPT-3.5, GPT-4):
cloving config
Follow the prompts to finalize your environment. This ensures Cloving can connect to your AI backend and tailor code generation to your preferences.
3. Initializing Cloving in Your Django Project
Navigate to your project’s root directory and run:
cloving init
Cloving analyzes your project and creates a cloving.json
file with metadata (like your Django version, installed apps, etc.). This context helps the AI produce more accurate model definitions.
4. Creating Django ORM Models
4.1 Example: Generating a Basic ORM Model
Assume you’re building a library management system and need a Book
model. Let Cloving produce it for you:
cloving generate code --prompt "Generate a Django ORM model for a Book with fields title, author, published_date, and ISBN" --files library/models.py
Sample Output:
# library/models.py
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=255)
author = models.CharField(max_length=255)
published_date = models.DateField()
isbn = models.CharField(max_length=13)
def __str__(self):
return self.title
Notes:
- Cloving recognizes basic Django field types (e.g.,
CharField
,DateField
) and sets a default max length forCharField
. - For custom logic or constraints (e.g., unique
isbn
), mention them in your prompt.
4.2 Adding or Refining Model Logic
You can refine the model further with interactive chat:
Revise the Book model to include a method that calculates the book's age
Cloving might produce:
from datetime import date
def get_age(self):
return date.today().year - self.published_date.year
Then you can merge or tweak as needed.
5. Generating Multiple Models
For larger projects with multiple models (e.g., Author
, Publisher
, Category
), Cloving can handle them in a single command:
cloving generate code --prompt "Generate Author and Publisher models for a library app" --files library/models.py
Cloving may append these new models:
class Author(models.Model):
name = models.CharField(max_length=255)
date_of_birth = models.DateField()
class Publisher(models.Model):
name = models.CharField(max_length=255)
city = models.CharField(max_length=100)
Adjust as needed for relationships (e.g., one-to-many or many-to-many fields).
6. Reviewing and Testing Models
6.1 Code Validation
After generation, verify your models using Django’s built-in commands:
python manage.py makemigrations
python manage.py migrate
If Cloving has introduced any errors or missing fields, correct them or request an interactive code fix:
cloving> The city field in Publisher should allow null values, please fix that
6.2 Unit Tests for Models
Although not strictly required for models, test coverage ensures logic (such as custom methods or signals) remains robust. You can direct Cloving to produce initial test stubs:
cloving generate unit-tests -f library/models.py
Cloving might output a test file like:
# tests/test_models.py
from django.test import TestCase
from library.models import Book
class BookModelTest(TestCase):
def test_str_representation(self):
book = Book.objects.create(
title="Sample Book",
author="John Doe",
published_date="2020-01-01",
isbn="1234567890123"
)
self.assertEqual(str(book), "Sample Book")
Extend these tests to cover custom methods or constraints.
7. Using Cloving Chat for Advanced Scenarios
Cloving chat fosters an interactive environment for complex or iterative tasks:
cloving chat -f library/models.py
Within this session, you can:
- Ask for advanced relationships (e.g.,
ForeignKey
,ManyToManyField
, or intermediate models). - Request help adding signals or custom managers.
- Iterate on performance improvements or indexing strategies.
8. Streamlining Commits
Good commit messages keep your project’s history clear. Use Cloving to automate them:
cloving commit
Cloving analyzes staged changes (like new or modified models) and suggests a succinct message, for example:
Add Book model with basic fields and age calculation method
9. Best Practices for Using Cloving with Django
- Initialize Each Project
Always runcloving init
so the AI can parse relevant settings (installed apps, project structure). - Detailed Prompts
Mention field types, constraints, relationships, or domain-specific logic in your prompt. - Refine Interactively
Large or complex models might need iterative improvements. Rely oncloving chat
for expansions or bug fixes. - Apply Django Conventions
Always confirm that the AI-generated fields follow best Django naming, constraint usage (e.g.unique=True
,null=True
), and relationships. - Test Thoroughly
Expand on Cloving’s auto-generated test stubs for coverage of custom logic, signals, or migrations.
10. Example Workflow
Below is a typical approach to building Django models with Cloving:
- Initialize:
cloving init
at your project’s root. - Generate:
cloving generate code --prompt "Create a Book model..." -f library/models.py
. - Refine: Possibly run
cloving chat -f library/models.py
to add constraints or relationships. - Test:
cloving generate unit-tests -f library/models.py
for basic coverage. - Commit: Summarize the changes with
cloving commit
. - Migrations: As usual, run
manage.py makemigrations
andmanage.py migrate
to finalize database schema updates.
11. Conclusion
By harnessing Cloving CLI in your Django projects, you can drastically simplify the process of creating and refining ORM models. Whether you’re building a small personal project or a large-scale application, Cloving’s AI-driven approach helps you keep models clean, well-structured, and tailored to your domain logic.
Remember: While Cloving automates repetitive tasks, it’s still crucial to review AI-generated code for domain-specific correctness, best practices, and style consistency. Embrace Cloving’s AI capabilities and let it speed up your Django development process, freeing you to focus on core features and innovation.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.