Creating Efficient Backend Services with Flask and GPT
Updated on January 03, 2025

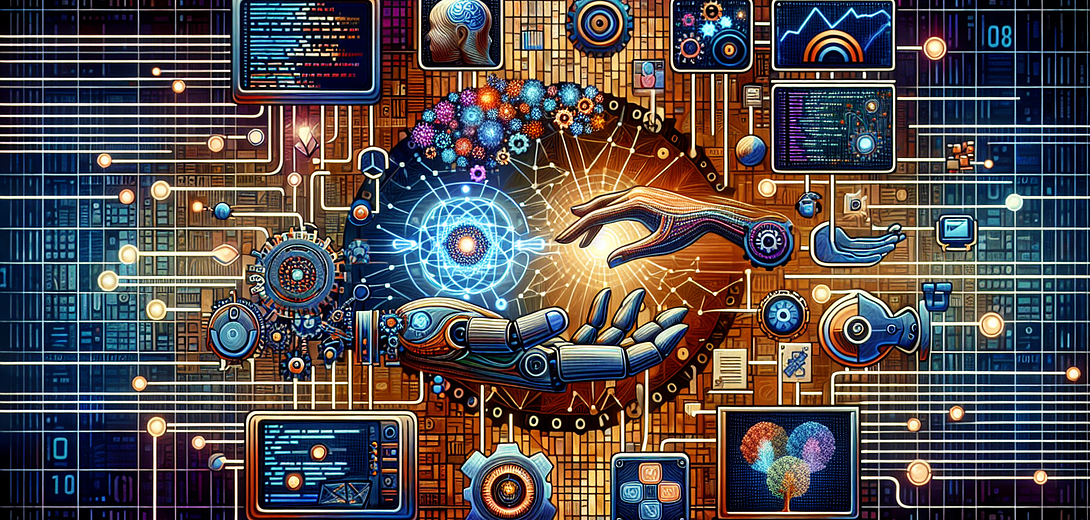
In the modern age of software development, the line between AI-assisted coding and human ingenuity is becoming increasingly blurred. The Cloving CLI tool is at the forefront of this revolution, enabling developers to harness the power of AI in creating efficient backend services using frameworks like Flask. In this blog post, we will guide you through the process of using Cloving to enhance your Flask development workflow, making you a more productive and effective programmer.
Understanding Cloving CLI
Cloving is an AI-powered CLI tool that complements your programming skills. It assists in generating code snippets, unit tests, and even aiding your development process with informed suggestions. The focus here will be on crafting backend services with Flask, leveraging Cloving to improve efficiency and code quality.
1. Setting Up Cloving
Before diving into Flask development, let’s ensure you have Cloving set up properly:
Installation:
First, install Cloving globally:
npm install -g cloving@latest
Configuration:
Configure Cloving with your AI model and API key:
cloving config
This step involves following prompts to select your AI model and input your API credentials.
2. Initialize Your Flask Project
To utilize Cloving’s features effectively, initialize it within your Flask project directory:
cloving init
The initialization allows Cloving to generate a context based on your project’s structure, creating a cloving.json
file with pertinent information about your application.
3. Generating Flask Backend Code
With Cloving, generating boilerplate code for your Flask application is a breeze.
Example:
Let’s assume you need to create a basic API endpoint for managing user data. Instead of writing everything from scratch, use:
cloving generate code --prompt "Create a Flask API endpoint for handling user data" --files app/routes.py
Cloving will produce a relevant code snippet:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/users', methods=['GET', 'POST'])
def manage_users():
if request.method == 'POST':
data = request.json
# Assume a function add_user_to_db(data) exists
add_user_to_db(data)
return jsonify({"status": "User added"}), 201
else:
# Assume a function get_all_users() exists
users = get_all_users()
return jsonify(users)
if __name__ == '__main__':
app.run(debug=True)
This autogenerated code sets up a simple API to add and retrieve users, naturally aligning with Flask’s design principles.
4. Interactive Code Versioning
Once Cloving generates the code, you can choose to refine or save it:
- Revise functionality
- Explain the logic
- Save or copy the snippet
Use the interactive option for additional revisions:
cloving generate code -i --prompt "Enhance the user endpoint to paginate responses" --files app/routes.py
The interactive mode will prompt you to edit existing code or provide more specific instructions.
5. Unit Test Generation
Ensuring code reliability is essential. Cloving can help by generating unit tests:
cloving generate unit-tests -f app/routes.py
This will create unit tests that match the functionalities of your Flask app, promoting robustness.
import pytest
from app import app
@pytest.fixture
def client():
with app.test_client() as client:
yield client
def test_get_users(client):
rv = client.get('/users')
assert rv.status_code == 200
def test_post_user(client):
rv = client.post('/users', json={"name": "John Doe"})
assert rv.status_code == 201
These tests ensure your endpoints behave as expected.
6. Using Cloving Chat for Exploration
For complex tasks or brainstorming, engage Cloving in chat:
cloving chat -f app/routes.py
This interactive session allows you to request AI-generated explanations, further code snippets, or even engage in a code review. Whether you need guidance on a Flask design pattern or advice on improving API performance, Cloving acts as a knowledgeable assistant.
7. AI-Powered Code Reviews
Optimize your codebase with an automated review process:
cloving generate review
Cloving will evaluate your project files and provide insights on optimization, potential bugs, or stylistic improvements in an easy-to-digest format.
Conclusion
Embracing the Cloving CLI for your Flask backend services demonstrates the strength of integrating AI into your development toolkit. The tool not only enhances code generation and unit testing but also fosters an environment for collaborative AI-driven problem solving.
In leveraging Cloving, you’re not replacing your coding expertise but rather augmenting it, boosting productivity and fostering innovation. Start using Cloving today and witness a paradigm shift in your Flask development journey.
Stay tuned for more tutorials as we unravel the potential of Cloving and AI in software development!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.