Creating DynamoDB Schemas with AI-Driven Code Generation by GPT
Updated on January 20, 2025

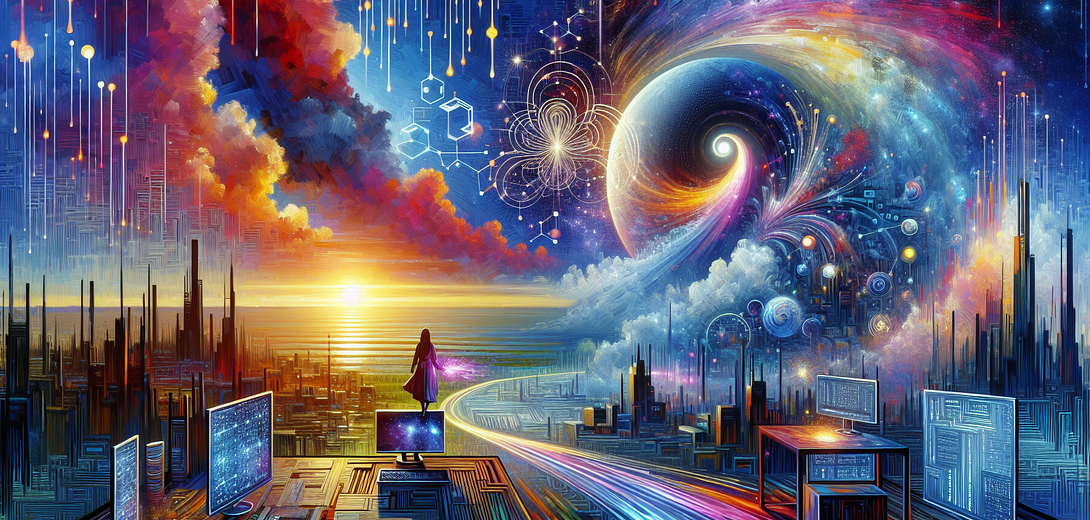
As developers, creating database schemas can often be both challenging and monotonous. With the emergence of AI-powered tools like the Cloving CLI, however, we can streamline this process and empower ourselves to generate efficient database schemas with ease. In this blog post, we’ll guide you through the process of creating DynamoDB schemas using Cloving’s AI-driven code generation.
Leveraging Cloving CLI for DynamoDB Schema Generation
Cloving’s CLI tool enriches your development environment with AI capabilities, offering intuitive code generation right from your terminal. In this post, we will explore how to design DynamoDB schemas using Cloving’s code generation features, enhancing productivity and ensuring high-quality code output.
1. Cloving Setup
Begin by setting up Cloving in your development environment.
Installation:
Install Cloving globally through npm:
npm install -g cloving@latest
Configuration:
To get started, configure Cloving by specifying the AI model and API key:
cloving config
This sets up Cloving with your preferred AI service provider.
Project Initialization:
Navigate to your project directory and initialize Cloving:
cloving init
Initializing Cloving helps it understand your project context and creates a cloving.json
file for metadata storage.
2. Using Cloving to Generate DynamoDB Schemas
Let’s move on to code generation, specifically focusing on DynamoDB schema design.
Example:
Imagine you are setting up a new application that requires a DynamoDB table for user data. Use Cloving’s code generation to create a schema:
cloving generate code --prompt "Design a DynamoDB schema for storing user profiles including userId, name, email, and dateOfBirth" --files schemas/userProfileSchema.ts
The AI will analyze the context and generate a schema like this:
// schemas/userProfileSchema.ts
import { DynamoDB } from 'aws-sdk';
const dynamodb = new DynamoDB();
const tableParams = {
TableName: 'UserProfiles',
KeySchema: [
{ AttributeName: 'userId', KeyType: 'HASH' } // Partition key
],
AttributeDefinitions: [
{ AttributeName: 'userId', AttributeType: 'S' },
{ AttributeName: 'name', AttributeType: 'S' },
{ AttributeName: 'email', AttributeType: 'S' },
{ AttributeName: 'dateOfBirth', AttributeType: 'S' }
],
ProvisionedThroughput: {
ReadCapacityUnits: 5,
WriteCapacityUnits: 5
}
};
dynamodb.createTable(tableParams, (err, data) => {
if (err) {
console.error("Error creating table:", err);
} else {
console.log("Table created:", data);
}
});
3. Reviewing and Revising Schema
After code generation, you may want to revise or review the schema. Utilize Cloving’s interactive capabilities to achieve this.
To make adjustments to the schema, you can simply ask Cloving to make specific changes. For example, if you need to add a new attribute for user addresses, you can start an interactive session:
$ cloving chat -f schemas/userProfileSchema.ts
In this chat interface, request:
Please add an address attribute to the user profile schema.
The AI will promptly adjust your schema and present revisions for your approval.
4. Generate Unit Tests for Schema
For ensuring schema integrity, you can generate unit tests with Cloving:
cloving generate unit-tests -f schemas/userProfileSchema.ts
This command auto-generates unit tests that validate the schema definition, vital for maintaining code quality.
// schemas/userProfileSchema.test.ts
import { expect } from 'chai';
import { DynamoDB } from 'aws-sdk';
import { tableParams } from './userProfileSchema';
describe('UserProfile Schema', () => {
it('should define a userId as the partition key', () => {
const keySchema = tableParams.KeySchema.find(key => key.AttributeName === 'userId');
expect(keySchema).to.not.be.undefined;
expect(keySchema.KeyType).to.equal('HASH');
});
it('should contain all defined attributes', () => {
const attributes = tableParams.AttributeDefinitions.map(attr => attr.AttributeName);
expect(attributes).to.include.members(['userId', 'name', 'email', 'dateOfBirth']);
});
});
5. Making Commits with AI Assistance
Finally, for better project management, Cloving can assist with meaningful commit messages by analyzing changes:
cloving commit
This command suggests a commit message, allowing you to make informative, context-driven commits seamlessly.
Conclusion
Integrating AI into your workflow through Cloving CLI simplifies the process of creating DynamoDB schemas, letting you focus on the logical structure of your data while AI handles much of the grunt work. From generating accurate schemas to assisting with code revisions and testing, Cloving enhances your productivity and code reliability.
Embrace the power of Cloving and harness AI-driven code generation to elevate your development experience in creating robust DynamoDB schemas.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.