Creating Efficient Backend Services with GPT in Python Flask
Updated on April 17, 2025

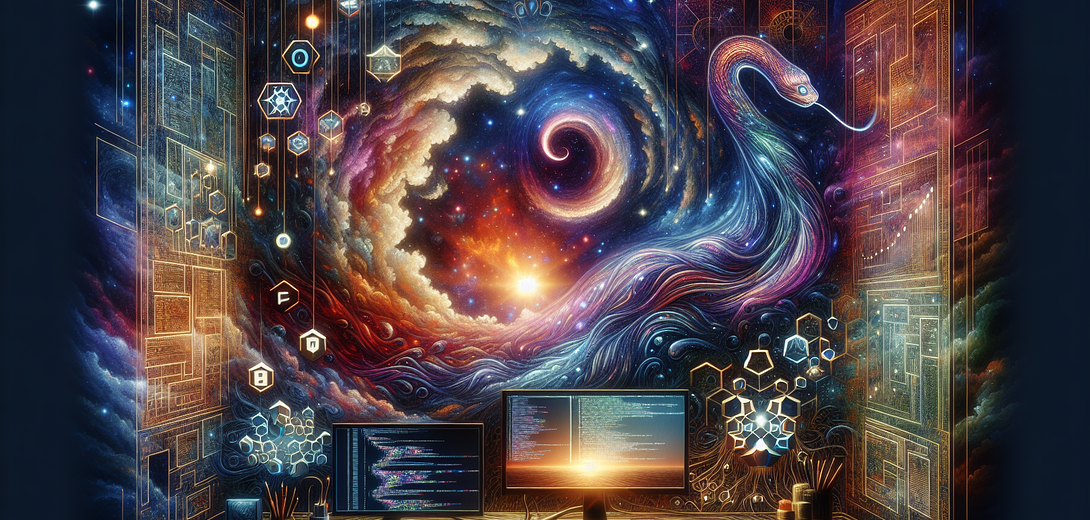
The demand for efficient and scalable backend services is ever-increasing. Integrating AI into backend processes can significantly streamline operations and improve code quality. This post will guide you through creating efficient backend services using the Cloving CLI tool and leveraging GPT models in a Python Flask application.
Getting Started with Cloving CLI
To harness the power of Cloving in your Flask projects, you need to set it up in your environment. Follow these steps to get started:
1. Installation and Configuration
First, install Cloving globally via npm:
npm install -g cloving@latest
Next, configure Cloving by setting up your AI model and preferences:
cloving config
This will prompt you to input your API key and select the AI model you’d like to use with Cloving.
2. Initialize Your Flask Project
Navigate to your Flask project directory and initialize Cloving:
cloving init
This will create a cloving.json
file that stores metadata about your project, helping Cloving understand its context for better AI-powered code generation.
Using Cloving for Backend Development
Cloving offers various commands and features that can further enhance your Python Flask backend development process. Let’s explore how.
3. Generating Flask Endpoints
Cloving can help generate Flask endpoints to handle various HTTP methods. For example, to create a new endpoint for managing user data, use the generate
command:
cloving generate code --prompt "Create a Flask endpoint for managing user data"
This command will generate a Python code snippet for a Flask endpoint:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/api/users', methods=['GET', 'POST'])
def manage_users():
if request.method == 'GET':
# Retrieve user data
return jsonify({"users": []})
elif request.method == 'POST':
# Add new user data
user = request.json
return jsonify({"message": "User added", "user": user})
if __name__ == '__main__':
app.run(debug=True)
4. Enhancing Endpoints with AI-Power
After generating the initial endpoint, you might want to enhance it with features like validation, error handling, or integrating GPT for processing user requests. Engage Cloving’s interactive chat for revisions:
cloving chat -f app.py
In the interactive session, you can provide additional requests:
cloving> Enhance the user endpoint with input validation and GPT-based summarization of user data
5. Creating Unit Tests for Flask Endpoints
Ensuring code quality through unit tests is crucial. Use Cloving to generate tests for your Flask endpoints:
cloving generate unit-tests -f app.py
This may result in tests like:
import unittest
from app import app
class FlaskAppTests(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_get_users(self):
response = self.app.get('/api/users')
self.assertEqual(response.status_code, 200)
def test_post_user(self):
response = self.app.post('/api/users', json={"name": "Alice"})
self.assertEqual(response.status_code, 200)
self.assertIn("Alice", response.get_json()["user"]["name"])
if __name__ == '__main__':
unittest.main()
6. Optimizing Flask Services with AI
Leverage AI models for tasks like data summarization or natural language processing. For instance, integrating GPT to summarize user inputs in your services can be achieved through Cloving:
cloving generate code --prompt "Integrate GPT for summarization in Flask"
7. Commit Changes with AI Assistance
Capture these enhancements effectively in your commit messages using Cloving:
cloving commit
This tool generates detailed commit messages, saving you time and ensuring all changes are well-documented.
Conclusion
With Cloving CLI, augment your backend services in Python Flask with AI capabilities, enhancing productivity and code quality. By leveraging its powerful commands, from generating code to creating unit tests and insightful commit messages, Cloving assists you in developing efficient and robust backend services.
Embrace Cloving’s AI-driven features to innovate and elevate your Python Flask projects to new heights.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.